Parser - setup Make sure to make a Parser class (does not derive from anything). It must have a constructor that accepts your collection of Tokens. We will be treating the collection of tokens as a queue - taking off the front. It isn't necessary to use a Java Queue, but you may. We will add three helper functions to parser. These should be private: matchAndRemove - accepts a token type. Looks at the next token in the collection: If the passed in token type matches the next token's type, remove that token and return it. If the passed in token type DOES NOT match the next token's type (or there are no more tokens) return null. expectEndsOfLine - uses matchAndRemove to match and discard one or more ENDOFLINE tokens. Throw a SyntaxErrorException if no ENDOFLINE was found. peek - accepts an integer and looks ahead that many tokens and returns that token. Returns null if there aren't enough tokens to fulfill the request. Parser - parse Make sure to make a public parse method. There are no parameters, and it returns Node. This will be called from main once lexing is complete. For now, we are going to only parse a subset of Shank V2 - mathematical expressions. Parse should call expression() then expectEndOfLine() in a loop until either returns null. Don't worry about storing the return node but you should print it out (using ToString()) for testing. Below is the parser file. There are errors in the parser.java code. Please fix those errors in the parser.java and make sure the parser works correctly. Attached is the rubric. package mypack; import java.util.List; import mypack.Token.TokenType; public class Parser { private List tokens; private int currentTokenIndex; public Parser(List tokens) { this.tokens = tokens; this.currentTokenIndex = 0; } public Node parse() throws SyntaxErrorException { Node result = null; while (peek() != null) { Node expr = expression(); expectEndOfLine(); System.out.println(expr.toString()); } return result; } private Node expression() { Node result = term(); while (peek() != null && (peek().getType() == TokenType.PLUS || peek().getType() == TokenType.MINUS)) { Token token = matchAndRemove(peek().getType()); Node right = term(); if (right == null) { throw new SyntaxErrorException("Expected right operand after " + token.getValue()); } result = new MathOpNode(token.getType(), result, right); } return result; } private Node term() { Node result = factor(); while (peek() != null && (peek().getType() == TokenType.TIMES || peek().getType() == TokenType.DIVIDE || peek().getType() == TokenType.MOD)) { Token token = matchAndRemove(peek().getType()); Node right = factor(); if (right == null) { throw new SyntaxErrorException("Expected right operand after " + token.getValue()); } result = new MathOpNode(token.getType(), result, right); } return result; } private Node factor() { Token token = matchAndRemove(TokenType.MINUS); Node result = null; if (peek() != null) { if (peek().getType() == TokenType.NUMBER) { Token numberToken = matchAndRemove(TokenType.NUMBER); if (token != null) { result = new IntegerNode(-Integer.parseInt(numberToken.getValue())); } else { result = new IntegerNode(Integer.parseInt(numberToken.getValue())); } } else if (peek().getType() == TokenType.IPAR) { matchAndRemove(TokenType.IPAR); result = expression(); expect(TokenType.RPAR); } } return result; } private Token matchAndRemove(TokenType type) { if (peek() != null && peek().getType() == type) { currentTokenIndex++; return tokens.get(currentTokenIndex - 1); } return null; } private void expect(TokenType type) throws SyntaxErrorException { Token token = matchAndRemove(type); if (token == null) { throw new SyntaxErrorException("Expected " + type.toString()); } } private void expectEndOfLine() throws SyntaxErrorException { expect(TokenType.ENDOFLINE); } private Token peek() { return currentTokenIndex < tokens.size() ? tokens.get(currentTokenIndex) : null; } }
Parser - setup
Make sure to make a Parser class (does not derive from anything). It must have a constructor that accepts your collection of Tokens. We will be treating the collection of tokens as a queue - taking off the front. It isn't necessary to use a Java Queue, but you may.
We will add three helper functions to parser. These should be private:
matchAndRemove - accepts a token type. Looks at the next token in the collection:
If the passed in token type matches the next token's type, remove that token and return it.
