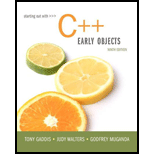
Read , Sort , Merge
Using the ListNode structure of Program 17-2, write the following functions:
ListNode *read()
ListNode *sort(ListNode* list1)
ListNode *merge(ListNode* list1, ListNode* list2)
The first function reads a sequence of whitespace-separated positive numbers and forms the numbers read into a linked list of nodes. The input for a sequence of numbers is terminated by - 1. The second function sorts a linked list of nodes and returns a pointer to the head of the sorted lists. The function should sort by rearranging existing nodes, not by making copies of existing nodes. The third function merges two linked lists that are already sorted into a linked list that is also sorted. The merge function should not make copies of nodes: Rather, it must remove nodes from the two lists and form those nodes into a new list.
Test your functions by having the user enter two sequences of numbers, sorting each sequence, and then merging and printing the resulting list.

Want to see the full answer?
Check out a sample textbook solution
Chapter 17 Solutions
Starting Out with C++: Early Objects (9th Edition)
Additional Engineering Textbook Solutions
C Programming Language
Starting Out with Python (4th Edition)
Web Development and Design Foundations with HTML5 (8th Edition)
Starting Out with Java: From Control Structures through Data Structures (3rd Edition)
Starting Out With Visual Basic (7th Edition)
- Old MathJax webview Old MathJax webview In Java Some methods of the singly linked list listed below can be implemented efficiently (in different respects) (as opposed to an array or a doubly linked list), others not necessarily which are they and why? b. Implement a function to add an element before the first element. c. Implement a function to add an item after the last one element. d. Implement a function to output an element of the list. e. Implement a function to output the entire list. f. Implement a function to output the number of elements. G. Implement a function to delete an item. H. Implement a function to clear the entire list. I. Implement functionality to search for one or more students by first name, last name, matriculation number or course of study. J. Implement functionality to sort the records of the student, matriculation number and course according to two self-selected sorting methods.arrow_forward#### Part 1 Write a Python function (`binary_search`) that implements the binary search algorithm. This function will take a list argument (`values`), a value to search for (`to_find`), a start index (`start_index`), and an end index (`end_index`). The function will return `True` if `to_find` is present in `values`, and `False` otherwise. Below, you will find some code to test your binary search: ```python values = [2,4,6,8,10,12,14,16,18,20] print(binary_search(values, 14, 0, len(values) - 1)) print(binary_search(values, 7, 0, len(values) - 1)) ``` The output of the test code is below: ``` True False ``` #### Part 2 Modify your `binary_search` function to count the number of comparisons (==, <, <=, >, or >=). The function will now return both the number of comparisons made and the `True` or `False` result. The code below calls your function, and creates a simple ASCII bar chart of the number of comparisons (divided by 10, to account for small differences). Some…arrow_forwardUsing the ListNode structure introduced in this chapter, write a function void printFirst(ListNode *ptr)that prints the value stored in the first node of a list passed to it as parameter. The function should print an error message and terminate the program if the list passed to it is empty.arrow_forward
- 16-Write a function called find_integer_with_most_divisors that receives a list of integers and returns the integer from the list with the most divisors .In the event of a tie, the first case with the most divisors returns .For example: If the list looks like this: [8, 12, 18, 6] In this list, the number 8 has four divisors, which are ;[1,2,4,8] :The number 12 has six divisors, which are ;[1,2,3,4,6,12] :The number 18 has six divisors, which are ;[1,2,3,6,9,18] :And the number 6 has four divisors, which are : [1,2,3,6]Note that both items 12 and 18 have the maximum number of divisors and are equal in number of divisors (both have 6 divisors) .Your function must return the first item with the maximum number of divisors ;Therefore it must return the following value: 12arrow_forwardWrite the code to create a linked list using c++ Include the following functionality: Insert at the head or tail of the list. Delete