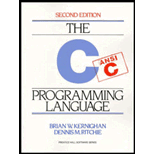
C Programming Language
2nd Edition
ISBN: 9780131103627
Author: Brian W. Kernighan, Dennis M. Ritchie, Dennis Ritchie
Publisher: Prentice Hall
expand_more
expand_more
format_list_bulleted
Question
Chapter 2, Problem 1E
Program Plan Intro
- Initialize the header files and main() function.
- To write the statements for printing the ranges of data types int, long, char, short, char, signed, and unsigned using the header file limits.h.
- Use the printf() statement to print all these.
Summary Introduction- The program prints the range of data types int, long, char, short, char, signed, and unsigned.
Program Description- The purpose of the program is toprint the range of data types int, long, char, short, char, signed, and unsigned.
Expert Solution & Answer

Explanation of Solution
Test Program:
/* Program: The program to display the range of data types. */ #include <stdio.h> #include <limits.h> intmain() { printf("bits: %d\n", CHAR_BIT); printf("unsigned char max: %d\n", UCHAR_MAX); printf("char max: %d\n", CHAR_MAX); printf("char min: %d\n", CHAR_MIN); printf("\n"); printf("unsigned int max: %u\n", UINT_MAX); printf("int min: %d\n",INT_MIN); printf("int max: %d\n",INT_MAX); printf("\n"); printf("unsigned short int max: %u\n", USHRT_MAX); printf("short int min: %d\n", SHRT_MIN); printf("short int max: %d\n", SHRT_MAX); printf("\n"); printf("unsigned long int max: %lu\n", ULONG_MAX); printf("long int min: %ld\n",LONG_MIN); printf("long int max: %ld\n",LONG_MAX); printf("\n"); return0; }
Sample Output -
bits: 8
unsigned char max: 255
char max: 127
char min: -128
unsigned int max: 4294967295
int min: -2147483648
int max: 2147483647
unsigned short int max: 65535
short int min: -32768
short int max: 32767
unsigned long int max: 4294967295
long int min: -2147483648
long int max: 2147483647
Want to see more full solutions like this?
Subscribe now to access step-by-step solutions to millions of textbook problems written by subject matter experts!
Students have asked these similar questions
A pedometer treats walking 1 step as walking 2.5 feet. Define a function named feet_to_steps that takes a float as a parameter, representing the number of feet walked, and returns an integer that represents the number of steps walked. Then, write a main program that reads the number of feet walked as an input, calls function feet_to_steps() with the input as an argument, and outputs the number of steps.
Use floating-point arithmetic to perform the conversion.
Ex: If the input is:
150.5
the output is:
60
The program must define and call the following function:def feet_to_steps(user_feet)
# Define your function here
if __name__ == '__main__': # Type your code here.
python
A pedometer treats walking 1 step as walking 2.5 feet. Define a function named feet_to_steps that takes a float as a parameter, representing the number of feet walked, and returns an integer that represents the number of steps walked. Then, write a main program that reads the number of feet walked as an input, calls function feet_to_steps() with the input as an argument, and outputs the number of steps.
Use floating-point arithmetic to perform the conversion.
Ex: If the input is:
150.5
the output is:
60
The program must define and call the following function:def feet_to_steps(user_feet)
my code(python):
def feet_to_steps(user_feet): steps = user_feet / 2.5 return steps
if __name__ == '__main__': user_feet = float(input())print(round(feet_to_steps(user_feet)))
How do I pass the unit test?
Using language C++ and includes the knowledge of data structure Do it by your own pls
Description
Given n non-negative numbers, you need to output the non-zero numbers in reverse order.
Input
The input data contains multiple test cases. The first line of the input is an integer T, indicating
the number of test cases.
The first line of each test case contains an integer n. The second line contains 72 non-negative
integers.
Output
For each test case, output the non-zero numbers in reverse order in a line. Simply print a blank
line if there is no positive number.
Sample Input/Output
Input
2
5
0 0 0 0 0
9
998244053
Output
35442899
Chapter 2 Solutions
C Programming Language
Ch. 2 - Prob. 1ECh. 2 - Write a loop equivalent to the for loop above...Ch. 2 - Write the function htoi(s), which converts a suing...Ch. 2 - Write an alternate version of squeeze(s1,s2) that...Ch. 2 - Prob. 5ECh. 2 - Prob. 6ECh. 2 - Prob. 7ECh. 2 - Prob. 8ECh. 2 - Prob. 9ECh. 2 - Rewrite the function lower, which converts upper...
