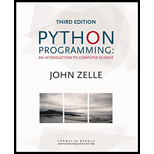
Draw a face
Program plan:
- Import the header file.
- Define the “drawFace” method
- Get the “x” and “y” positions
- Get the “p1” and “p2” positions
- Call the “Oval” method for drawing the face
- Set the color to the face
- Draw the face
- Get the “lc” and “rc” position
- Call the “Circle” method
- Set the color to the face
- Call the “draw” method
- Call the “Circle” method
- Set the color to the face
- Call the “draw” method
- Get the “m1”, “m2”, and “m3” values
- Call the “Rectangle” method
- Set the color to the face
- Call the “draw” method
- Call the “Line” method
- Get the center position
- Get the “x” and “y” position
- Call the “Point” method
- Call the “Line” method
- Call the “draw” method
- Get the “x” and “y” position for different variables
- Calculate “t” and “t1” value
- Call the “Rectangle” method
- Call the “draw” method
- Iterate “i” until it reaches 7
- Clone the teeth by calling “clone” method
- Move the face by calling “move” method
- Call the “draw” method
- Define the main method.
- Read the filename from the user
- Get the image width and height from the file
- Set the graph win size
- Set the coords for graph
- Draw the face
- Get the number of faces from the user
- Iterate “i” until it reaches “n” value
- Get the mouse action
- Call the “drawFace” method
- Call the function “main()”.

This Python program is used to get the GIF (Graphic Interchange format) file and draw the smileys when the user clicking the GIF image.
Explanation of Solution
Program:
#import the header file
from graphics import *
#definition of "drawFace" method
def drawFace(center, size, window):
#get the "x" value
x1 = center.getX()
#get the "y" value
y1 = center.getY()
#get the "p1" value
p1 = Point(x1-(.7 * size), y1 - size)
#get the "p2" value
p2 = Point(x1+(.7 * size), y1 + size)
#call the "Oval" method
head = Oval(p1, p2)
#fill the color
head.setFill("white")
#call the "draw" method
head.draw(window)
#get the "lc" value
lc = Point(x1 - .2 * size, y1 + .6 * size)
#get the "rc" value
rc = Point(x1 + .2 * size, y1 + .6 * size)
#call the "Circle" method
leftEye = Circle(lc, .13 * size)
#fill the color
leftEye.setFill("white")
#call the "draw" method
leftEye.draw(window)
#call the "Circle" method
rightEye = Circle(rc, .13 * size)
#fill the color
rightEye.setFill("white")
#call the "draw" method
rightEye.draw(window)
#get the "m1" value
m1 = Point(x1 - .3 * size, y1 - .5 * size)
#get the "m2" value
m2 = Point(x1 + .3 * size, y1 - .25 * size)
#get the "m3" value
m3 = Point(x1 + .3 * size, y1 - .5 * size)
#call the "Rectangle" method
mouth = Rectangle(m1, m2)
#fill the color
mouth.setFill("white")
#call the "draw" method
mouth.draw(window)
#call the "Line" method
leftLip = Line(m1, Point(x1- .3 * size, y1 - .25 * size))
#get the center position
mLeftCent = leftLip.getCenter()
#get the "x" position
mLCx = mLeftCent.getX()
#get the "y" position
mLCy = mLeftCent.getY()
#call the "Point" method
mRightCent = Point(x1 + .3 * size, mLCy)
#call the "Line" method
lip = Line(mLeftCent, mRightCent)
#call the "draw" method
lip.draw(window)
#get the "x" positions
m1x = m1.getX()
m3x = m3.getX()
#get the "y" positions
m1y = m1.getY()
m2y = m2.getY()
#calculate the "t" value
t = m1x - m3x
#calculate the "t1" value
t1 = Point(m1x - (1/8 * t), m2y)
#call the "Rectangle" method
teeth = Rectangle(m1, t1)
#call the "draw" method
teeth.draw(window)
#iterate "i" until it reaches 7
for i in range (7):
#clone the face
t2 = teeth.clone()
#call the "move" method
t2.move(-i * (1/8 * t), 0)
#call the "draw" method
t2.draw(window)
#definition of main method
def main():
#get the input filename from the user
fname = input("Enter filename: ")
infile = Image(Point(10, 10), fname)
#get the width of the image
wWidth = infile.getWidth()
#get the height of the image
wHeight = infile.getHeight()
#set graph win name
window = GraphWin('Smile!', wWidth,wHeight)
#set the coords
window.setCoords(0, 0, 20, 20)
#draw a smiley
infile.draw(window)
#get how many faces the user wants to draw
n = eval(input("How many faces should we block? "))
#iterate "i" until it reaches "n"
for i in range(n):
#get the mouse action
point = window.getMouse()
#draw a face by calling the method "drawFace"
drawFace(point, 3, window)
#call the "main" method
main()
Output:
Enter filename: test_img.gif
How many faces should we block? 2
>>>
Screenshot of “Smile!” window
Want to see more full solutions like this?
