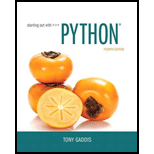
Car Class
Write a class named Car that has the following data attributes:
■ _ _year_model (for the car's year model)
■ _ _ make (for the make of the car)
■ _ _speed (for the car's current speed)
The Car class should have an _ _init_ _ method that accepts the car's year model and make as arguments. These values should be assigned to the object's _ _year_model and _ _make data attributes. It should also assign 0 to the _ _speed data attribute.
The class should also have the following methods:
■ accelerate
The accelerate method should add 5 to the speed data attribute each time it is called.
■ brake
The brake method should subtract 5 from the speed data attribute each time it is called.
■ get_speed
The get_speed method should return the current speed.
Next, design a

Learn your wayIncludes step-by-step video

Chapter 10 Solutions
Starting Out with Python (4th Edition)
Additional Engineering Textbook Solutions
Modern Database Management (12th Edition)
Introduction To Programming Using Visual Basic (11th Edition)
Web Development and Design Foundations with HTML5 (8th Edition)
Problem Solving with C++ (9th Edition)
Concepts Of Programming Languages
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
- RetailItem ClassWrite a class named RetailItem that holds data about an item in a retail store. The class should store the following data in attributes: item description, units in inventory, and price. Once you have written the class, write a program that creates three RetailItem objects and stores the following data in them: Description Units in Inventory Price Item #1 Jacket 12 59.95 Item #2 Designer Jeans 40 34.95 Item #3 Shirt 20 24.95arrow_forwardCircle Class (Easy) Write a Circle class that has the following member variables: radius : a double The class should have the following member functions: Default Constructor: default constructor that sets radius to 0.0. Constructor: accepts the radius of the circle as an argument. setRadius: an mutator function for the radius variable. getRadius: an accessor function for the radius variable. getArea: returns the area of the circle, which is calculated as area = pi * radius * radius getCircumference: returns the circumference of the circle, which is calculated as circumference = 2 * pi * radius Step1: Create a declaration of the class. Step2: Write a program that demonstrates the Circle class by asking the user for the circle’s radius, creating Circle objects, and then reporting the circle’s area, and circumference. You should create at least two circle objects, one sets the radius to 0.0 and one accepts the radius as an…arrow_forwardDouble Bubble For this exercise you need to create a Bubble class and construct two instances of the Bubble object. You will then take the two Bubble objects and combine them to create a new, larger combined Bubble object. This will be done using functions that take in these Bubble objects as parameters. The Bubble class contains one data member, radius_, and the corresponding accessor and mutator methods for radius_, GetRadius and SetRadius. Create a member function called CalculateVolume that computes for the volume of a bubble (sphere). Use the value 3.1415 for PI. Your main function has some skeleton code that asks the user for the radius of two bubbles. You will use this to create the two Bubble objects. You will create a CombineBubbles function that receives two references (two Bubble objects) and returns a Bubble object. Combining bubbles simply means creating a new Bubble object whose radius is the sum of the two Bubble objects' radii. Take note that the CombineBubbles function…arrow_forward
- (Date Class) Create a class called Date that includes three pieces of information as datamembers—a month (type int), a day (type int) and a year (type int). Your class should have a constructor with three parameters that uses the parameters to initialize the three data members. For thepurpose of this exercise, assume that the values provided for the year and day are correct, but ensurethat the month value is in the range 1–12; if it isn’t, set the month to 1. Provide a set and a get function for each data member. Provide a member function displayDate that displays the month, dayand year separated by forward slashes (/). Write a test program that demonstrates class Date’s capabilities.arrow_forwardIn java programming language Design a class named Account that contains:• A private double data field named annualInterestRate that stores the current interest rate.Assume all accounts have the same interest rate (i.e. static member data).• A private int data field named nextAccountNb that stores the next account number to beassigned (default 300). Assume all accounts use this data member to assign the account number while creating an account (i.e. static member data).• A private int data field named accounNb for the account.• A private String data field named f_name for first name.• A private String data field named l_name for last name.• A private double data field named balance for the account.• A private Date data field named dateCreated that stores the date when the account wascreated. (usejava.util.Dateclass)• A constructor that creates an account for a given first name, last name, and initial balance. It sets up a new account number, first name, last name, balance, date of…arrow_forwardemployee and production worker classes write an employee class that keeps data attributes for the following pieces of information: • employee name • employee number next, write a class named productionworker that is a subclass of the employee class. the productionworker class should keep data attributes for the following information: • shift number (an integer, such as 1, 2, or 3) • hourly pay rate the workday is divided into two shifts: day and night. the shift attribute will hold an integer value representing the shift that the employee works. the day shift is shift 1 and the night shift is shift 2. write the appropriate accessor and mutator methods for each class. once you have written the classes, write a program that creates an object of the productionworker class and prompts the user to enter data for each of the object’s data attributes. store the data in the object, then use the object’s accessor methods to retrieve it and display it on the screen. satak overfallowarrow_forward
