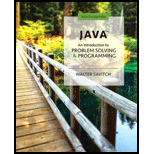
Explanation of Solution
Creating “CharacterFrequency.java”:
- Import required package.
- Define “CharacterFrequency” class.
- Define main function.
- Create array for digit count using “ArrayList”.
- Initialize the digit counts.
- Create object for scanner class.
- Prompt statement for telephone number.
- Read the telephone number from user.
- Compute the count of each character in the telephone number using “for” loop.
- Define each character in telephone number.
- Performs “switch” case.
- If the digit character is “0”, then increment the count of character “0” using “set” and “get” method
- If the digit character is “1”, then increment the count of character “1”.
- If the digit character is “2”, then increment the count of character “2”.
- If the digit character is “3”, then increment the count of character “3”.
- If the digit character is “4”, then increment the count of character “4”.
- If the digit character is “5”, then increment the count of character “5”.
- If the digit character is “6”, then increment the count of character “6”.
- If the digit character is “7”, then increment the count of character “7”.
- If the digit character is “8”, then increment the count of character “8”.
- If the digit character is “9”, then increment the count of character “9”.
- Prompt statement for count of each digit.
- Display the count of each digit using “for” loop.
- Display the count by using “get” method.
- Display count of each digit.
- Define main function.
Program:
The below java program is used to compute the count of each digit in a telephone number using “ArrayList”.
“CharacterFrequency.java”
//Import required package
import java.util.*;
//Define "CharacterFrequency" class
public class CharacterFrequency
{
//Define main function
public static void main(String[] args)
{
/* Create array for digit count using "ArrayList" */
ArrayList<Integer> digit_count = new ArrayList<Integer>();
//Initialize the digit counts
for(int i = 0; i < 10; i++)
{
digit_count.add(0);
}
//Create object for scanner class
Scanner reader = new Scanner(System.in);
//Prompt statement for user
System.out.print("Enter a telephone number: ");
//Read the telephone number from user
String telephoneNumber = reader.next();
/* Compute the count of each character in the telephone number */
for(int i = 0; i < telephoneNumber.length(); i++)
{
/* Define each character in telephone number */
Character digit = telephoneNumber.charAt(i);
//Performs "switch" case
switch(digit)
{
/* If the digit character is "0", then */
case '0':
/* Increment the count of character '0' by using "set" and "get" method */
digit_count.set(0, digit_count.get(0)+1);
break;
/* If the digit character is "1", then */
case '1':
/* Increment the count of character '1' */
digit_count...

Want to see the full answer?
Check out a sample textbook solution
Chapter 12 Solutions
Java: An Introduction to Problem Solving and Programming (8th Edition)
- Compare and contrast the array and arrayList. Give at least one example that describe when to use arrayList compared to an array.arrow_forwardUse the ArrayList class Add and remove objects from an ArrayList Protect from index errors when removing Practice with input loop Details: This homework is for you to get practice adding and removing objects from an ArrayList. The Voter class was used to create instances of Voters which held their name and a voter identification number as instance variables, and the number of instances created as a static variable. This was the class diagram: The constructor takes a string, passed to the parameter n, which is the name of the voter and which should be assigned to the name instance variable. Every time a new voter is created, the static variable nVoters should be incremented. Also, every time a new voter is created, a new voterID should be constructed by concatenating the string “HI” with the value of nVoters and the length of the name. For example, if the second voter is named “Clark Kent”, then the voterID should be “HI210” because 2 is the value of nVoters and 10 is the number…arrow_forwardjava Write a program to do the following: Create an ArrayList of Student. Add 7 elements to the ArrayList. Use for each loop to print all the elements of an ArrayList. Remove an element from an ArrayList using the index number. Remove the element from an ArrayList using the content (element name).arrow_forward
- Write Java code for the following: Create an Arraylist that can hold Integer objects. Check and display whether the Arraylist is empty or not. Add an element 50 into the Arraylist. Add another element 60 in the position of 0 in the Arraylist. Replace the element to 70 in index 1.arrow_forward/** * The constructor has been partially implemented for you. cards is the * ArrayList where you'll be adding all the cards you're given. In addition, * there are two arrays. You don't necessarily need to use them, but using them * will be extremely helpful. * * The rankCounts array is of the same length as the number of Ranks. At * position i of the array, keep a count of the number of cards whose * rank.ordinal() equals i. Repeat the same with Suits for suitCounts. For * example, if your Cards are (Clubs 4, Clubs 10, Spades 2), your suitCounts * array would be {2, 0, 0, 1}. * * @param cards * the list of cards to be added */ public PokerAnalysis(List<Card> cards) { this.cards = new ArrayList<Card>(); this.rankCounts = new int[Rank.values().length]; this.suitCounts = new int[Suit.values().length]; throw new UnsupportedOperationException(); }arrow_forwardWrite Java code for the following: a. Create an Arraylist that can hold Integer objects. b. Check and display whether the Arraylist is empty or not. C. Add an element 50 into the Arraylist. d. Add another element 60 in the position of 0 in the Arraylist. e. Replace the element to 70 in index 1.arrow_forward
- Write a class Employee with name and salary. Create an ArrayList that sorts the Employees based on salary.arrow_forwardThe implementation of a queue in an array, as given in this chapter, uses the variable count to determine whether the queue is empty or full. You can also use the variable count to return the number of elements in the queue. On the other hand, class linkedQueueType does not use such a variable to keep track of the number of elements in the queue. Redefine the class linkedQueueType by adding the variable count to keep track of the number of elements in the queue. Modify the definitions of the functions addQueue and deleteQueue as necessary. Add the function queueCount to return the number of elements in the queue. Also, write a program to test various operations of the class you defined.arrow_forwardPlease keep the simulation neat and well organized. It is a request. Perform QuickSort on the given array. You need to show the whole simulation. A = [10,3,9,18,21,26,30,8,15,6,17].arrow_forward
- Please keep the simulation neat and well organized. It is a request. NOTE: I AM ASKING FOR SIMULATION, NOT THE CODE. SO DON'T WRITE THE CODE. Perform QuickSort on the given array. You need to show the whole simulation. A = [10,3,9,18,21,26,30,8,15,6,17].arrow_forwardThe function remove of the class arrayList removes only the first occurrence of an element. Add the function removeAll to the class arrayList that would remove all occurrences of a given element. Also, write the definition of the function removeAll and a program to test this functionarrow_forwardplease use the name, phonenumber and phonebookentry to build this. The three parallel arrays are gone — replaced with a single array of type PhonebookEntry. You should be reading in the entries using the read method of your PhonebookEntry class (which in turn uses the read methods of the Name and PhoneNumber classes). Use the equals methods of the Name and PhoneNumber classes in your lookup and reverseLookup methods. Use the toString methods to print out information. Make 100 the capacity of your Phonebook array Throw an exception (of class Exception) if the capacity of the Phonebook array is exceeded. Place a try/catch around your entire main and catch both FileNotFoundExceptions and Exceptions (remember, the order of appearance of the exception types in the catch blocks can make a difference). Do not use BufferedReader while(true) breaks The name of your application class should be Phonebook. Also, you should submit ALL your classes (i.e., Name, Strip off public from all your class…arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
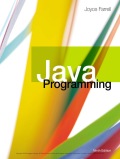
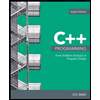