Chapter 6 Solutions
Python Programming: An Introduction to Computer Science, 3rd Ed.
- Task: Loading data from files This exercise will require you to load some information from files and use it in your program. scene.txt contains a description of a series of shapes and colours to draw. You need to write code to read in the file data and draw the requested shapes in the correct colour. Each line in scene.txt will contain one of the following starting keywords followed by some data: COLOUR followed by 3 values: R, G, B CIRCLE followed by 3 values: X, Y, RADIUS RECT Followed by 4 values: X, Y, W, H LINE Followed by 4 values: X1, Y1, X2, Y2 CIRCLES are defined from the center. RECT's are defined from the top left. All values are space separated, and you can assume all input is correct (no errors). Please solve this program to draw the scene. I can't upload the file scene.txt, so I decide to screenshot a file for you. Subject: Java Programmingarrow_forward1. In the first loop, the low number will be the starting point for the loop and thehigh will be the ending point. The loop should display the iterator number andthat number x 10 on the same line, separated by a tab. See example on p. 177.2. The second loop should accumulate all the numbers between the starting pointand ending point. You will need to create and initialize an accumulator such astotal before you start the loop.arrow_forwardA Personal Fitness Tracker is a wearable device that tracks your physical activity, calories burned, heart rate, sleeping patterns, and so on. One common physical activity that most of these devices track is the number of steps you take each day. The steps.txt file contains the number of steps a person has taken each day for a year. There are 365 lines in the file, and each line contains the number of steps taken during a day. (The first line is the number of steps taken on January 1st, the second line is the number of steps taken on January 2nd, and so forth.) Write a program that reads the file, then displays the average number of steps taken for each month. (The data is from a year that was not a leap year, so February has 28 days.) You don't need to see the file , Just write a code using the "file" like this def main(): with open("steps.txt", "r") as file:arrow_forward
- • Use a while loop • Use multiple loop controlling conditions • Use a boolean method • Use the increment operator • Extra credit: Reuse earlier code and call two methods from main Details: This assignment will be completed using the Eclipse IDE. Cut and paste your code from Eclipse into the Assignment text window. This is another password program. In this case, your code is simply going to ask for a username and password, and then check the input against four users. The program will give the user three tries to input the correct username-password combination. There will be four acceptable user-password combinations: • alpha-alpha1 • beta-beta1 • gamma-gamma1 • delta - delta1 If the user types in one of the correct username-password combinations, then the program will output: "Login successful." Here are a couple of example runs (but your code needs to work for all four user-password combinations): Username: beta Type your current password: beta1 Login successful. Username: delta Type…arrow_forwardIn this lab, you write a while loop that uses a sentinel value to control a loop in a Python program. You also write the statements that make up the body of the loop. The source code file already contains the necessary assignment and output statements. Each theater patron enters a value from 0 to 4 indicating the number of stars that the patron awards to the Guide’s featured movie of the week. The program executes continuously until the theater manager enters a negative number to quit. At the end of the program, you should display the average star rating for the movie. Instructions Make sure the file MovieGuide.pyis selected and open. Write thewhile loop using a sentinel value to control the loop, and also write the statements that make up the body of the loop. Execute the program by clicking the Run button at the bottom of the screen. Input the following as star ratings: 0, 3, 4, 4, 1, 1, 2, -1 Ensure that the average output is correct.arrow_forwardIn this lab, you write a while loop that uses a sentinel value to control a loop in a Python program. You also write the statements that make up the body of the loop. The source code file already contains the necessary assignment and output statements. Each theater patron enters a value from 0 to 4 indicating the number of stars that the patron awards to the Guide’s featured movie of the week. The program executes continuously until the theater manager enters a negative number to quit. At the end of the program, you should display the average star rating for the movie.arrow_forward
