An airport has a runway for airplanes landing and taking off. When the runway is busy, airplanes wishing to take off or land have to wait. Landing airplanes get priority, and if the runway is available, it can be used. Implement a Java class, Airport.java, for this simulation, using two appropriate lists, one for the airplanes waiting to take off and one for those waiting to land. Note that the data structures you select for the two lists must be suitable for this purpose. For instance, the sooner an airplane comes for landing, the sooner it will land. Also, you must keep the record of all the airplanes that have already landed or taken off in one single list, to print out the activity log whenever asked, such that the sooner an airplane landed, the later it shows in the printout. To get a clear idea, please have a close look at the expected outputs of the execution of the tester class provided. The user enters the following commands: (The user entry has already been done in the tester class) • t flight-number (for taking off the airplane with the flight number flight-number) • l flight-number (for landing the airplane with the flight number flight-number) • n (for conducting the next possible landing or taking off operation) • p (for printing the current status of the two queues) • g (for printing the list of the airplanes already taken off or landed) • q (to quit the program) The first two commands, t and l, place the indicated flight in the take-off or landing queue, respectively. Command n will conduct the current take-off or landing, print the action (take-off or land) and the flightnumber, and enable the next one. Command p will print out the current content of the two queues. Command g will print out the list of all the airplanes that have already taken off or landed. Command q will quit the program. Provide required instance variables. Provide the following methods in this class: • The Airport class must have one default constructor to initialize the instance variables. • addTakeOff: It receives a flight-number as a String and updates the corresponding list. • addLanding: It receives a flight-number as a String and updates the corresponding list. • handleNextAction: It checks the landing and take-off lists and conducts one single operation as described above. It also returns a String showing the conducting operation, as you can see in the expected output. • waitingPlanes: It returns a String showing the list of all the waiting airplanes for landing/take-off, as you can see in the expected output. • log: It returns a String showing the list of all the operations already conducted, as you can see in the expected output. Note: The sooner an airplane landed/taken off, the later it must appear in the log. • Overloaded log: It receives the name of a text file as a String and writes the same output of the above log function into the text file instead of showing it on the screen. Note: The sooner an airplane lands/is taken off, the later it must appear in the log. The tester class, AirportTester.java, has been provided to help you with the development as well as executing and comparing the expected and actual outputs. The expected outputs of the AirportTester class using your code for Airport.java should be similar to the lines on the next page. Texts with green color are the user inputs. IN JAVA
An airport has a runway for airplanes landing and taking off. When the runway is busy, airplanes wishing to take off or land have to wait. Landing airplanes get priority, and if the runway is available, it can be used. Implement a Java class, Airport.java, for this simulation, using two appropriate lists, one for the airplanes waiting to take off and one for those waiting to land. Note that the data structures you select for the two lists must be suitable for this purpose. For instance, the sooner an airplane comes for landing, the sooner it will land. Also, you must keep the record of all the airplanes that have already landed or taken off in one single list, to print out the activity log whenever asked, such that the sooner an airplane landed, the later it shows in the printout. To get a clear idea, please have a close look at the expected outputs of the execution of the tester class provided. The user enters the following commands: (The user entry has already been done in the tester class) • t flight-number (for taking off the airplane with the flight number flight-number) • l flight-number (for landing the airplane with the flight number flight-number) • n (for conducting the next possible landing or taking off operation) • p (for printing the current status of the two queues) • g (for printing the list of the airplanes already taken off or landed) • q (to quit the program) The first two commands, t and l, place the indicated flight in the take-off or landing queue, respectively. Command n will conduct the current take-off or landing, print the action (take-off or land) and the flightnumber, and enable the next one. Command p will print out the current content of the two queues. Command g will print out the list of all the airplanes that have already taken off or landed. Command q will quit the program. Provide required instance variables. Provide the following methods in this class: • The Airport class must have one default constructor to initialize the instance variables. • addTakeOff: It receives a flight-number as a String and updates the corresponding list. • addLanding: It receives a flight-number as a String and updates the corresponding list. • handleNextAction: It checks the landing and take-off lists and conducts one single operation as described above. It also returns a String showing the conducting operation, as you can see in the expected output. • waitingPlanes: It returns a String showing the list of all the waiting airplanes for landing/take-off, as you can see in the expected output. • log: It returns a String showing the list of all the operations already conducted, as you can see in the expected output. Note: The sooner an airplane landed/taken off, the later it must appear in the log. • Overloaded log: It receives the name of a text file as a String and writes the same output of the above log function into the text file instead of showing it on the screen. Note: The sooner an airplane lands/is taken off, the later it must appear in the log. The tester class, AirportTester.java, has been provided to help you with the development as well as executing and comparing the expected and actual outputs. The expected outputs of the AirportTester class using your code for Airport.java should be similar to the lines on the next page. Texts with green color are the user inputs.
IN JAVA


Step by step
Solved in 2 steps

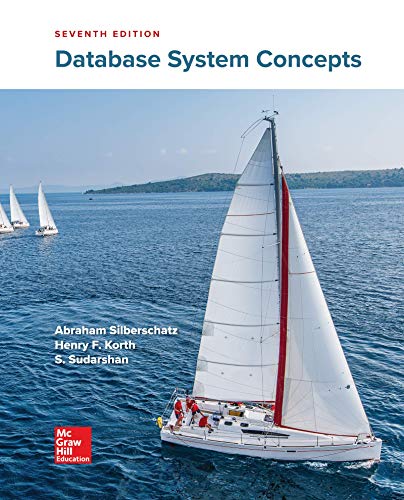
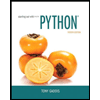
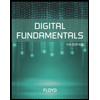
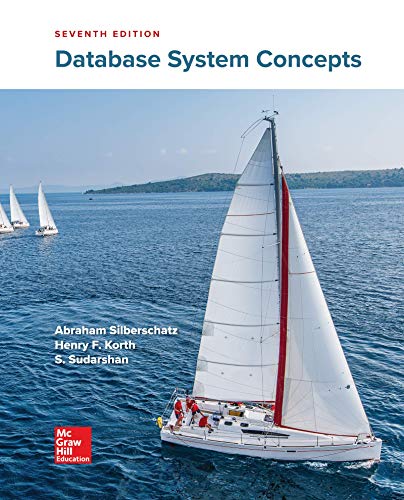
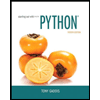
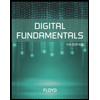
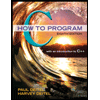
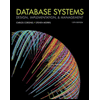
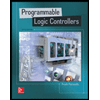