I have the following code in java: import java.util.ArrayList; import java.util.Scanner; public class Auxiliar { static ArrayList animales = new ArrayList<>(); static void imprimirAnimales() { for (Animal animal : animales) { System.out.println("Nombre: " + animal.getNombre() + ", Especie: " + animal.getEspecie() + ", Edad: " + animal.getEdad()); } } public static void main(String[] args) { Scanner scanner = new Scanner(System.in); int opcion = 0; while (opcion != 5) { System.out.println("¿Qué acción desea realizar?"); System.out.println("1. Añadir animal"); System.out.println("2. Modificar animal"); System.out.println("3. Eliminar animal"); System.out.println("4. Imprimir lista de animales"); System.out.println("5. Salir"); opcion = scanner.nextInt(); scanner.nextLine(); switch (opcion) { case 1: agregarAnimal(scanner); break; case 2: modificarAnimal(scanner); break; case 3: eliminarAnimal(scanner); break; case 4: imprimirAnimales(); break; case 5: break; default: System.out.println("Opción inválida"); break; } } } static void agregarAnimal(Scanner scanner) { System.out.println("Ingrese el nombre del animal:"); String nombre = scanner.nextLine(); System.out.println("Ingrese la especie del animal:"); String especie = scanner.nextLine(); System.out.println("Ingrese la edad del animal:"); int edad = scanner.nextInt(); scanner.nextLine(); Animal animal = new Animal(nombre, especie, edad); animales.add(animal); System.out.println("Animal agregado correctamente."); } static void modificarAnimal(Scanner scanner) { System.out.println("Ingrese el índice del animal a modificar:"); int indice = scanner.nextInt(); scanner.nextLine(); if (indice >= 0 && indice < animales.size()) { Animal animal = animales.get(indice); System.out.println("Ingrese el nuevo nombre del animal:"); String nombre = scanner.nextLine(); System.out.println("Ingrese la nueva especie del animal:"); String especie = scanner.nextLine(); System.out.println("Ingrese la nueva edad del animal:"); String edad = scanner.next(); animal.setNombre(nombre); animal.setEspecie(especie); animal.setEdad(Integer.parseInt(edad)); System.out.println("Animal modificado correctamente."); } else { System.out.println("Índice inválido."); } } static void eliminarAnimal(Scanner scanner) { System.out.println("Ingrese el índice del animal a eliminar:"); int indice = scanner.nextInt(); scanner.nextLine(); if (indice >= 0 && indice < animales.size()) { animales.remove(indice); System.out.println("Animal eliminado correctamente."); } else { System.out.println("Índice inválido."); } } } class Animal { private String nombre; private String especie; private int edad; public Animal(String nombre, String especie, int edad) { this.nombre = nombre; this.especie = especie; this.edad = edad; } public String getNombre() { return nombre; } public void setNombre(String nombre) { this.nombre = nombre; } public String getEspecie() { return especie; } public void setEspecie(String especie){ this.especie = especie; } public int getEdad() { return edad; } public void setEdad(int edad) { this.edad = edad; } } And I want to make the following changes to it: 1. The input asks for the name, species and age of the animal. Change this data to be: Name, code, sex, age, state and food. 2. If a value other than a number is added when requesting the age, the program generates an error. Can it be returned to be entered again in number format? 3. Once the whole process is finished (using option 5 to cancel) the list of all registered animals is printed
I have the following code in java:
import java.util.ArrayList;
import java.util.Scanner;
public class Auxiliar {
static ArrayList<Animal> animales = new ArrayList<>();
static void imprimirAnimales() {
for (Animal animal : animales) {
System.out.println("Nombre: " + animal.getNombre() + ", Especie: " + animal.getEspecie() + ", Edad: " + animal.getEdad());
}
}
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
int opcion = 0;
while (opcion != 5) {
System.out.println("¿Qué acción desea realizar?");
System.out.println("1. Añadir animal");
System.out.println("2. Modificar animal");
