Need assistance running this code. Please include output. I am given an error. Python language # This program allows the user to see the time zone # for a selected city. import tkinter class TimeZone: def __init__(self): # Create the main window. self.main_window = tkinter.Tk() self.main_window.title('Time Zones') # Create the widgets. self._ _build_prompt_label() self._ _build_listbox() self._ _build_output_frame() self._ _build_quit_button() # Start the main loop. tkinter.mainloop() # This method creates the prompt_label widget. def _ _build_prompt_label(self): self.prompt_label = tkinter.Label( self.main_window, text='Select a City') self.prompt_label.pack(padx=5, pady=5) # This method creates and populates the city_listbox widget. def _ _build_listbox(self): # Create a list of city names. self._ _cities = ['Denver', 'Honolulu', 'Minneapolis', 'New York', 'San Francisco'] # Create and pack the Listbox. self.city_listbox = tkinter.Listbox( self.main_window, height=0, width=0) self.city_listbox.pack(padx=5, pady=5) # Bind a callback function to the Listbox. self.city_listbox.bind( '<>', self._ _display_time_zone) # Populate the Listbox. for city in self._ _cities: self.city_listbox.insert(tkinter.END, city) # This method creates the output_frame and its contents. def _ _build_output_frame(self): # Create the frame. self.output_frame = tkinter.Frame(self.main_window) self.output_frame.pack(padx=5) # Create the Label that reads "Time Zone:". self.output_description_label = tkinter.Label( self.output_frame, text='Time Zone:') self.output_description_label.pack( side='left', padx=(5, 1), pady=5) # Create a StringVar variable to hold the time zone name. self._ _timezone = tkinter.StringVar() # Create the Label that displays the time zone name. self.output_label = tkinter.Label( self.output_frame, borderwidth=1, relief='solid', width=15, textvariable=self._ _timezone) self.output_label.pack(side='right', padx=(1, 5), pady=5) # This method creates the Quit button. def _ _build_quit_button(self): self.quit_button = tkinter.Button( self.main_window, text='Quit', command=self.main_window.destroy) self.quit_button.pack(padx=5, pady=5) # Callback function for the city_listbox widget. def _ _display_time_zone(self, event): # Get the current selections. index = self.city_listbox.curselection() # Get the city. city = self.city_listbox.get(index[0]) # Determine the time zone. if city == 'Denver': self._ _timezone.set('Mountain') elif city == 'Honolulu': self._ _timezone.set('Hawaii-Aleutian') elif city == 'Minneapolis': self._ _timezone.set('Central') elif city == 'New York': self._ _timezone.set('Eastern') elif city == 'San Francisco': self._ _timezone.set('Pacific') # Create an instance of the TimeZoneclass if _ _name_ _ == '_ _main_ _': time_zone = TimeZone()
Need assistance running this code. Please include output. I am given an error. Python language
# This program allows the user to see the time zone
# for a selected city.
import tkinter
class TimeZone:
def __init__(self):
# Create the main window.
self.main_window = tkinter.Tk()
self.main_window.title('Time Zones')
# Create the widgets.
self._ _build_prompt_label()
self._ _build_listbox()
self._ _build_output_frame()
self._ _build_quit_button()
# Start the main loop.
tkinter.mainloop()
# This method creates the prompt_label widget.
def _ _build_prompt_label(self):
self.prompt_label = tkinter.Label(
self.main_window, text='Select a City')
self.prompt_label.pack(padx=5, pady=5)
# This method creates and populates the city_listbox widget.
def _ _build_listbox(self):
# Create a list of city names.
self._ _cities = ['Denver', 'Honolulu', 'Minneapolis',
'New York', 'San Francisco']
# Create and pack the Listbox.
self.city_listbox = tkinter.Listbox(
self.main_window, height=0, width=0)
self.city_listbox.pack(padx=5, pady=5)
# Bind a callback function to the Listbox.
self.city_listbox.bind(
'<<ListboxSelect>>', self._ _display_time_zone)
# Populate the Listbox.
for city in self._ _cities:
self.city_listbox.insert(tkinter.END, city)
# This method creates the output_frame and its contents.
def _ _build_output_frame(self):
# Create the frame.
self.output_frame = tkinter.Frame(self.main_window)
self.output_frame.pack(padx=5)
# Create the Label that reads "Time Zone:".
self.output_description_label = tkinter.Label(
self.output_frame, text='Time Zone:')
self.output_description_label.pack(
side='left', padx=(5, 1), pady=5)
# Create a StringVar variable to hold the time zone name.
self._ _timezone = tkinter.StringVar()
# Create the Label that displays the time zone name.
self.output_label = tkinter.Label(
self.output_frame, borderwidth=1, relief='solid',
width=15, textvariable=self._ _timezone)
self.output_label.pack(side='right', padx=(1, 5), pady=5)
# This method creates the Quit button.
def _ _build_quit_button(self):
self.quit_button = tkinter.Button(
self.main_window, text='Quit',
command=self.main_window.destroy)
self.quit_button.pack(padx=5, pady=5)
# Callback function for the city_listbox widget.
def _ _display_time_zone(self, event):
# Get the current selections.
index = self.city_listbox.curselection()
# Get the city.
city = self.city_listbox.get(index[0])
# Determine the time zone.
if city == 'Denver':
self._ _timezone.set('Mountain')
elif city == 'Honolulu':
self._ _timezone.set('Hawaii-Aleutian')
elif city == 'Minneapolis':
self._ _timezone.set('Central')
elif city == 'New York':
self._ _timezone.set('Eastern')
elif city == 'San Francisco':
self._ _timezone.set('Pacific')
# Create an instance of the TimeZoneclass
if _ _name_ _ == '_ _main_ _':
time_zone = TimeZone()

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

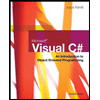
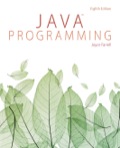
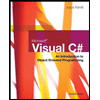
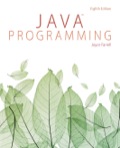