If the passed in token type DOES NOT match the next token's type (or there are no more tokens) return null.
expectEndsOfLine - uses matchAndRemove to match and discard one or more ENDOFLINE tokens. Throw a SyntaxErrorException if no ENDOFLINE was found.
peek - accepts an integer and looks ahead that many tokens and returns that token. Returns null if there aren't enough tokens to fulfill the request.
Parser - parse
Make sure to make a public parse method. There are no parameters, and it returns Node. This will be called from main once lexing is complete. For now, we are going to only parse a subset of Shank V2 - mathematical expressions. Parse should call expression() then expectEndOfLine() in a loop until either returns null. Don't worry about storing the return node but you should print it out (using ToString()) for testing.
Below is the parser file. There are errors in the parser.java code. Please fix those errors in the parser.java and make sure the parser works correctly. Attached is the rubric.
package mypack;
import java.util.List;
import mypack.Token.TokenType;
public class Parser {
private List<Token> tokens;
private int currentTokenIndex;
public Parser(List<Token> tokens) {
this.tokens = tokens;
this.currentTokenIndex = 0;
}
public Node parse() throws SyntaxErrorException {
Node result = null;
while (peek() != null) {
Node expr = expression();
expectEndOfLine();
System.out.println(expr.toString());
}
return result;
}
private Node expression() {
Node result = term();
while (peek() != null && (peek().getType() == TokenType.PLUS || peek().getType() == TokenType.MINUS)) {
Token token = matchAndRemove(peek().getType());
Node right = term();
if (right == null) {
throw new SyntaxErrorException("Expected right operand after " + token.getValue());
}
result = new MathOpNode(token.getType(), result, right);
}
return result;
}
private Node term() {
Node result = factor();
while (peek() != null && (peek().getType() == TokenType.TIMES || peek().getType() == TokenType.DIVIDE || peek().getType() == TokenType.MOD)) {
Token token = matchAndRemove(peek().getType());
Node right = factor();
if (right == null) {
throw new SyntaxErrorException("Expected right operand after " + token.getValue());
}
result = new MathOpNode(token.getType(), result, right);
}
return result;
}
private Node factor() {
Token token = matchAndRemove(TokenType.MINUS);
Node result = null;
if (peek() != null) {
if (peek().getType() == TokenType.NUMBER) {
Token numberToken = matchAndRemove(TokenType.NUMBER);
if (token != null) {
result = new IntegerNode(-Integer.parseInt(numberToken.getValue()));
} else {
result = new IntegerNode(Integer.parseInt(numberToken.getValue()));
}
} else if (peek().getType() == TokenType.IPAR) {
matchAndRemove(TokenType.IPAR);
result = expression();
expect(TokenType.RPAR);
}
}
return result;
}
private Token matchAndRemove(TokenType type) {
if (peek() != null && peek().getType() == type) {
currentTokenIndex++;
return tokens.get(currentTokenIndex - 1);
}
return null;
}
private void expect(TokenType type) throws SyntaxErrorException {
Token token = matchAndRemove(type);
if (token == null) {
throw new SyntaxErrorException("Expected " + type.toString());
}
}
private void expectEndOfLine() throws SyntaxErrorException {
expect(TokenType.ENDOFLINE);
}
private Token peek() {
return currentTokenIndex < tokens.size() ? tokens.get(currentTokenIndex) : null;
}
}


The Parser class in Java is an abstract class that provides the basic methods for processing input from a stream of characters. It is used for reading and manipulating text in applications. The Parser class provides methods for parsing data from a stream of characters, and for transforming that data into objects. It can be used to parse text from XML documents and from HTML documents, as well as from other sources. The most commonly used implementation of the Parser class is the org.xml.sax.Parser class, which is used to parse data from an XML document.
Trending now
This is a popular solution!
Step by step
Solved in 3 steps

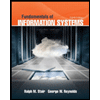
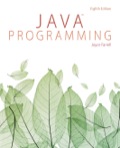
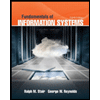
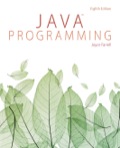