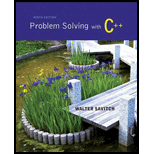
Problem Solving with C++ (9th Edition)
9th Edition
ISBN: 9780133591743
Author: Walter Savitch
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 17, Problem 6PP
Program Plan Intro
Priority queue
Program plan:
- Define a file “Priority.h” to declare operation and functions.
- Include the directives for header file.
- Declare a “PriorityQueue” template class.
- Define a template class named “Node”.
- Inside the access specifier “public”, define a constructor of class “Node”.
- Declare a friend class “PriorityQueue”.
- Inside the access specifier “private”, create an object for template.
- Declare an integer variable “priority” and create a pointer variable “*next”.
- Define a template class named “PriorityQueue”.
- Inside the access specifier “public”, Declare a constructor of class “PriorityQueue”.
- Declare the “add()” function to add items in the queue.
- Declare the “remove()” function to remove items from the queue.
- Declare the “isEmpty()” Boolean function to return the status of queue.
- Inside the access specifier “public”, Declare a constructor of class “PriorityQueue”.
- Define a file “main().cpp” to call functions from “Priority.h” and perform all computations.
- Define a constructor of class “PriorityQueue()” to assign “null” to “head” of the queue.
- Define “add()” function to add new node onto the front of the queue.
- Define “remove()” function to remove the smallest priority from the queue.
- Define “isEmpty()” function to return “true” or “false” if the queue is empty.
- Define a “main()” function.
- Create an object for class “PriorityQueue”.
- Add and remove some items in the queue and print the result.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Tour.java
Create a Tour data type that represents the sequence of points visited in a TSP tour. Represent the
tour as a circular linked list of nodes, one for each point in the tour. Each Node contains two
references: one to the associated Point and the other to the next Node in the tour. Each
constructor must take constant time. All instance methods must take time linear (or better) in the
number of points currently in the tour.
To represent a node, within Tour.java, define a nested class Node:
private class Node {
private Point p;
private Node next;
}
Your Tour data type must implement the following API. You must not add public methods to the
API; however, you may add private instance variables or methods (which are only accessible in the
class in which they are declared).
public class Tour
// Creates an empty tour.
public Tour()
// Creates the 4-point tour a→b→c→d→a (for debugging).
public Tour(Point a, Point b, Point c, Point d)
// Returns the number of points in this tour.
public…
Java Program
Consider a class Student that has an attribute age and a method getAge(): StudentList is a class representing a linkedlist of students. Write sumListAge_lterative......), a method that computes and returns the sum of the ages of all students in the list using the iterative way. Parameters of the method should be specified accordingly
Assignment 05 PART 1 Queues
- Note Part 1 is a separate deliverable:
Write a class with a main method that uses a priority queue to store a list of prioritized chores. This
is all the information that is provided. The rest is up to you. You may decide how to store your
chores and how to store your priorities.
YOU MUST WRITE your own Queue class – do not use the Java Library, Queue Class.
Chapter 17 Solutions
Problem Solving with C++ (9th Edition)
Ch. 17.1 - Write a function template named maximum. The...Ch. 17.1 - Prob. 2STECh. 17.1 - Define or characterize the template facility for...Ch. 17.1 - Prob. 4STECh. 17.1 - Display 7.10 shows a function called search, which...Ch. 17.1 - Prob. 6STECh. 17.2 - Give the definition for the member function...Ch. 17.2 - Give the definition for the constructor with zero...Ch. 17.2 - Give the definition of a template class called...Ch. 17.2 - Is the following true or false? Friends are used...
Ch. 17 - Write a function template for a function that has...Ch. 17 - Prob. 2PCh. 17 - Prob. 3PCh. 17 - Redo Programming Project 3 in Chapter 7, but this...Ch. 17 - Display 17.3 gives a template function for sorting...Ch. 17 - (This project requires that you know what a stack...Ch. 17 - Prob. 6PPCh. 17 - Prob. 7PPCh. 17 - This project requires that you complete...
