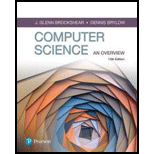
Explanation of Solution
Modified interface for Queue and its code:
The modified interface for queue and its corresponding queue of integer’s implementation in java and C# is shown below:
//Interface for QueueType
interface QueueType
{
//Function declaration to add a value to queue
public void insertQueue(int item);
//Function declaration to remove a value from queue
public int removeQueue();
//Function declaration for check if queue is empty
public boolean isEmpty();
//Function declaration for check if queue is Full
public boolean isFull();
}
//Class for QueueOfInteger
class QueueOfIntegers implements QueueType
{
//Initializes the size of queue
private int size = 20;
//Create an array for QueueEntries
private int[] QueueEntries = new int[size];
/* Declare the variable for front of queue, rear of queue and length of queue*/
private int frontQueue, rearQueue, queueLength;
//Function definition for insert Queue
public void insertQueue(int NewEntry)
{
//If the rearQueue is equal to '-1', then
if (rearQueue == -1)
{
//Assign the frontQueue to "0"
frontQueue = 0;
//Assign the rearQueue to "0"
rearQueue = 0;
/* Assign the Array of QueueEntries to NewEntry */
QueueEntries[rearQueue] = NewEntry;
}
/* If the rearQueue+1 is greater than or equal to "size" */
else if (rearQueue + 1 >= size)
System.out.println("Queue Overflow Exception");
//If the rearQueue+1 is less than "size"
else if ( rearQueue + 1 < size)
/* Assign the Array of QueueEntries to NewEntry */
QueueEntries[++rearQueue] = NewEntry;
//Increment the queue length
queueLength++ ;
}
//Function definition for remove a value from queue
public int removeQueue()
{
//If the queue is not empty, then
if(!isEmpty())
//Decrement the length of queue
queueLength--;
/* Assign the front of queue entries to element */
int element = QueueEntries[front];
//If the queue front is equal to rear
if(frontQueue == rearQueue)
{
/* Assign the value to front and rear of queue */
frontQueue = -1;
rear...

Want to see the full answer?
Check out a sample textbook solution
Chapter 8 Solutions
Computer Science: An Overview (13th Edition) (What's New in Computer Science)
- C# Display the stack with the given information: int topNum; NumberStack stack2 = new NumberStack(5); stack2.Push(5);stack2.Push(7);stack2.Push(12);stack2.Push(3); public void PrintStack() { //Loop through each element of the stack and display it for (int i = size - 1 ; i >= 0; i--) { Console.WriteLine(stack[i]); } }arrow_forwardGiven a class Stack with the interface public void push(char n) // pushes n onto stack public char pop() // return the top of the stack, removing element from stack public boolean isEmpty() // return true if stack is empty Write a method public void addStars(Stack<Character> stack) which takes a Stack as input and adds two asterisk (‘*’) at the front and back of all the elements in the stack. The stack must be restored to its original order plus the asterisks. For example, the stack [bottom] a b c d [top] Would become [bottom] ** a b c d ** [top]arrow_forwardAdd the following operation to the Class StackClass: void reverseStack(StackClass<T> otherStack); This operation copies the elements of a stack in reverse order onto another stack. Consider the following statements: StackClass<T> stack1; StackClass<T> stack2; The statement stack1.reverseStack(stack2); copies the elements of stack1 onto stack2 in the reverse order. That is, the top element of stack1 is the bottom element of stack2, and so on. The old contents of stack2 are destroyed and stack1 is unchanged. Write the definition of the method to implement the operation reverseStack. Also write a program to test the method reverseStack. In StackClass.java, please finish method - public void reverseStack(StackClass<T> otherStack) and testing main program public class Problem53{public static void main(String[] args){StackClass<Integer> intStack = new StackClass<Integer>();StackClass<Integer> tempStack = new StackClass<Integer>(); //Please…arrow_forward
- 1. Assume you have a queue with operations: enqueue(), dequeue(), isEmpty(). How would you use the queue methods to simulate a stack, in particular, push() and pop() ? Hint: use two queues, one of which is the main one and one is temporary.arrow_forwardAdd the following operation to the Class StackClass: void reverseStack(StackClass<T> otherStack); This operation copies the elements of a stack in reverse order onto another stack. Consider the following statements: StackClass<T> stack1; StackClass<T> stack2; The statement stack1.reverseStack(stack2); copies the elements of stack1 onto stack2 in the reverse order. That is, the top element of stack1 is the bottom element of stack2, and so on. The old contents of stack2 are destroyed and stack1 is unchanged. Write the