Please help convert the following Java coding to C++ /LinkedList.java============== //Interface public interface LinkedList { public boolean isEmpty(); public void insertAtStart(T data); public void insertAtEnd(T data); public void deleteAtStart(); public void deleteAtEnd(); public T getStartData(); public T getEndData(); public void deleteLinkedList(); } //===end of LinkedList.java===== //==LinkNode.java================ public class LinkNode { private T data; private LinkNode next; public LinkNode(T data,LinkNode next) { this.setData(data); this.setNext(next); } public T getData() { return data; } public void setData(T data) { this.data = data; } public LinkNode getNext() { return next; } public void setNext(LinkNode next) { this.next = next; } } //==end of LinkNode.java========= //===singlyLinkedList.java============= public class SinglyLinkedList implements LinkedList{ private int count; private LinkNode start; private LinkNode end; public SinglyLinkedList(){ start=null; end=null; setCount(0); } public void createSinglyLinkedList() { start=null; end=null; setCount(0); } public boolean isEmpty() { return start==null; } public int getCount() { return count; } public void setCount(int count) { this.count = count; } public void insertAtStart(T data) { LinkNode node=new LinkNode(data,null); count++; if(isEmpty()){ start=node; end=node; return; } node.setNext(start); start=node; } public void insertAtEnd(T data) { if(isEmpty()) { insertAtStart(data); return; } LinkNode node=new LinkNode(data,null); count++; end.setNext(node); end=node; } public void deleteAtStart() { //list is empty if(isEmpty()) return; //only one element in list if(count==1) { start=end=null; count=0; return; } start=start.getNext(); count--; } public void deleteAtEnd() { //list is empty if(isEmpty()) return; //only one element in list if(count==1) { start=end=null; count=0; return; } LinkNode tmp=start; while(tmp.getNext().getNext()!=null) { tmp=tmp.getNext(); } tmp.setNext(null); end=tmp; count--; } public String toString() { String str="["; LinkNode tmp=start; while(tmp!=null) { str+=tmp.getData()+" "; tmp=tmp.getNext(); } str+="]"; return str; } public T getStartData() { T value = null; try { value=start.getData(); }catch(Exception e) { System.out.println("Empty"); } return value; } public T getEndData() { T value = null; try { value=end.getData(); }catch(Exception e) { System.out.println("Empty"); } return value; } public void deleteLinkedList() { start=end=null; count=0; } } //==end of singlyLinkedList.java=======
Please help convert the following Java coding to C++
/LinkedList.java==============
//Interface
public interface LinkedList<T> {
public boolean isEmpty();
public void insertAtStart(T data);
public void insertAtEnd(T data);
public void deleteAtStart();
public void deleteAtEnd();
public T getStartData();
public T getEndData();
public void deleteLinkedList();
}
//===end of LinkedList.java=====
//==LinkNode.java================
public class LinkNode<T> {
private T data;
private LinkNode<T> next;
public LinkNode(T data,LinkNode<T> next) {
this.setData(data);
this.setNext(next);
}
public T getData() {
return data;
}
public void setData(T data) {
this.data = data;
}
public LinkNode<T> getNext() {
return next;
}
public void setNext(LinkNode<T> next) {
this.next = next;
}
}
//==end of LinkNode.java=========
//===singlyLinkedList.java=============
public class SinglyLinkedList<T> implements LinkedList<T>{
private int count;
private LinkNode<T> start;
private LinkNode<T> end;
public SinglyLinkedList(){
start=null;
end=null;
setCount(0);
}
public void createSinglyLinkedList() {
start=null;
end=null;
setCount(0);
}
public boolean isEmpty() {
return start==null;
}
public int getCount() {
return count;
}
public void setCount(int count) {
this.count = count;
}
public void insertAtStart(T data) {
LinkNode<T> node=new LinkNode<T>(data,null);
count++;
if(isEmpty()){
start=node;
end=node;
return;
}
node.setNext(start);
start=node;
}
public void insertAtEnd(T data) {
if(isEmpty()) {
insertAtStart(data);
return;
}
LinkNode<T> node=new LinkNode<T>(data,null);
count++;
end.setNext(node);
end=node;
}
public void deleteAtStart() {
//list is empty
if(isEmpty())
return;
//only one element in list
if(count==1) {
start=end=null;
count=0;
return;
}
start=start.getNext();
count--;
}
public void deleteAtEnd() {
//list is empty
if(isEmpty())
return;
//only one element in list
if(count==1) {
start=end=null;
count=0;
return;
}
LinkNode<T> tmp=start;
while(tmp.getNext().getNext()!=null) {
tmp=tmp.getNext();
}
tmp.setNext(null);
end=tmp;
count--;
}
public String toString() {
String str="[";
LinkNode<T> tmp=start;
while(tmp!=null) {
str+=tmp.getData()+" ";
tmp=tmp.getNext();
}
str+="]";
return str;
}
public T getStartData() {
T value = null;
try {
value=start.getData();
}catch(Exception e) {
System.out.println("Empty");
}
return value;
}
public T getEndData() {
T value = null;
try {
value=end.getData();
}catch(Exception e) {
System.out.println("Empty");
}
return value;
}
public void deleteLinkedList() {
start=end=null;
count=0;
}
}
//==end of singlyLinkedList.java=======

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 5 images

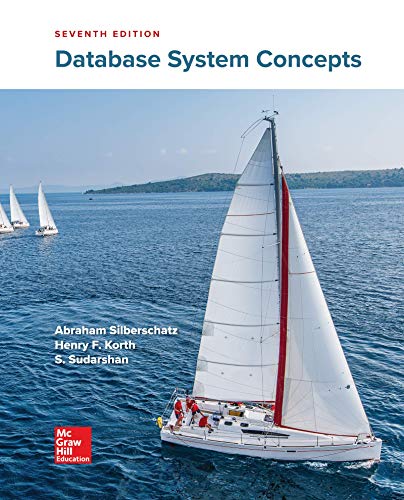
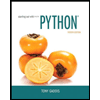
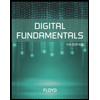
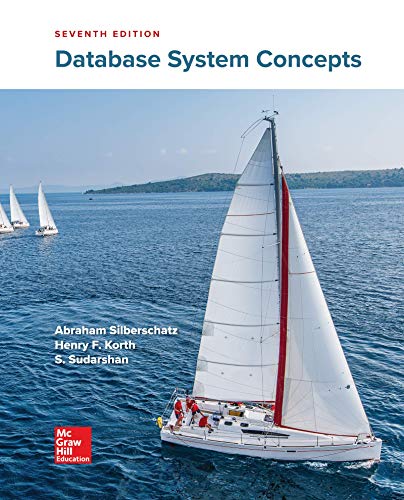
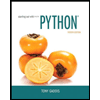
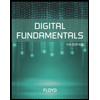
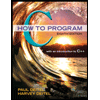
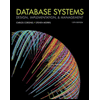
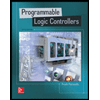