Write a Java program to perform the following task: First define an Employee class which includes employee's name, salary and hire date. The hire date contains three integers day, month and year. The Employee class contains methods to be able to access employee's name, salary and hire date. An employee can be created from the Employee class in one of the three ways: name only; or name and salary only; or name, salary and hire date of the employee. Creating an employee without any information is not allowed. Second, define a Supervisor class (subclass of the employee class). A supervisor is an employee with a year-end bonus and a status level: L for lower level manager and U for upper level position. In the main method in your program, declare an array of to hold at least ten employees (called emp ary) which will be used to hold employee records. Enter the following employee information according to the instruction Name Salary Hire Date Supervisor Level Bonus| Lori Green Mary Spade Joe Smith 50,000 47,000 54,000 63,000 41,000 65,000 3/4/2000 11/2/2001 5/7/1999 Jeff Berger April Apple John Smith 8/2/1995 4/23/2002 6/30/2003 yes L 5,000 7,000 Ginny Fernandez 69,000 Paul Fullerton 1/20/2001 U yes 2/27/2004 71,000 yes L 4,500 a). Enter the first three records at the time these three employee objects (records) are created using the appropriate class constructor. b). Enter the name for the fourth and fifth record at the time the employee object (record) is created using the appropriate class constructor, and then use class methods to enter salary and hire date information from keyboard after the employee objects are created. c). Enter the remaining records of employee using the appropriate class constructor, and then use class methods to enter the rest fields (level and bonus) from keyboard. d). Now calculate the average salary of these employees and print the average. e) Sort the employee records by salary and print all employee records (name, salary and hire date as shown above) in descending order in salary. f). Resort the records by the income (including bonus) and print the result in descending order in income.
Write a Java program to perform the following task: First define an Employee class which includes employee's name, salary and hire date. The hire date contains three integers day, month and year. The Employee class contains methods to be able to access employee's name, salary and hire date. An employee can be created from the Employee class in one of the three ways: name only; or name and salary only; or name, salary and hire date of the employee. Creating an employee without any information is not allowed. Second, define a Supervisor class (subclass of the employee class). A supervisor is an employee with a year-end bonus and a status level: L for lower level manager and U for upper level position. In the main method in your program, declare an array of to hold at least ten employees (called emp ary) which will be used to hold employee records. Enter the following employee information according to the instruction Name Salary Hire Date Supervisor Level Bonus| Lori Green Mary Spade Joe Smith 50,000 47,000 54,000 63,000 41,000 65,000 3/4/2000 11/2/2001 5/7/1999 Jeff Berger April Apple John Smith 8/2/1995 4/23/2002 6/30/2003 yes L 5,000 7,000 Ginny Fernandez 69,000 Paul Fullerton 1/20/2001 U yes 2/27/2004 71,000 yes L 4,500 a). Enter the first three records at the time these three employee objects (records) are created using the appropriate class constructor. b). Enter the name for the fourth and fifth record at the time the employee object (record) is created using the appropriate class constructor, and then use class methods to enter salary and hire date information from keyboard after the employee objects are created. c). Enter the remaining records of employee using the appropriate class constructor, and then use class methods to enter the rest fields (level and bonus) from keyboard. d). Now calculate the average salary of these employees and print the average. e) Sort the employee records by salary and print all employee records (name, salary and hire date as shown above) in descending order in salary. f). Resort the records by the income (including bonus) and print the result in descending order in income.
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter11: Inheritance And Composition
Section: Chapter Questions
Problem 6PE
Related questions
Question
Help me with my Java

Transcribed Image Text:Write a Java program to perform the following task:
First define an Employee class which includes employee's name, salary and hire date. The hire date contains three integers day,
month and year. The Employee class contains methods to be able to access employee's name, salary and hire date. An employee
can be created from the Employee class in one of the three ways: name only; or name and salary only; or name, salary and hire
date of the employee. Creating an employee without any information is not allowed.
Second, define a Supervisor class (subclass of the employee class). A supervisor is an employee with a year-end bonus and a
status level: L for lower level manager and U for upper level position.
In the main method in your program, declare an array of to hold at least ten employees (called emp ary) which will be used to
hold employee records. Enter the following employee information according to the instruction
Name
Salary
Hire Date Supervisor
Level
Bonus
Lori Green
50,000
47,000
54,000
63,000
41,000
65,000
3/4/2000
11/2/2001
Mary Spade
Joe Smith
5/7/1999
Jeff Berger
April Apple
John Smith
8/2/1995
4/23/2002
6/30/2003
yes
L
5,000
Ginny Fernandez 69,000
Paul Fullerton
1/20/2001
U
7,000
yes
2/27/2004
71,000
yes
L
4,500
a). Enter the first three records at the time these three employee objects (records) are created using the appropriate class
constructor.
b). Enter the name for the fourth and fifth record at the time the employee object (record) is created using the appropriate class
constructor, and then use class methods to enter salary and hire date information from keyboard after the employee objects are
created.
c). Enter the remaining records of employee using the appropriate class constructor, and then use class methods to enter the rest
fields (level and bonus) from keyboard.
d). Now calculate the average salary of these employees and print the average.
e) Sort the employee records by salary and print all employee records (name, salary and hire date as shown above) in descending
order in salary.
f). Resort the records by the income (including bonus) and print the result in descending order in income.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 10 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
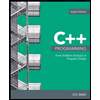
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
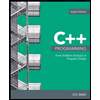
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning