Input for this program will be a from a text file containing a valid ACSL Assembly Language program. Each line will contain one command. All labels will be single characters, and there will be 5 PRINT statements in the program. The data for the READ statements will follow the END statement, and the data file will have a maximum of 50 lines. Output to the screen, each PRINT statement in the program, labeled numerically, with the current value of the listed LOC. Let the user input the file name from the keyboard. If coding in C++, use the string class. Refer to the sample output below.
Input for this program will be a from a text file containing a valid ACSL Assembly Language program. Each line will contain one command. All labels will be single characters, and there will be 5 PRINT statements in the program. The data for the READ statements will follow the END statement, and the data file will have a maximum of 50 lines. Output to the screen, each PRINT statement in the program, labeled numerically, with the current value of the listed LOC. Let the user input the file name from the keyboard. If coding in C++, use the string class. Refer to the sample output below.
Chapter8: Advanced Method Concepts
Section: Chapter Questions
Problem 7RQ
Related questions
Question
C++

Transcribed Image Text:Write a program to compile and output ACSL Assembly Language programs. The ACSL Assembly
Language was introduced earlier in the semester with the Chapter 4 material. With this compiler only the
commands listed in the chart below will be used. Execution starts at the first line of the program and
continues sequentially, except for "branch" instructions, until the "end" instruction is encountered. The result
of each operation is stored in a special word of memory, called the "accumulator" (ACC). Each line of an
ACSL Assembly Language program has 3 components: a label (usually optional), an opcode (always
required), and a loc field (nearly always required):
The label, if present, is an alphanumeric character string beginning in the first column. A label
must begin with an alphabetic character (A through Z, or a through z), and labels are case-sensitive. The
label field is required for the DC opcode; it is optional for all other opcodes.
●
●
Valid opcodes are listed in the chart below; they are uppercase and case sensitive. Opcodes are
reserved words of the language and may not be used as a label.
The loc field is either a reference to a label (e.g., "ADD A") or immediate data (e.g., "LOAD
=123"). Only those opcodes with an asterisk in the following chart are allowed to use the immediate data
format of the loc field. The loc field is required for all opcodes, except for the END opcode. It is prohibited on
the END opcode.
OPCODE
*LOAD
STORE
*ADD
*SUB
*MULT
*DIV
BE
BG
BL
BU
END
READ
PRINT
DC
ACTION
Contents of LOC are placed in the ACC. LOC is unchanged.
Contents of ACC are placed in the LOC. ACC is unchanged.
Contents of LOC are added to the contents of the ACC. The sum
is stored in the ACC. LOC is unchanged. Addition is modulo
1,000,000.
Contents of LOC are subtracted from the contents of the ACC.
The difference is stored in the ACC. LOC is unchanged.
Subtraction is modulo 1,000,000.
The contents of LOC are multiplied by the contents of the ACC.
The product is stored in the ACC. LOC is unchanged.
Multiplication is modulo 1,000,000.
Contents of LOC are divided into the contents of the ACC. The
signed integer part of the quotient is stored in the ACC. LOC
is unchanged.
Branch to instruction labeled with LOC if ACC = 0.
Branch to instruction labeled with LOC if ACC > 0.
Branch to instruction labeled with LOC if ACC < 0.
Branch unconditionally to instruction labeled with LOC.
Program terminates. LOC field is ignored.
Read a signed integer (modulo 1,000,000) into LOC.
Print the contents of LOC.
The value of the memory word defined by the LABEL field is
defined to contain the specified constant. The LABEL field is
mandatory for this opcode. The ACC is not modified.

Transcribed Image Text:Input for this program will be a from a text file containing a valid ACSL Assembly Language program.
Each line will contain one command. All labels will be single characters, and there will be 5 PRINT
statements in the program. The data for the READ statements will follow the END statement, and the data file
will have a maximum of 50 lines. Output to the screen, each PRINT statement in the program, labeled
numerically, with the current value of the listed LOC. Let the user input the file name from the keyboard. If
coding in C++, use the string class. Refer to the sample output below.
Sample File:
A
D
NO
23
DC
1
READ B
READ C
PRINT C
LOAD =1
MULT
с
BE
D
STORE A
PRINT A
DIV B
BL
D
STORE C
PRINT C
SUB
BG
A
D
STORE B
PRINT B
ADD
A
STORE A
PRINT A
END
Sample Run:
Enter file name: assemb.txt
1. 3
2. 3
3. 1
4. -2
5. 1
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
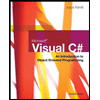
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
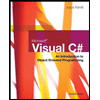
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,