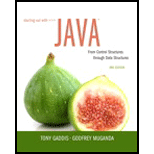
Starting Out with Java: From Control Structures through Data Structures (3rd Edition)
3rd Edition
ISBN: 9780134038179
Author: Tony Gaddis, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 20, Problem 2PC
Program Plan Intro
Linked List Sorting and Reversing
Program Plan:
For filename: Modified “LinkedList1.java”
- In this class, additionally need to add two methods “sort()” and “reverse()”.
- Define the method “sort()” which is used to sort the elements in the list by alphabetical order.
- Create two variables “current_node” and “index_value” using “Node” class and this variable set to “null”.
- Declare a string variable “temp_value”.
- Check whether list is empty. If the list is empty, then returns nothing.
- Otherwise,
- Check condition using “for” loop. That is the current node will point to first.
- Check the condition using “for” loop that is for index value will point to next node of current node.
- If data in the “current_node” is greater than the data in “index_value”, then swap the data of “current_node” and “index_index”.
- Check the condition using “for” loop that is for index value will point to next node of current node.
- Check condition using “for” loop. That is the current node will point to first.
- Define the method “reverse()” which is used to reverse the elements in the list.
- Create a list “rev_list” and set it to “null”.
- Performs “while” loop. This loop will perform up to the first node equals to “null”.
- Set a reference “ref” to first node.
- Set “first” to next first node.
- Set next node to “rev_list”.
- Set the “rev_list” to reference “ref”.
- Make the list “rev_list” as the new list.
For filename: “LinkedList1Demo.java”:
- Import required package.
- Define “LinkedList1Demo” class.
- Create an instance for “LinkedList1” class, text area, command text field, and result text field.
- Define constructor for “LinkedList1Demo()” class.
- Create instance.
- Create a panel for “Command Result” text field.
- Create a label for command result.
- Place the text area in center of the frame.
- Create a panel and label for “Command” text field.
- Set up the frame.
- Define private class “commandTextListener” that reply to the user entered command.
- Define “actionPerformed” class.
- Read the command from the command text field.
- Create an object for “Scanner” class.
- Read command from user.
- Check the user entered command using “switch” statement.
- If the user entered command is “sort”, then,
-
- Sort the linked list “new_list” by calling the method “sort()”.
- Displays lists in alphabetical order.
- Assign a status message for the “sort” command was finished.
- Displays status message to “Command Result” text field.
- If the user entered command is “reverse”, then
-
- Reverse the linked list “new_list” by calling the method “reverse()”.
- Prints the reversed list.
- Assign a status message for the “reverse” command was finished.
- Displays status message to “Command Result” text field.
- If the user entered command is “add”, then
-
- Check condition. If it is true, then
- Read index and string element from user.
- Add that index and its element to linked list “new_list” by calling the method “add”.
- Otherwise,
- Read string element from user.
- Add string element to the list “new_list” by calling the method “add”.
- Display the added string elements.
- Check condition. If it is true, then
- If the user entered command is “remove”, then
-
- Check condition. If it is true, then
- Read an index from user.
- Remove an element at given index.
- Display the removed element at given index to “Command Result” text field.
- Otherwise,
- Read an element from the list.
- Obtain the Boolean result.
- Convert the Boolean into string.
- Display the Boolean result to “Command Result” text field.
- Displays string elements in the list
- Check condition. If it is true, then
- If the user entered command is “isEmpty”, then
-
- Check the given list is empty or not by calling the method “isEmpty” and store the result in “result_text3”.
- Display the result to “Command Result” text field.
- If the user entered command is “size”, then
-
- Compute the size of list “new_list” by calling the method “size()” and store the result in “result_text4”.
- Display the result to “Command Result” text field.
- Define “actionPerformed” class.
- Define main function.
- The instance of “LinkedList1Demo” class displays its window.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
please follow instructions correctly.
You are required to complete the LinkedList class. This class is used as a linked list that has many methods to perform operations on the linked list. To create a linked list, create an object of this class and use the addFirst or addLast or add(must be completed) to add nodes to this linked list. Your job is to complete the empty methods. For every method you have to complete, you are provided with a header, Do not modify those headers(method name, return type or parameters). You have to complete the body of the method.
package chapter02;
public class LinkedList
{
protected LLNode list;
public LinkedList()
{
list = null;
}
public void addFirst(T info)
{
LLNode node = new LLNode(info);
node.setLink(list);
list = node;
}
public void addLast(T info)
{
LLNode curr = list;
LLNode newNode = new LLNode(info);
if(curr == null)
{
list = newNode;
}
else…
Question 2: Linked List Implementation
You are going to create and implement a new Linked List class. The Java Class name is
"StringLinkedList". The Linked List class only stores 'string' data type. You should not change the
name of your Java Class. Your program has to implement the following methods for the
StringLinked List Class:
1. push(String e) - adds a string to the beginning of the list (discussed in class)
2. printList() prints the linked list starting from the head (discussed in class)
3. deleteAfter(String e) - deletes the string present after the given string input 'e'.