Knowledge Booster
Similar questions
- You need to implement a class for Dequeue i.e. for double ended queue. In this queue, elements can be inserted and deleted from both the ends. You don't need to double the capacity. You need to implement the following functions - 1. constructor You need to create the appropriate constructor. Size for the queue passed is 10. 2. insertFront - This function takes an element as input and insert the element at the front of queue. Insert the element only if queue is not full. And if queue is full, print -1 and return. 3. insertRear - This function takes an element as input and insert the element at the end of queue. Insert the element only if queue is not full. And if queue is full, print -1 and return. 4. deleteFront - This function removes an element from the front of queue. Print -1 if queue is empty. 5. deleteRear - This function removes an element from the end of queue. Print -1 if queue is empty. 6. getFront - Returns the element…arrow_forwardQuestion 11 Write a method called changeQueue to be considered inside the ArrayQueue class and has one parameter item of type E. Your method will change the queue in such a way that if the the first element of the queue and the last element of the queue are equal, the first and last elements in the queue will be replaced with item, otherwise, do not do any change. Similarly, the same process is done for the second element in the queue and the element before the last in the queue and so on. Assume you have a non-empty queue with even number of elements. You are not allowed to call any method from the the ArrayQueue class. Do not use iterators. Method head: public void changeQueue(E item) Example1: Before run: front rear "this" queue: 10 29 2 25 10 Item1: 100 After run: front rear "this" queue: 100 29 100 100 5 100 Use the editor to format your answerarrow_forwardProblem Description: 1. Suppose that we want to add a method to a class of queues that will splice two queues together. This method adds to the end of a queue all items that are in a second queue. The header of the method could be as follows: public void splice (QueueInterface anotherQueue) Write this method in such a way that it will work in any class that implements QueueInterface.arrow_forward
- In this task, a skip list data structure should be implemented. You can follow the followinginstructions:- Implement the class NodeSkipList with two components, namely key node and arrayof successors. You can also add a constructor, but you do not need to add anymethod.- In the class SkipList implement a constructor SkipList(long maxNodes). The parameter maxNodes determines the maximal number of nodes that can be added to askip list. Using this parameter we can determine the maximal height of a node, thatis, the maximal length of the array of successors. As the maximal height of a nodeit is usually taken the logarithm of the parameter. Further, it is useful to constructboth sentinels of maximal height.- In the class SkipList it is useful to write a method which simulates coin flip andreturns the number of all tosses until the first head comes up. This number representsthe height of a node to be inserted.- In the class SkipList implement the following methods:(i) insert, which accepts…arrow_forwardA readinglist is a doubly linked list in which each element of the list is a book. So, you must make sure that Books are linked with the previous prev and next element. A readinglist is unsorted by default or sorted (according to title) in different context. Please pay attention to the task description below. Refer to the relevance classes for more detail information. Implement the add_book_sorted method of the ReadingList class. Assume the readinglist is sorted by title, the add_book_sorted method takes an argument new_book (a book object), it adds the new_book to the readinglist such that the readinglist remain sorted by title. For example, if the readinglist contain the following 3 books: Title: Artificial Intelligence Applications Author: Cassie Ng Published Year: 2000 Title: Python 3 Author: Jack Chan Published Year: 2016 Title: Zoo Author: Cassie Chun Published Year: 2000 If we add another book (titled "Chinese History"; author "Qin Yuan"; and published year 1989) to the…arrow_forwardComputer Science Write a program in the Java language that includes: 1. A class for books, taking into account the encapsulation and getter, setter. 2. Entering books by the user using Scanner 3. LinkedList class for: (Adding a new book - Inserting a book- Searching for a book using the ISBN Number - Viewing all books,delete book). 4. Serial number increases automatically with each book that is entered. 5. Printing the names of books is as follows: Serial - ISBN - Name - PubYear - Price - Notes. 6-Design a main menu that includes: • Add Book • Insert Book • Delete Book • Search . Display . Exitarrow_forward