one item (based on an entered value) or all of the list. Print out the linked list. Use the following class and struct to create your linked list: #include <iostream> #include <algorithm> using std::cout; using std::endl; using std::swap; struct Node { int m_data; Node* m_next; }; class LList { public: LList(); LList(int data); LList(const LList& copy); LList(LList&& move) noexcept; ~LList(); int getData(); Node* getNext(); void setData(int data); void insertHead(int data); void insertTail(int data); void deleteNode(int data); void setNext(LList* next); LList& operator = (const LList& copy); LList& operator = (LList&& move) noexcept; void printList(); private: Node* head; };arrow_forwardIf you have the following node declaration:struct Node {int number;struct Node * next;};typedef struct Node node;node *head,*newNode;Write a C program that contains the following functions to manipulate this linked list : 3. A function deletes the element in the middle of the list (free this memory location) (if the list has 100 or 101 elements, it will delete the 50th element). The function will take a list as a parameter and return the updated list. 4. 2nd function named changeFirstAndLast that swaps the node at the end of the list and the node at the beginning of the list. The function will take a list as a parameter and return the updated list. 5. 3rd function using given prototype below. This function cuts the first node of the list and adds it to the end as last node. It takes beginning address of the list as a parameter and returns the updated list.node* cutheadaddlast(node* head);arrow_forward
- Write the following function that merges two sorted lists into a new sorted list:def merge(list1, list2): Implement the function in a way that takes len(list1) + len(list2) comparisons. Write a test program that prompts the user to enter two sorted lists and displays the merged list.arrow_forwardC++ Programing D.S.Malik 16-13: This chapter defined and identified various operations on a circular linked list. Use the definitions of the class circularLinkedList and its member functions. (You may assume that the elements of the circular linked list are in ascending order.) In the main.cpp file, write a program to test various operations of the class defined in the step above. Your program should accept a sorted list as input and output the following: The length of the list. The list after deleting a number. A message indicating if a number is contained in the list Example of Program:arrow_forwardscheme: Q9: Sub All Write sub-all, which takes a list s, a list of old words, and a list of new words; the last two lists must be the same length. It returns a list with the elements of s, but with each word that occurs in the second argument replaced by the corresponding word of the third argument. You may use substitute in your solution. Assume that olds and news have no elements in common. (define (sub-all s olds news) 'YOUR-CODE-HERE )arrow_forward
- Q1:Given the following linked list : Write a function to do each of the following ,then test the functions in the main Program . head 25 30 45 65 60 ptrl a. Insert node(15) to front of node(25). b. Insert node(50) between node(45) and node(65). (using ptrl) c. Delete node( 60).arrow_forwarda) Write a function to get the value of the Nu node in a Linked List. [Note: The first (N=1) item in the list means the item at index 0.] It takes two parameters: the list or its head, and N. Return False if the list has fewer than N elements. The Linked List structure supports the following function. def getlead(self): return selt.head # it points to a Node structure The Node structure supports the following functions. def getData(self): return self.data # it returns the value stored in the Node def getNext(self): return self next # it points to the next Node b) Write a function that counts the number of times a given integer occurs in a Linked List. Assume similar structures as defined in 1.arrow_forwardb. Using user-defined function, create a C++ or Java application implementing a linked list datastructure. The application should be able to perform the following operations on the datastructure can with the following operations.i. Insertion into the list ii. Deletion from the list iii. Printing the content of the listarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
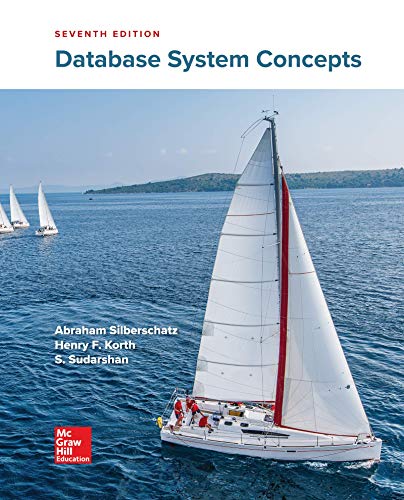
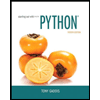
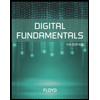
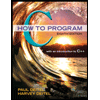
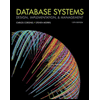
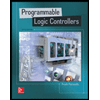