Using client-server socket programming, implement a multi-threaded server that returns to the client the synonym of a word as stored in a dictionary. The client should prompt the user for a word, reads the word from the screen and sends it to the server and waits for response from the server. Once the client gets the synonym of the word, it should print the word and its synonym on the screen and prompt for another word.
Using client-server socket programming, implement a multi-threaded server that
returns to the client the synonym of a word as stored in a dictionary. The client
should prompt the user for a word, reads the word from the screen and sends it to
the server and waits for response from the server. Once the client gets the synonym
of the word, it should print the word and its synonym on the screen and prompt for
another word. This should continue until the user enters the characters @ to quit.
Example:
>>> python TCPClient.py
Input a word: weak
weak ==> frail
Input a word: fair
fair ==> just
Input a word: @
Notes:
1) A file that contains some words and their synonyms is provided
2) In the server code, read the file to a global python dictionary object
engDict = {} # empty dictionary object
with open('dictionary.txt') as f: # open the file
for line in f:
tok = line.split()
engDict[tok[0]] = tok[1]
print(engDict)
3) Here is a Skelton of the server
[some code here ]
class ServeClient(threading.Thread):
def __init__(self, conn, (ip, port)):
threading.Thread.__init__(self)
self.ip = ip
[add code here ]
def run (self) :
while True:
data =
[ add code here ]
if cmd == '@':
[add code here ]
if engDict.has_key(cmd):
[add code here ]
else:
[add code here ]
[add code here ]
with open('dictionary.txt') as f:
[add code here ]
serverName = socket.gethostname()
serverPort = 12345
serverSocket = [add code here]
serverSocket.setsockopt(socket.SOL_SOCKET, socket.SO_REUSEADDR, 1)
[add code here ]
print 'Server ready to recieve '
threads = []
while True:
[ add code here ]
newThread = ServeClient(connectionSocket, addr)
[ add code here ]
for t in threads:
t.join()
What to turn in
Turn in by the due date the two files client.py and server.py
![Using client-server socket programming, implement a multi-threaded server that
returns to the client the synonym of a word as stored in a dictionary. The client
should prompt the user for a word, reads the word from the screen and sends it to
the server and waits for response from the server. Once the client gets the synonym
of the word, it should print the word and its synonym on the screen and prompt for
another word. This should continue until the user enters the characters @ to quit.
Example:
> python TCPClient.py
Input a word: weak
weak => frail
Input a word: fair
fair => just
Input a word: @
Notes:
1) A file that contains some words and their synonyms is provided
2) In the server code, read the file to a global python dictionary object
engDict = {} # empty dictionary object
with open('dictionary.txt') as f:
for line in f:
tok = line.split()
engDict[tok[@]] = tok(1]
print(engDict)
# open the file
3) Here is a Skelton of the server
[some code here )
class Serveclient(threading. Thread):
def _init_(self, conn, (ip, port)):
threading. Thread._init_(self)
self. ip - ip
[add code here ]
def run (self) :
while True:
data =
[ add code here ]](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F1e0b9a48-3273-4b57-9b99-1c7a735641e2%2F5c4839d8-488b-4640-b07a-88b748c8d3a0%2F5bbuhkd_processed.png&w=3840&q=75)
![if cmd == 'e':
[add code here )
if engDict.has_key(cmd):
[add code here )
else:
[add code here
[add code here )
with open('dictionary.txt') as f:
fadd code here 1
serverName = socket. gethostname ()
serverPort = 12345
serverSocket = [add code here
serverSocket.setsockopt (socket.SOL_SOCKET, socket.SO_REUSEADDR, 1)
[add code here )
print 'Server ready to recieve
threads - [)
while True:
[ add code here )
newThread = Serveclient(connectionSocket, addr)
[ add code here ]
for t in threads:
t.join()
What to turn in
Turn in by the due date the two files client.py and server.py](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F1e0b9a48-3273-4b57-9b99-1c7a735641e2%2F5c4839d8-488b-4640-b07a-88b748c8d3a0%2F7gmnsnt_processed.png&w=3840&q=75)

Step by step
Solved in 3 steps with 3 images

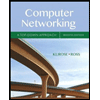
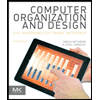
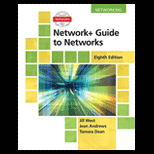
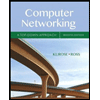
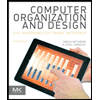
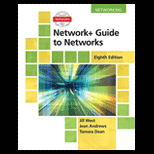
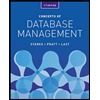
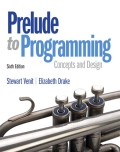
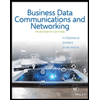