- Using a Sentinel Value to Control a while Loop Summary In this lab, you write a while loop that uses a sentinel value to control a loop in a Java program that has been provided. You also write the statements that make up the body of the loop. The source code file already contains the necessary variable declarations and output statements. Each theater patron enters a value from 0 to 4 indicating the number of stars the patron awards to the Guide’s featured movie of the week. The program executes continuously until the theater manager enters a negative number to quit. At the end of the program, you should display the average star rating for the movie. Instructions Ensure the file named MovieGuide.java is open. Write the while loop using a sentinel value to control the loop, and write the statements that make up the body of the loop to calculate the average star rating. Execute the program by clicking Run. Input the following: 0, 3, 4, 4, 1, 1, 2, -1 Check that the average…arrow_forwardA for statement is a loop that goes through a list of things. Therefore, it keeps running as long as there are objects to process. Is this a true or a false statement?arrow_forwardVirus DNA files As a future doctor, Jojo works in a laboratory that analyzes viral DNA. Due to the pandemic During the virus outbreak, Jojo received many requests to analyze whether there was any viral DNA in the patient. This number of requests made Jojo's job even more difficult. Therefore, Jojo ask Lili who is a programmer for help to make a program that can read the file containing the patient's DNA data and viral DNA and then match them. If on the patient's DNA found the exact same string pattern, then write to the index screen the found DNA. Data contained in the file testdata.in Input Format The first line of input is the number of test cases TThe second row and so on as many as T rows are the S1 string of patient DNA and the S2 string of viral DNA separated by spaces Output Format The array index found the same string pattern. Constraints 1 ≤ T ≤ 1003 ≤ |S2| ≤ |S1| ≤ 100|S| is the length of the string.S will only consist of lowercase letters [a-z] Sample Input (testdata.in)…arrow_forward
- Please I really need help with this question it is confusing me a lot I don't understand how should I start, Also can you please attach the TEXT FILE Screenshot. PLEASE! Write a program that allows the user to navigate lines of text in a file. The program should prompt the user for a filename, read the file and input the lines of text into a list. The program then enters a loop in which it prints the number of lines in the file and prompts the user for a line number. Actual line number range from 1 to the number of lines in the file. If the input is 0, the program quits. Otherwise, the program prints the text associated with that number. You must also run the script and provide a screenshot of the output along with the textfile.arrow_forwardIt is possible to create a for loop that processes a list of things. Consequently, it continues to operate as long as there are objects to be processed by the system. Is this statement correct or incorrect?arrow_forwardIn C++ (don't copy paste the same answer that is already on this site, post unique code) Structures A menu-driven program gives the user the option to find statistics about different baseball players. The program reads from a file and stores the data for ten baseball players, including player’s team, name of player, number of homeruns, batting average, and runs batted in. (You can make up your data, or get it online ) Write a program that declares a struct to store the data for a player. Declare an array of 10 components to store the data for 10 baseball players. The program prints out a menu (in a loop, so this can be done again and again) giving the user a choice to: print out all users and statistics print out the statistics for a specific player print out all data for a specific team update the data for a particular player (change one of the statistics) DO ALL WORK IN FUNCTIONS. USE A FUNCTION TO READ THE DATA, A FUNCTION TO PRINT THE MENU, and FUNCTIONS FOR EACH OF THE MENU…arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
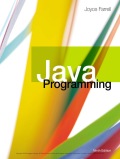
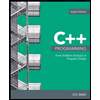