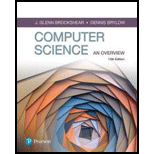
Explanation of Solution
The algorithm for printing a linked list in reverse order using a stack is shown below:
Procedure ReverseLinkedListPrint(List)
CurrentPtr ← Head pointer of list
While (CurrentPtr is not equal to NULL) do
Push the entry pointed to by CurrentPtr on to stack;
CurrentPtr ← Value of next pointer in entry pointed to by CurrentPtr.
While (Stack is not empty) do
Print the top value in the stack
Pop an entry from the stack.
Algorithm explanation:
- The given algorithm is used to print a linked list in reverse order using a stack.
- From the above algorithm, first define the procedure “ReverseLinkedListPrint” with argument “List”...
Explanation of Solution
Recursive function for printing the linked list in reverse order:
The recursive function for printing the linked list in reverse order is shown below:
Function ReverseLinkedListPrint(List)
If the head pointer of List is not NULL, then
Recursively call the function "ReverseLinkedListPrint" with the first entry of given List;
Print the first entry in List
Function Explanation:
- The given function is used to print the linked list in reverse order using recursive function.
- First define the function “ReverseLinkedListPrint”...

Want to see the full answer?
Check out a sample textbook solution
Chapter 8 Solutions
Computer Science: An Overview (13th Edition) (What's New in Computer Science)
- 4. Sort elements in an array-based stack in ascending order, i.e. make the smallest element to be the top element of the stack. E.g. given the stack elements (from bottom to top): 90, 70, 80, 10, sort the elements to make the stack elements become (from bottom to top): 90, 80, 70, 10. The only data structure you can use is array-based stack. In addition to the given stack, you can use only one extra stack to store some temporary data. Given a stack st, use one extra stack, tmpst, to store temporary data. Here are some hints of the idea. Pop out the top element of st to a variable tmp. If the stack tmpst is empty, push tmp onto tmpst; if tmpst is not empty, pop out its top element and push that element onto st until the top element of tmpst is smaller than tmp, then push tmp onto tmpst .. a. Write a program to implement the stack based sorting. b. Take the input (90, 70, 80, 10) as an example. c. Print all the push and pop operations in proper format. d. At the end, print the sorted…arrow_forward1. a function that takes in a list (L), and creates a copy of L. note: The function should return a pointer to the first element in the new L. [iteration and recursion]. 2. a function that takes in 2 sorted linked lists, and merges them into a single sorted list. note: This must be done in-place, and it must run in O(n+m).arrow_forwardQ. No. 2. Write a program to input and push 12, 7, 8, 9, 4, 6, 5, 8 and 2 more values any of your choice into a stack. Pop the values from the stack and compute factorial of each using recursive function and show values on the screen.arrow_forward
- QUESTION: NOTE: This assignment is needed to be done in OOP(c++/java), the assignment is a part of course named data structures and algorithm. A singly linked circular list is a linked list where the last node in the list points to the first node in the list. A circular list does not contain NULL pointers. A good example of an application where circular linked list should be used is a items in the shopping cart In online shopping cart, the system must maintain a list of items and must calculate total bill by adding amount of all the items in the cart, Implement the above scenario using Circular Link List. Do Following: First create a class Item having id, name, price and quantity provide appropriate methods and then Create Cart/List class which holds an items object to represent total items in cart and next pointer Implement the method to add items in the array, remove an item and display all items. Now in the main do the following Insert Items in list Display all items. Traverse…arrow_forwardWrite programs for the following operations on linked list in python.a. To search for an element in a single linked list.b. To count the occurrence of an element in a single linked list.c. To implement stack operations using single linked list.d. To implement queue operations using single linked list.e. To check whether two single lists are same or not.f. To count the occurrences of all the elements in a single linked list.g. To find the smallest element in a double linked listarrow_forwardAs recursion is implemented using a stack, an object of class Stack must be declared and initialized in the program to hold the class objects of every recursive call? a)true b) falsearrow_forward
- C++ Programming RECURSION AND ITS USE IN PROGRAMMING. Write a program for this task using recursion. Development of a recursive triad. Create a recursive call tree for a recursive algorithm. Task : Given an array of size N. Create a function to determine the element that is farthest from the arithmetic mean value of the array elements (that is, the maximum difference by the module).arrow_forwardDevelop an application using java language that store characters A, B and C in a stack array and then displays both the size and the last-in element of the stack. The application should then remove the last element of the stack and then display again both the size and the last-in element of the stack. Appropriate stack methods should be used to add, delete and display characters.arrow_forwardShow a complete run of Quicksort on the following array X :X: 1 2 3 4 5 6 7 8 9 1017 5 -3 40 46 50 16 0 22 4 show all recursive calls using the stack below. Show the results of each call to below Split on a newline in the table to the left.arrow_forward
- Complete using Standard C programming. Implement a singly linked list that performs the following: Displays the maximum value in the linked list using recursion. Displays the linked list in reverse order using recursion. Merge two single linked lists and display.arrow_forwardPractice recursion on lists Practice multiple base conditions Combine recursion call with and,or not operators Search for an element in a list using recursion Instructions In this lab, we will write a recursive function for searching a list of integers/strings. Write a recursive function to determine if a list contains an element. Name the function recursive_search(aList, value), where aList is a list and value if primitive type is the object we want to search for. Return a boolean, specifically True if and only if value is an element of aList else return False from the function Examples: recursive_search([1,2,3], 2) == True recursive_search([1,2,3], 4) == False recursive_search([ ], 4) == False recursive_search([ [ 1 ], 2 ], 1) == False # -----> (because the list contains [ 1 ] and 2, not 1) Hint: Think about what the base case is? When is it obvious that the element is not in the list? If we are not at the base case, how can you use the information about the first element of the…arrow_forwardWhat happens if the base condition is not defined in recursion ? a. Stack underflow b. Stack Overflow c. None of these d. Both a and barrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
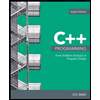