System.out.println("3. Eliminar animal");
System.out.println("4. Imprimir lista de animales");
System.out.println("5. Salir");
opcion = scanner.nextInt();
scanner.nextLine();
switch (opcion) {
case 1:
agregarAnimal(scanner);
break;
case 2:
modificarAnimal(scanner);
break;
case 3:
eliminarAnimal(scanner);
break;
case 4:
imprimirAnimales();
break;
case 5:
break;
default:
System.out.println("Opción inválida");
break;
}
}
}
static void agregarAnimal(Scanner scanner) {
System.out.println("Ingrese el nombre del animal:");
String nombre = scanner.nextLine();
System.out.println("Ingrese la especie del animal:");
String especie = scanner.nextLine();
System.out.println("Ingrese la edad del animal:");
int edad = scanner.nextInt();
scanner.nextLine();
Animal animal = new Animal(nombre, especie, edad);
animales.add(animal);
System.out.println("Animal agregado correctamente.");
}
static void modificarAnimal(Scanner scanner) {
System.out.println("Ingrese el índice del animal a modificar:");
int indice = scanner.nextInt();
scanner.nextLine();
if (indice >= 0 && indice < animales.size()) {
Animal animal = animales.get(indice);
System.out.println("Ingrese el nuevo nombre del animal:");
String nombre = scanner.nextLine();
System.out.println("Ingrese la nueva especie del animal:");
String especie = scanner.nextLine();
System.out.println("Ingrese la nueva edad del animal:");
String edad = scanner.next();
animal.setNombre(nombre);
animal.setEspecie(especie);
animal.setEdad(Integer.parseInt(edad));
System.out.println("Animal modificado correctamente.");
} else {
System.out.println("Índice inválido.");
}
}
static void eliminarAnimal(Scanner scanner) {
System.out.println("Ingrese el índice del animal a eliminar:");
int indice = scanner.nextInt();
scanner.nextLine();
if (indice >= 0 && indice < animales.size()) {
animales.remove(indice);
System.out.println("Animal eliminado correctamente.");
} else {
System.out.println("Índice inválido.");
}
}
}
class Animal {
private String nombre;
private String especie;
private int edad;
public Animal(String nombre, String especie, int edad) {
this.nombre = nombre;
this.especie = especie;
this.edad = edad;
}
public String getNombre() {
return nombre;
}
public void setNombre(String nombre) {
this.nombre = nombre;
}
public String getEspecie() {
return especie;
}
public void setEspecie(String especie){
this.especie = especie;
}
public int getEdad() {
return edad;
}
public void setEdad(int edad) {
this.edad = edad;
}
}
And I want to make the following changes to it:
1. The input asks for the name, species and age of the animal. Change this data to be: Name, code, sex, age, state and food.
2. If a value other than a number is added when requesting the age, the program generates an error. Can it be returned to be entered again in number format?
3. Once the whole process is finished (using option 5 to cancel) the list of all registered animals is printed

Step by step
Solved in 3 steps with 4 images

Hello! I'm not sure if it shows the second answer I got for the question where they had to be modified to invoke a list of lists.
The code worked perfectly for me, but the "delete animals" option does not make any changes to the list and it stays as it is. What I can do?
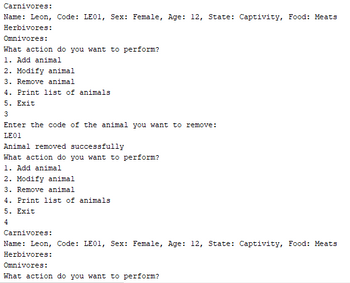
From the code supplied by the tutor, modify it so that:
1. Ask what kind of animal it is (for example, carnivore, herbivore, and omnivore) When prompting for the information, it is saved in a list for each of the three animal types (for example, lions in carnivores, bears in omnivores, giraffes in herbivores) and they are saved depending on the number that the user adds in each list.
2. At the end, a list of lists is printed that presents the data of each of the saved animals according to their type.
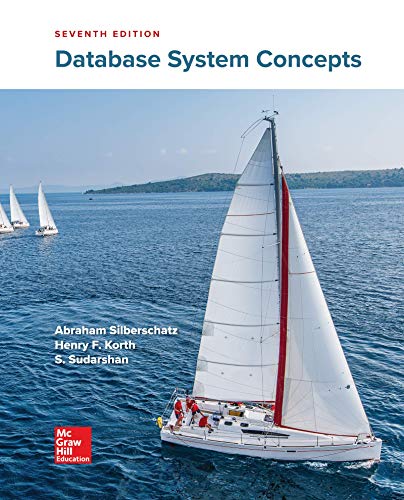
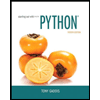
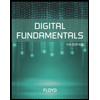
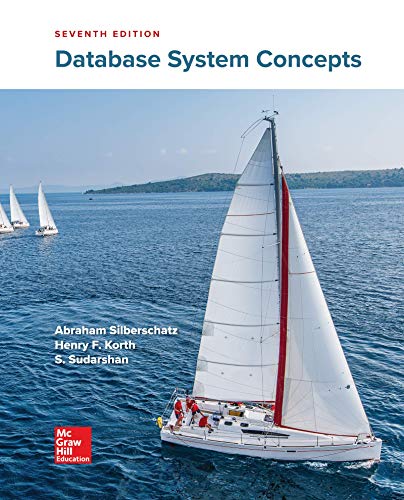
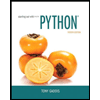
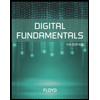
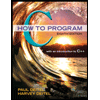
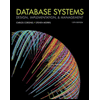
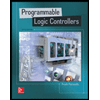