4. updateToLower() - changes the stored string values in the list to lowercase.
5. concatStr(int p1, int p2) - Retrieves the two strings at given node positions p1 and p2. The
retrieved strings are joined as a single string. This new string is then pushed to the list
using push() method.
Write a Python code using the given function and conditions. Do not use Numpy. Use LinkedList Manipulation.
Given function: def insert(self, newElement, index)
Pre-condition: The list is not empty.
Post-condition: This method inserts newElement at the given index of the list. If an element with the same key as newElement value already exists in the list, then it concludes the key already exists and does not insert the key. [You must also check the validity of the index].
Chapter 20 Solutions
Starting Out with Java: From Control Structures through Data Structures (3rd Edition)
Ch. 20.1 - Prob. 20.1CPCh. 20.1 - Prob. 20.2CPCh. 20.3 - Prob. 20.4CPCh. 20 - A list is a collection that _____. a. associates...Ch. 20 - Prob. 2MCCh. 20 - Prob. 3MCCh. 20 - Prob. 4MCCh. 20 - Prob. 5MCCh. 20 - Prob. 6MCCh. 20 - Prob. 7MC
Ch. 20 - Prob. 11TFCh. 20 - Prob. 12TFCh. 20 - Prob. 13TFCh. 20 - Prob. 14TFCh. 20 - Prob. 15TFCh. 20 - Prob. 16TFCh. 20 - Prob. 17TFCh. 20 - Prob. 18TFCh. 20 - Prob. 29TFCh. 20 - Prob. 20TFCh. 20 - Prob. 1FTECh. 20 - Prob. 2FTECh. 20 - Prob. 3FTECh. 20 - Prob. 4FTECh. 20 - Prob. 5FTECh. 20 - Prob. 1AWCh. 20 - Prob. 2AWCh. 20 - Prob. 3AWCh. 20 - Prob. 4AWCh. 20 - Prob. 3SACh. 20 - Prob. 4SACh. 20 - Prob. 5SACh. 20 - Consult the online Java documentation and...Ch. 20 - Prob. 1PCCh. 20 - Prob. 2PC
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Direction: Continue the code below and add case 4, case 5, and case 6. Add 3 more functions aside from insert, getValue, and clear from List ADT import java.util.LinkedList; import java.util.Scanner; class SampleLL { } public static void main(String[] args) { LinkedList 11s = new LinkedList(); String msg = "Choose a function: \n [1] Insert, [2]Get Value, [3]Clear, [0] Exit"; System.out.println(msg); Scanner scan= new Scanner(System.in); int choice scan.nextInt (); while(true) { } if (choice =0) { } System.exit(0); switch(choice) { } case 1: System.out.println("Enter a word/symbol:"); break; case 2: System.out.println("Enter a number: "); break; 11s.add(scan.next()); break; case 3 11s.clear(); default: System.out.println("Invalid input!"); break; System.out.println(11s.get (scan.nextInt())); System.out.println(msg); choice scan.nextInt ();arrow_forwardC# Assume you have a LinkedList of Node objects. Both classes have all the normal operations shown below. Your job is to program the DeleteTail method of the LinkedList class. This method locates and deletes the last element of the linked list. You may not change its signature line. Keep your code clean, but no documentation is necessary. A good solution will be between 5 and 10 lines of code, not counting whitespace.arrow_forwardMake a doubly linked list and apply all the insertion, deletion and search cases. The node willhave an int variable in the data part. Your LinkedList will have a head and a tail pointer.Your LinkedList class must have the following functions: 1) insert a node1. void insertNodeAtBeginning(int data);2. void insertNodeInMiddle(int key, int data); //will search for keyand insert node after the node where a node’s data==key3. void insertNodeAtEnd(int data);2) delete a node1. bool deleteFirstNode(); //will delete the first node of the LL2. bool deleteNode(int key); //search for the node where its data==keyand delete that particular node3. bool deleteLastNode(); //will delete the last node of the LL3) Search a node1. Node* searchNodeRef(int key); //will search for the key in the datapart of the node2. bool searchNode(int key); The program must be completely generic, especially for deleting/inserting the middle nodes. Implement all the functions from the ABOVE STATEMENTS and make a login and…arrow_forward
- Instruction: To test the Linked List class, create a new Java class with the main method, generate Linked List using Integer and check whether all methods do what they’re supposed to do. A sample Java class with main method is provided below including output generated. If you encounter errors, note them and try to correct the codes. Post the changes in your code, if any. Additional Instruction: Linked List is a part of the Collection framework present in java.util package, however, to be able to check the complexity of Linked List operations, we can recode the data structure based on Java Documentation https://docs.oracle.com/javase/8/docs/api/java/util/LinkedList.html package com.linkedlist; public class linkedListTester { public static void main(String[] args) { ListI<Integer> list = new LinkedList<Integer>(); int n=10; for(int i=0;i<n;i++) { list.addFirst(i); } for(int…arrow_forwardIf N represents the number of elements in the collection, then the contains method of the ArrayCollection class is O(1). True or False If N represents the number of elements in the list, then the index-based add method of the ABList class is O(N). True or Falsearrow_forwardChu Bethany Daryl next pext next bead The above is a LinkedList. 3. Why do you not move the head node-reference and set up the p node-reference to iterate the moving from one node to the next node while you are inserting, removing nodes or printing a list? 4. What is the advantage of using a LinkedList over an ArrayList? Nextarrow_forward
