Add a proper function to the following linked list that will copy all elements with value above average into another list , then apply it to the list created in main function and print your resultant list. Example: l.copy(l2); will copy all elements with value greater than average into l2 #include using namespace std; struct node { int data; node *next,*prev; node(int d,node *p=0,node *n=0) { data=d; prev=p; next=n; } }; class list { node *head; public: list(); void print(); void add_end(int el); }; list::list() { head=0; } void list::add_end(int el) { if(head==0) head=new node(el); else { node *t=head; for(;t->next!=0;t=t->next); t->next=new node(el,t); } } void list::print() { for(node *t=head;t!=0;t=t->next) cout<data<<" "; cout<
Add a proper function to the following linked list that will copy all elements with value above average into another list , then apply it to the list created in main function and print your resultant list.
Example:
l.copy(l2); will copy all elements with value greater than average into l2
#include <iostream>
using namespace std;
struct node
{
int data;
node *next,*prev;
node(int d,node *p=0,node *n=0)
{ data=d; prev=p; next=n; }
};
class list
{
node *head;
public:
list();
void print();
void add_end(int el);
};
list::list()
{
head=0;
}
void list::add_end(int el)
{
if(head==0)
head=new node(el);
else
{
node *t=head;
for(;t->next!=0;t=t->next);
t->next=new node(el,t);
}
}
void list::print()
{
for(node *t=head;t!=0;t=t->next)
cout<<t->data<<" ";
cout<<endl;
}
void main()
{
list l;
l.add_end(7);
l.add_end(3);
l.add_end(9);
l.add_end(2);
l.add_end(3);
l.add_end(8);
l.add_end(6);
l.print();
system("pause");

Step by step
Solved in 4 steps with 3 images

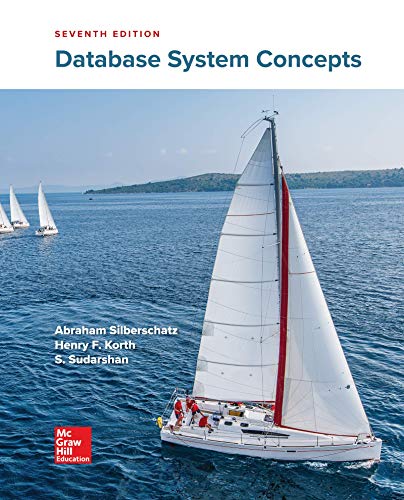
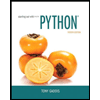
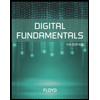
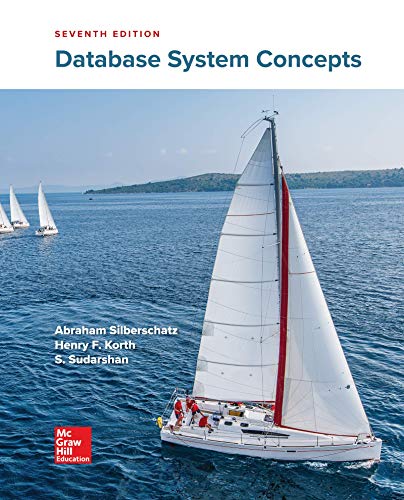
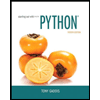
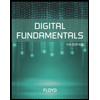
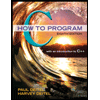
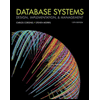
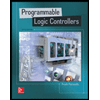