- C++ programming design a class to implement a sorted circular linked list. The usual operations on a circular list are:Initialize the list (to an empty state). Determine if the list is empty. Destroy the list. Print the list. Find the length of the list. Search the list for a given item. Insert an item in the list. Delete an item from the list. Copy the list. Write the definitions of the class circularLinkedList and its member functions (You may assume that the elements of the circular linked list are in ascending order). Also, write a program to test the operations of your circularLinkedList class. NOTE: The nodes for your list can be defined in either a struct or class. Each node shall store int values.arrow_forwardCreate a class “Node” which is a single node of a singly linked list. The node stores an integer datatype. The class has two data members, an integer and pointer to the same Node class.Implement the following methods:1. The default constructor: Set value of the data member to zero2. A parameterized constructor: Node(int a); takes an integer variable and assigns it to the data member of this object. Set next pointer to NULL.3. Node* GetNextPointer() const; Retun next pointer of this Node4. void SetNextPointer(Node *ptr); Set the next pointer of this node to the ptr that is passed to the function.5. void SetData(int a); Set data of this node equal to ‘a’6. int GetData() const; returns the data of this object.The data member are private whereas the methods are public. part 4 5 and 6 In c++.arrow_forwardCreate a class “Node” which is a single node of a singly linked list. The node stores an integer datatype. The class has two data members, an integer and pointer to the same Node class. Implement the following methods:1. The default constructor: Set value of the data member to zero2. A parameterized constructor: Node(int a); takes an integer variable and assigns it to the data member of this object. Set next pointer to NULL.3. Node* GetNextPointer() const; Retun next pointer of this Node4. void SetNextPointer(Node *ptr); Set the next pointer of this node to the ptr that is passed to the function.5. void SetData(int a); Set data of this node equal to ‘a’6. int GetData() const; returns the data of this object.The data member are private whereas the methods are public.arrow_forward
- import java.util.ArrayList; /** * This class describes a user of Twitter. A user has a user ID * (e.g. @testudo), a list of tweets, a count of the number of followers, * and a list of users that this user follows. Unlike P5, * there is no upper limit on the number of tweets or users to follow. * * You may NOT import any library class other than java.util.ArrayList. * You may NOT add any instance variables to keep a count of the number * of tweets or users being followed. You may add instance variables * for other purposes as long as they are private. */public class TwitterUser { private String userID; private int followers; private ArrayList<Tweet> listTweets; private ArrayList<TwitterUser> toFollow; /** * A constructor that takes the user's ID. If the ID is null, or * longer than 32 characters, or it does not start with "@", * throw an IllegalArgumentException. * * All other instance variables should be initialized…arrow_forwardUsing c++ I would like to implement a queue as a class with a linked list. This queue Is used to help the class print job in displaying things like: a confirmation of the job request received and the status ( denied/accepted) along with the details such as a tracking number, position in the queue( if accepted), reason for denial (if denied), etc. These classes will be used in a menu-driven application that has the following options: request a print job ask for the job name; the name may have blank spaces and consist of alphanumeric characters only, must start with a letter display a confirmation of the job request received and the status ( denied/accepted) along with the details such as a tracking number, position in the queue( if accepted), reason for denial (if denied), etc execute a print job (remove from the queue ) display a confirmation along with the tracking number, the name of the print job, and the number of print jobs currently in the queue display number of print…arrow_forwardPart 3: Building a Point of Sales (POS) linked list data structure. In a POS system, a transaction is based on items purchased by the customer. The following is an example of a customer transaction receipt, where the prices shown in the receipt are GST inclusive. Write a linked list classes (one class for Node and another class for List), which store the items in the transaction. Test the classes by printing the items in the linked list and show the total price of the transaction. The following listing is the sample output for your reference: ============================== BC Items Price ============================== 10 Pagoda Gnut 110g 3.49 11 Hup Seng Cream Cracker 4.19 12 Yit Poh 2n1 Kopi-o 7.28 13 Zoelife SN & Seed 5.24 14 Gatsby S/FO Wet&Hard 16.99 15 GB W/G U/Hold 150g 6.49 ============================== Total (GST Incl.) 43.68…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
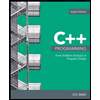
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning