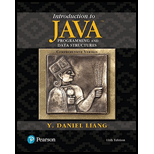
(Convert Celsius to Fahrenheit) Write a
fahrenheit = (9 / 5) * celsius + 32
Hint: In Java, 9 / 5 is 1, but 9.0 / 5 is 1.8.
Here is a sample run:

Convert Celsius to Fahrenheit
Program Plan:
- Define the class
- Define the main method using public static main.
- Declare the required variables.
- Prompt the user to enter Celsius value.
- Read the input
- Convert it into Fahrenheit value.
- Display the result on the screen.
- Define the main method using public static main.
The below program is used to convert Celsius to Fahrenheit.
Explanation of Solution
Program:
//class definition
public class Cel_Far
{
//Main method
public static void main(String[] args)
{
//declare scanner variable
Scanner in = new Scanner(System.in);
// Prompt the user to enter Celsius
System.out.print("Enter a temperature in Celsius: ");
//read the input
double celsius = in.nextDouble();
// Convert Celsius to Fahrenheit
double fh = (9.0 / 5) * celsius + 32;
// Display the result on the screen
System.out.println(celsius + " Celsius is " +
fh + " Fahrenheit");
}
}
Enter a temperature in Celsius: 43.5
43.5 Celsius is 110.3 Fahrenheit
Want to see more full solutions like this?
Chapter 2 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Additional Engineering Textbook Solutions
Web Development and Design Foundations with HTML5 (9th Edition) (What's New in Computer Science)
Java: An Introduction to Problem Solving and Programming (7th Edition)
Java How to Program, Early Objects (11th Edition) (Deitel: How to Program)
Computer Systems: A Programmer's Perspective (3rd Edition)
Web Development and Design Foundations with HTML5 (8th Edition)
Problem Solving with C++ (9th Edition)
- (Find the two highest scores)Write a program that prompts the user to enter the number of students and each student’s name and score, and displays the name and score of the student with the highest score and the student with the second-highest score.Sample RunEnter the number of students: 5Enter a student name: SmithEnter a student score: 60Enter a student name: JonesEnter a student score: 96Enter a student name: PetersonEnter a student score: 85Enter a student name: GreenlawEnter a student score: 98Enter a student name: ZhangEnter a student score: 95Top two students:Greenlaw's score is 98.0Jones's score is 96.0arrow_forward(In Java) In the game Clacker, the numbers 1 through 12 are initially displayed. The player throws two dice and may cover the number representing the total or the two numbers on the dice. For example, for a throw of 3 and 5, the player may cover the 3 and the 5 or just the 8. Play continues until all the numbers are covered. The goal is to cover all numbers in the fewest rolls. Create a Clacker application that displays 12 buttons numbered 1 through 12. Each of these buttons can be clicked to ‘cover’ it. A covered button displays nothing. Another button labelled Roll can be clicked to roll the dice. Include labels to display the appropriate die images for each roll. Another label displays the number of rolls taken. A New Game button can be clicked to clear the labels and uncover the buttons for a new game.arrow_forward(Game: scissor, rock, paper) Write a program that plays the popular scissor–rock–paper game. Rules: A scissor can cut a paper, a rock can knock a scissor, a paper can wrap a rock. The program should randomly generates a number 0, 1, or 2 representing scissor, rock, and paper. The program should prompt the user to enter a number 0, 1, or 2 and displays a message indicating whether the user or the computer WINs, LOSEs, or DRAWs.arrow_forward
- ) The Bahraini Charity Club is conducting a fundraiser by selling chilli dinners to go.The price is BD2.1 for an adult meal and BD1.2 for a child’s meal.Write a Java program that accepts the number of each type of meal ordered and displaythe total money collected for adult meals, children’s meals and all meals.arrow_forward(Geometry: distance of two points) Write a program that prompts the user to enter two points (x1, y1) and (x2, y2) and displays their distance between them. The formula for computing the distance is: Square root of ((x2 - x1) squared + (y2 - y1) squared) Note that you can use pow(a, 0.5) to compute square root of a. Sample Run Enter x1 and y1: 1.5 -3.4 Enter x2 and y2: 4 5 The distance between the two points is 8.764131445842194arrow_forwardCan you help me with the codes required for this assignment? the programming langauge is Java. (Sort three integers) Write a program that prompts the user to enter three integers and display the integers in non-decreasing order. Sample output:Enter three integers: 10 2 7 2 7 10arrow_forward
- (Written in Java or Python) Write a program that allows a user to select 3 points on a graphics panel. Then, draw a triangle using these 3 points. Allow the user to specify a number of random points to be generated inside of the triangle. For each random point that is within the boundaries of the triangle draw a small dot at each of the points that are halfway between the randomly generated point and each vertex of the triangle. Do not draw the randomly generated point. Allow the user to repeat the process without removing the old dots. Allow the user to clear the screen.arrow_forward(Find the number of years) Write a program that prompts the user to enter the minutes (e.g., 1 billion), and displays the number of years and days for the minutes. For simplicity, assume a year has 365 days. Sample Run Enter the number of minutes: 1000000000 1000000000 minutes is approximately 1902 years and 214 daysarrow_forward(Geometry: point in a circle?) Write a program that prompts the user to enter apoint (x, y) and checks whether the point is within the circle centered at (0, 0) withradius 10. For example, (4, 5) is inside the circle and (9, 9) is outside the circle, asshown in Figure 3.7a.(Hint: A point is in the circle if its distance to (0, 0) is less than or equal to 10.The formula for computing the distance is 2(x2 - x1)2 + (y2 - y1)2. Test yourprogram to cover all cases.) Two sample runs are shown below: Enter a point with two coordinates: 4 5Point (4.0, 5.0) is in the circleEnter a point with two coordinates: 9 9Point (9.0, 9.0) is not in the circlearrow_forward
- (Check password) Some websites impose certain rules for passwords. Write a method that checks whether a string is a valid password. Suppose the password rules are as follows: A password must have at least eight characters. A password consists of only letters and digits. A password must contain at least two digits. Write a program that prompts the user to enter a password and displays Valid Password if the rules are followed or Invalid Password otherwise.arrow_forwardTake screenshots of your worka) Write a Java program, which prints numbers 1 to 10 then terminates.b) Write a simple Java program that prints the days of the week.c) Daniel has the following data (10,20,30,40,50,60,70,80,90,100), he wants to print all the data except40,50,and 60. Write a Java program to guide him on how he can go about it.d) Write a java program that prints the months of the year that have 31days.arrow_forward) Write a Java method that prints integers from 1 to 100 (see the output). Use the following output as a guide:arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
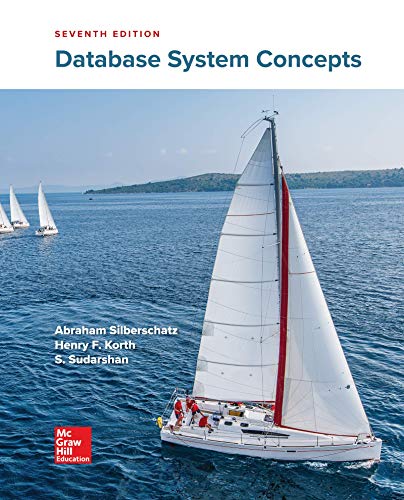
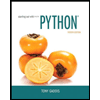
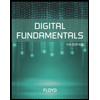
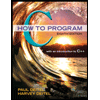
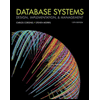
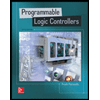