definition of the method to implement the operation reverseStack. Also write a program to test the method reverseStack. In StackClass.java, please finish method - public void reverseStack(StackClass<T> otherStack) and testing main program – Problem53.java. Note: java programming, please use given outline code public class Problem53{ publicstaticvoidmain(String[] args){ StackClass<Integer> intStack = newStackClass<Integer>();…arrow_forwardBook: Java Software Structures Author: John Lewis; Joe Chase Javascript 1. Create a generic type java interface StackADT<T>with the following methods: a.public void push(T element);//Push an element at the top of a stack b.public T pop();//Remove and return an element from the top of a stack c.public T peek();//return the top element without removing it d.public booleanisEmpty();//Return True if a stack is emptye.public int size();//Return the number of elements in a stack f.public String toString();//Print all the elements of a stack 2. Define ArrayStack<T>class which will implementStackADT<T>interface 3. Use your ArrayStack<T>class to compute a postfix expression, for instance, 3 4 * will yield12;3 4 6 + *will yield 30 etc. During computing a postfix expression: a.While you perform a push operation, if the stack is full, generate an exception b.While you perform a pop operation, if the stack is empty, generate an exception c.If two operands are not available…arrow_forward
- In Java please create a generic stack code and implement these methods peek() returns a reference to the item at the top of the stack without removing it from the stack contains(T t) is a boolean method that returns true if the stack contains t, the parameter, otherwise returns false. The stack contains t if at least one T on the stack is equal to t using the equals() method. Note that there is always an available equals() method in Java, because Object, the common parent of all classes, contains one. Implement this method without using the contains()method of the list.arrow_forwardA data structure known as a drop-out stack functions exactly like a stack, with the exception that if the stack size is n, the first element is lost when the n + 1 element is pushed. Use an array to implement a drop-out stack. (Hint: It would make sense to implement a circular array.)arrow_forwardX1019: Use a Stack to a Reverse order of a Queue Use these two interfaces to solve this problem. 1 public interface QueueADT { public void clear(); public boolean enqueue(E it); 3 public E dequeue (); public E frontValue(); public int numElements(); public boolean isEmpty(); 8 } 4 5 6. 7 9 10 interface StackADT { public void clear(); public boolean push(E it); 11 12 public E pop(); public E topValue(); public int numElements(); public boolean isEmpty(); 13 14 15 16 17 } Write a method that reverses the elements in a queue. For example, if the queue starts with "A","B"C" then after the routine runs, the queue should have "C","B", "A. This should work for all values in the queue. One easy way to do this is to use a stack and copy all elements from the queue and then copy the contents of the stack back to the queue. You should use a StackArray . If queue is null then do nothing. Make sure you use the interfaces from above. Your Answer: 1 void reverseQueue (QueueADT queue) 2{ 3 4} 5arrow_forward
- Project Overview: This project is for testing the use and understanding of stacks. In this assignment, you will be writing a program that reads in a stream of text and tests for mismatched delimiters. First, you will create a stack class that stores, in each node, a character (char), a line number (int) and a character count (int). This can either be based on a dynamic stack or a static stack from the book, modified according to these requirements. I suggest using a stack of structs that have the char, line number and character count as members, but you can do this separately if you wish.Second, your program should start reading in lines of text from the user. This reading in of lines of text (using getline) should only end when it receives the line “DONE”.While the text is being read, you will use this stack to test for matching “blocks”. That is, the text coming in will have the delimiters to bracket information into blocks, the braces {}, parentheses (), and brackets [ ]. A string…arrow_forwardExplain the difference between a static stack and a dynamic stack.arrow_forwardIn this problem you will need to delete the middle element left middle element of the provided stack and return the stack new Stack. import java.util.Stack;public class DeleteMiddleOfStack{public static Stack solution(Stack stack){// ↓↓↓↓ your code goes here ↓↓↓↓return new Stack<>();}}arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
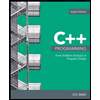