- Construct a Time class containing integer data members seconds, minutes, and hours. Have the class contain two constructors: The first should be a default constructor having the prototype Time(int, int, int), which uses default values of 0 for each data member. The second constructor should accept a long integer representing a total number of seconds and disassemble the long integer into hours, minutes, and seconds. The final member method should display the class data members.arrow_forwardEmployee and ProductionWorker Classes Create an Employee class that has properties for the following data: Employee name Employee number Next, create a class named ProductionWorker that is derived from the Employee class. The ProductionWorker class should have properties to hold the following data: Shift number (an integer, such as 1, 2, or 3) Hourly pay rate The workday is divided into two shifts: day and night. The Shift property will hold an integer value representing the shift that the employee works. The day shift is shift 1 and the night shift is shift 2. Create an application that creates an object of the ProductionWorker class and lets the user enter data for each of the object’s properties. Retrieve the object’s properties and display their values.arrow_forward2. Car Class Write a class named Car that has the following data attributes: _ _year_model (for the car’s year model) _ _make (for the make of the car) _ _speed (for the car’s current speed) The Car class should have an _ _init_ _ method that accepts the car’s year model and make as arguments. These values should be assigned to the object’s _ _year_model and _ _make data attributes. It should also assign 0 to the _ _speed data attribute. The class should also have the following methods: accelerate The accelerate method should add 5 to the speed data attribute each time it is called. brake The brake method should subtract 5 from the speed data attribute each time it is called. get_speed The get_speed method should return the current speed. Next, design a program that creates a Car object then calls the accelerate method five times. After each call to the accelerate method, get the current speed of the car and display it. Then call the brake method five times. After each call to the…arrow_forward
- (Rational Class) Create a class called Rational for performing arithmetic with fractions.Write a program to test your class. Use integer variables to represent the private data of the class—the numerator and the denominator. Provide a constructor that enables an object of this class to beinitialized when it’s declared. The constructor should contain default values in case no initializersare provided and should store the fraction in reduced form. For example, the fractionwould be stored in the object as 1 in the numerator and 2 in the denominator. Provide publicmember functions that perform each of the following tasks:a) Adding two Rational numbers. The result should be stored in reduced form.b) Subtracting two Rational numbers. The result should be stored in reduced form.c) Multiplying two Rational numbers. The result should be stored in reduced form.d) Dividing two Rational numbers. The result should be stored in reduced form.e) Printing Rational numbers in the form a/b, where a is…arrow_forward(Rational Numbers) Create a class called Rational for performing arithmetic with fractions. Write a program to test your class. Use integer variables to represent the private instance variables of the class—the numerator and the denominator. Provide a constructor that enables an object of this class to be initialized when it's declared. The constructor should store the fraction in reduced form. The fraction 2/4 is equivalent to 1/2 and would be stored in the object as 1 in the numerator and 2 in the denominator. Provide a no-argument constructor with default values in case no initializers are provided. Provide public methods that perform each of the following operations: a) Add two Rational numbers: The result of the addition should be stored in reduced form. Implement this as a static method. b) Subtract two Rational numbers: The result of the subtraction should be stored in reduced form. Implement this as a static method.…arrow_forwardWrite base class with the name Shape . The Shape class have Private Variable: area, a double used to hold the shape's area. Public Member Functions: getArea the Accessor. This function should return the value in the member variable area. Parameterized constructor—Initializes area member. Defualt constructor— Empty body calcArea. This function should be a incomplete function.Next, define a class named Triangle . It should be child of Shape class. It should have the following members:Protected Member Variables: base, a integer used to hold the base of triangle.Height, a integer used to hold the height of the triangle. Public Member Functions: constructor—Initializes values for base, height. The overridden calcArea function in Circle described below.calcArea—calculates the area of the Triangle (1/2* base * height) and stores the result in the inherited member area.After you have created these classes, create a driver program that defines a Triangle object. Demonstrate that each object…arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
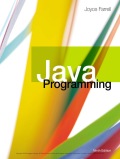
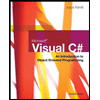