- PYTHON LAB: Inserting an integer in descending order (doubly-linked list) Given main.py and an IntNode class, complete the IntList class (a linked list of IntNodes) by writing the insert_in_descending_order() method to insert new IntNodes into the IntList in descending order. Ex. If the input is: 3 4 2 5 1 6 7 9 8 the output is: 9 8 7 6 5 4 3 2 1 _____________________________________________________________________________________ Main.py: from IntNode import IntNode from IntList import IntList if __name__ == "__main__": int_list = IntList() input_line = input() input_strings = input_line.split(' ') for num_string in input_strings: # Convert from string to integer num = int(num_string) # Insert into linked list in descending order new_node = IntNode(num) int_list.insert_in_descending_order(new_node) int_list.print_int_list() IntNode.py class IntNode: def __init__(self, initial_data, next = None,…arrow_forwardQuestion 1 If N represents the number of elements in the collection, then the add method of the SortedArrayCollection class is O(N). True False Question 2 If N represents the number of elements in the list, then the index-based remove method of the ABList class is O(N). True False Question 3 The add method of our Collection ADT might throw the CollectionOverflowException. True False Question 4 A list allows retrieval of information based on the size of the information. True False Question 5 Our CollectionInterface defines a single constructor. True False Question 6 Our lists allow null elements. True False Question 7 Even though our collections will be generic, our CollectionInterface is not generic. True False Question 8 Our lists are unbounded. True False Question 9 If N represents the number of elements in the collection, then the contains method of the ArrayCollection class is O(N). True False Question 10 Our lists allow duplicate elements. True Falsearrow_forwardpackage Linked_List; public class RefUnsortedList<T> implements ListInterface<T>{protected int numElements; // number of elements in this listprotected LLNode<T> currentPos; // current position for iteration// set by find methodprotected boolean found; // true if element found, else falseprotected LLNode<T> location; // node containing element, if foundprotected LLNode<T> previous; // node preceeding locationprotected LLNode<T> list; // first node on the listpublic RefUnsortedList(){numElements = 0;list = null;currentPos = null;}public void add(T element)// Adds element to this list.{LLNode<T> newNode = new LLNode<T>(element);newNode.setLink(list);list = newNode;numElements++;}protected void find(T target)// Searches list for an occurence of an element e such that// e.equals(target). If successful, sets instance variables// found to true, location to node containing e, and previous// to the node that links to location. If not successful,…arrow_forward
- package Linked_List; public class RefUnsortedList<T> implements ListInterface<T> {protected int numElements; // number of elements in this listprotected LLNode<T> currentPos; // current position for iteration// set by find methodprotected boolean found; // true if element found, else falseprotected LLNode<T> location; // node containing element, if foundprotected LLNode<T> previous; // node preceeding locationprotected LLNode<T> list; // first node on the listpublic RefUnsortedList(){numElements = 0;list = null;currentPos = null;}public void add(T element)// Adds element to this list.{LLNode<T> newNode = new LLNode<T>(element);newNode.setLink(list);list = newNode;numElements++;}protected void find(T target)// Searches list for an occurence of an element e such that// e.equals(target). If successful, sets instance variables// found to true, location to node containing e, and previous// to the node that links to location. If not successful,…arrow_forwardo create the linkage for the nodes of our linkedlist. The class includes several methods for adding nodes to the list, removingnodes from the list, traversing the list, and finding a node in the list. We alsoneed a constructor method that instantiates a list. The only data member inthe class is the header node.use c#arrow_forwardjava program java method: Write a method replace to be included in the class KWLinkedList (for doubly linked list) that accepts two parameters, searchItem and repItem of type E. The method searches for searchItem in the doubly linked list, if found then replace it with repItem and return true. If the searchItem is not found in the doubly linked list, then insert repItem at the end of the linked list and return false. Assume that the list is not empty. You can use ListIterator and its methods to search the searchItem in the list and replace it with repItem if found. Do not call any method of class KWLinkedList to add a new node at the end of the list. Method Heading: public boolean replace(E searchItem, E repItem) Example: searchItem: 15 repItem: 17 List (before method call): 9 10 15 20 4 5 6 List (after method call) : 9 10 17 20 4 5 6arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
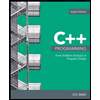
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
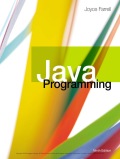
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT