Write a Java program that implements both Linear Search and Binary Search. The program will take a collection of objects (generic type data) as input and print the number of comparisons needed to find a target element within that collection. You will create the following two Java classes: 1. SearchCombo.java : Code for both linearSearch and binarySearch will be in this class. You may take help from the textbook Chapter 9, Section 9.1. However, note that the design require- ments are different from the textbook code. •Both search methods must use the Comparable interface and the compareTo() method. •Your program must be able to handle different data types, i.e., use generics. •For binarySearch, if you decide to use a midpoint computation formula that is different from the textbook, explain that formula briefly as a comment within your code. 2. Tester.java : This class will contain the main() method. The user will be asked to enter the collection of elements (comma or space can be used to separate the elements). The output will be the number of comparisons the two searching techniques require. Review the sample output given below to get an idea of what the program output should look like.
Write a Java program that implements both Linear Search and Binary Search. The program will
take a collection of objects (generic type data) as input and print the number of comparisons needed
to find a target element within that collection.
You will create the following two Java classes:
1. SearchCombo.java : Code for both linearSearch and binarySearch will be in this class. You
may take help from the textbook Chapter 9, Section 9.1. However, note that the design require-
ments are different from the textbook code.
•Both search methods must use the Comparable<T> interface and the compareTo() method.
•Your program must be able to handle different data types, i.e., use generics.
•For binarySearch, if you decide to use a midpoint computation formula that is different from
the textbook, explain that formula briefly as a comment within your code.
2. Tester.java : This class will contain the main() method. The user will be asked to enter the
collection of elements (comma or space can be used to separate the elements). The output will
be the number of comparisons the two searching techniques require.
Review the sample output given below to get an idea of what the program output should look like.


Trending now
This is a popular solution!
Step by step
Solved in 2 steps

take a collection of objects (generic type data) as input and print the number of comparisons needed
to find a target element within that collection.
You will create the following two Java classes:
1. SearchCombo.java : Code for both linearSearch and binarySearch will be in this class. You
may take help from the textbook Chapter 9, Section 9.1. However, note that the design require-
ments are different from the textbook code.
•Both search methods must use the Comparable<T> interface and the compareTo() method.
•Your program must be able to handle different data types, i.e., use generics.
•For binarySearch, if you decide to use a midpoint computation formula that is different from
the textbook, explain that formula briefly as a comment within your code.
collection of elements (comma or space can be used to separate the elements). The output will
be the number of comparisons the two searching techniques require.
Review the sample output given below to get an idea of what the program output should look like.
Your output may include additional information.
public static <T>
boolean linearSearch(T[] data, int min, int max, T target) {
int index = min;
boolean found = false;
while (!found && index <= max) {
found = data [index].equals(target);
index++;
}
return found;
}
public static <T extends Comparable<T>>
boolean binarySearch(T[] data, int min, int max, T target) {
boolean found = false;
int midpoint = (min + max)/2;
if (data[midpoint].compareTo(target)==0)
found = true;
else if(data[midpoint].compareTo(target)>0) {
if(min<=midpoint-1)
found = binarySearch(data, min, midpoint-1, target);
}
else if (midpoint+1<=max)
found=binarySearch(data, midpoint+1, max, target);
return found;
}
}
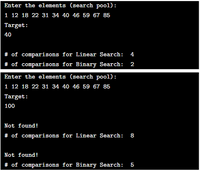
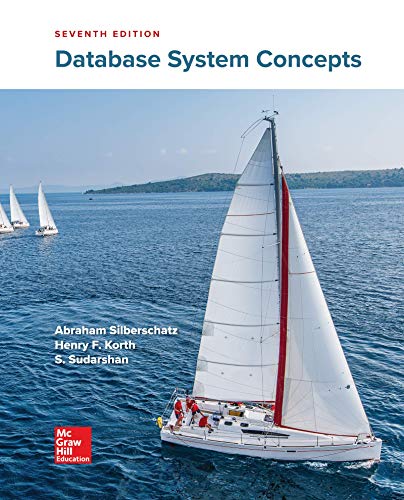
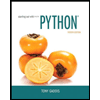
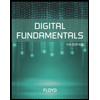
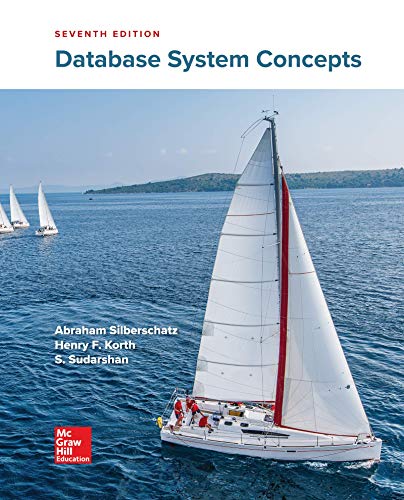
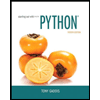
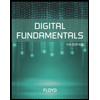
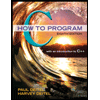
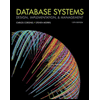
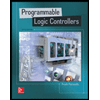