Knowledge Booster
Similar questions
- (Numerical) Using the srand() and rand() C++ library functions, fill an array of 1000 floating-point numbers with random numbers that have been scaled to the range 1 to 100. Then determine and display the number of random numbers having values between 1 and 50 and the number having values greater than 50. What do you expect the output counts to be?arrow_forward(Practice) Write specification statements for the following: a. An array of integers with 6 rows and 10 columns b. An array of integers with 2 rows and 5 columns c. An array of characters with 7 rows and 12 columns d. An array of characters with 15 rows and 7 columns e. An array of double-precision numbers with 10 rows and 25 columns f. An array of double-precision numbers with 16 rows and 8 columnsarrow_forwardPython Programming- Computer-Assisted Instruction Part 1: Computer-Assisted Instruction (CAI) refers to the use of computers in education. Write a script to help an elementary school student learn multiplication. Create a function that randomly generates and returns a tuple of two positive one-digit integers. Use that function's result in your script to prompt the user with a question, such as: How much is 6 times 7? For a correct answer, display the message "Very good!" and ask another multiplication question. For an incorrect answer, display the message "No. Please try again." and let the student try the same question repeatedly until the student finally gets it right. Part 2: Varying the computer's responses can help hold the student's attention. Modify the program so that various comments are displayed for each answer. Possible responses to a correct answer should include 'Very good!' , 'Nice work!' and 'Keep up the good work!' Possible responses to an incorrect answer shojld…arrow_forward
- With the aid of c++, write a program which utilizes a random number generator to bring out(generate) a 2 digits +(positive) integer and permits the user to do 1 or more of the operations listed below.Solve /expresss the following. i.Reverse digits of the number ii.If the number has an exponent of 2,3 or 4 ii.Considering the number is a 3 digits number with its last digit <=4 and the exponent of the 1st 2 digits being that of the last digit N.b The 'number' word used above represents the randomly generated 2 digits +(positive) integer. a.In case the random number generated is less than 10,add ten to the number. b.Check if the number is a prime after each operation c.The code shouldn't be made up of global variables and also each of these operations should be implemented by a separate function d.Code should be menu driven and each successive operation must be performed on the number generated by the last operation. e.There should be a creation of an enumeration type called selection…arrow_forward: Write a program in C++ to input attendance as 0 (for absent) and 1 (for present) for 25 students in 32 lectures and store it in a 2-D array. After the input, write loops for calculating and displaying the index numbers of the students who has short attendance (i.e. less than 75%). Output should be like this:Please enter attendance of all 25 students for given lecture (total 32 lectures):Enter attendance of Lecture “1”Student 1: <user enters 0 or 1>Student 2: <user enters 0 or 1> and so on….arrow_forwardPrograming C Just with #include Matrix Addition and Subtraction (Associatively) Write a program that performs matrices addition and subtraction. As shown in the example below, you have to ask the user for the number of rows and number of columns that both matrices should have (they both have to be the same size, that is why you only ask once). You will then ask the user for the values of each matrix. Then, you will print the values that the user inputted, the added values of both matrices, and the subtracted values (matrix 2 should be subtracted from matrix 1). In this challenge Guidelines/steps: • The number of rows and the number of columns should be global variables. • Once you get these two from the user, you should declare your two matrices in main. • Then, from main, you will call function aaa twice; first, you will send matrix 1 and populate it with values inputted by the user. You will call the function a second time, send matrix 2 and populate it. In other words, function aaa…arrow_forward
- A pedometer treats walking 1 step as walking 2.5 feet. Define a function named feet_to_steps that takes a float as a parameter, representing the number of feet walked, and returns an integer that represents the number of steps walked. Then, write a main program that reads the number of feet walked as an input, calls function feet_to_steps with the input as an argument, and outputs the number of steps as an integer. Use floating-point arithmetic to perform the conversion. Ex: If the input is: 150.5 the output is: 60 The program must define and call the following function: def feet_to_steps (user_feet) 461710.3116374.qx3zqy7 LAB ACTIVITY 7.10.1: LAB: Step counter 1 # Define your function here 3 if __name_ 4 '__main__': #Type your code here. main.py 0/10 Load default template...arrow_forwardWrite a simple calculator program with four operations: add, subtract, multiply, and divide. Create a separate function for each that returns the result of the operation (for example, an “add” function that returns the sum of two values). In the main() function, the program should ask the user to enter two numbers (floating point type) separated by a '+', '-', '*', or '/' character. The program should then call the appropriate function and display the result of the operation.arrow_forwardSecuring data is very important. We are developing an encryption module for the communication system in C++. You are supposed to input data in a character array of size 100. After taking input in array pass this array to a function to encrypt. This function applies following encryption on data. For testing purposes, after encryption function is called, the main function should print the encrypted data in the array. Convert upper case letters to lower case and lower case letters to upper case. After conversion replace each alphabet with its next alphabet, for example “A” will be replaced by “B”, “B” will be replaced by “C”, and so on. Similarly “a” will be replaced by “b” etc. However, “Z” should be replaced by “A” and “z” should be replaced by “a”. Digits must be replaced by subtracting it from 9 for example 0 should be replaced by 9 (9-0=9), 1 should be replaced by 8 (9-1=8), 2 should be replaced by 7 (9-2=7)………… and 9 should be replaced by 0 (9-9=0). Spaces should be replaced by $…arrow_forward
- Write a C++ program that declares an array alpha of 50 components of type double. Initialize the array so that the first 25 components are equal to the square of the index variable, and the last 25 components are equal to three times the index variable. Output the array so that 10 elements per line are printed. Use the array only and do not define any elements of the array in the program. All elements must be typed by the user. In the main program, only simple function calls will be allowed.arrow_forwardWrite a function that prints the ASCII values of the char- acters using the following header: void printASCII(char ch1, char ch2, int numberPerLine) This function prints the ASCII values of characters between ch1 and ch2 with the specified number of characters per line. Write a test program that prints 6 ASCII values per line of characters from 'a' to 'm'.arrow_forwardWrite a program to check the divisibility of two numbers. Use the concept of default argument here. If the number is not divisible, check whether the first number is a prime or not, using the previously defined divisibility check function.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
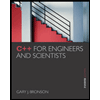
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
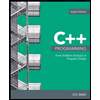
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning