your askForYesNo method, how would you get the same result with only using one if/else if/else statement?? (IN JAVA PROGRAMMING) You also need to fix up your styling: All your method comments need to be in a block comment (/** comment */) and should include the parameters taken and values returned All your variables need to have a line comment (//comment) next to them explaining what they are going to be used for
With your askForYesNo method, how would you get the same result with only using one if/else if/else statement?? (IN JAVA PROGRAMMING)
You also need to fix up your styling:
- All your method comments need to be in a block comment (/** comment */) and should include the parameters taken and values returned
- All your variables need to have a line comment (//comment) next to them explaining what they are going to be used for
import java.util.Scanner;
/**
* 4.3CR User Input Functions
* a functions to read a number within a range, and read more natural boolean values from the user
*
* @author MEHAKPREET KAUR
* @version 04/08/2021
*/
public class UserInput {
// method to diplay askForInt
public static int askForInt(Scanner in, String ask, int min, int max){
int value; //value entered by user
System.out.print(ask + "(" + min + "-" + max + "): ");
value = in.nextInt();
//loop check for invalid value
while(value < min || value > max){
System.out.println("invald value");
System.out.print(ask + "(" + min + "-" + max + "): ");
value = in.nextInt();
}
return value;
}
//method to display askForDouble
public static double askForDouble(Scanner in, String ask, double min, double max){
double value;
System.out.print(ask + "(" + min + "-" + max + "): ");
value = in.nextDouble();
//loop check for invalid value
while(value < min || value > max){
System.out.println("invald value");
System.out.print(ask + "(" + min + "-" + max + "): ");
value = in.nextDouble();
}
return value;
}
//this method repetedly prompt the user to enter the valid boolean value until it falls under given range
public static Boolean askForYesNo(Scanner in, String ask) {
System.out.println(ask + " ( yes/no )");
String value = in.next();
Boolean resultValue = true;
Boolean isValid;
do {
value = value.toLowerCase();
if (value.equals("y") || value.equals("n") || value.equals("yes") || value.equals("no") || value.equals("true") || value.equals("false")) {
isValid = true;
} else {
isValid = false;
}
if (value.equals("y") || value.equals("yes") || value.equals("true")) {
resultValue = true;
break;
} else if (value.equals("n") || value.equals("no") || value.equals("false")) {
resultValue = false;
break;
} else {
System.out.println("Invalid Value");
System.out.println(ask + " ( yes/no )");
value = in.next();
}
} while (!isValid);
return resultValue;
}
//this is the main method of the program
public static void main(String[] args) {
Scanner in = new Scanner(System.in);
//All values are initialised so code will compile and run
int guess = -1; //user's guess (between 1 and 10)
double percent = -1; //a percentage (as a value between 0 and 1)
boolean again = false; //do they want to go again?
System.out.println("Testing prompt for int... the number should be saved in guess.");
System.out.println(" - Enter '30' -- should loop with error");
System.out.println(" - Enter '-1' -- should loop with error");
System.out.println(" - Enter '3' and it should work");
// guess = askForInt(in, "Enter a number", 1, 10);
guess = askForInt(in, "Enter a number", 1, 10);
System.out.println("Guess: " + guess);
System.out.println();
System.out.println("Testing prompt for double... the number should be saved in percent.");
System.out.println(" - Enter '30' -- should loop with error");
System.out.println(" - Enter '-2' -- should loop with error");
System.out.println(" - Enter '0.5' and it should work");
// percent = askForDouble(in, "Enter percent value", 0.0, 1.0);
percent = askForDouble(in, "Enter percent value", 0.0, 1.0);
System.out.println("Percent: " + percent);
System.out.println();
System.out.println("Testing prompt for yes/no... the result is saved in again.");
System.out.println(" - Extend these boolean tests by adding more messages to verify your solution!");
System.out.println(" - Enter 'yes' and it should succeed");
// again = askForYesNo(in, "Play again?");
again = askForYesNo(in, "Play again?");
System.out.println("Again: " + again);
System.out.println();
System.out.println(" - Verify that it can also read in false...");
// again = askForYesNo(in, "Play again?");
again = askForYesNo(in, "Play again?");
System.out.println("Again: " + again);
System.out.println();
System.out.println("Tests complete...");
}
}

Step by step
Solved in 4 steps with 1 images

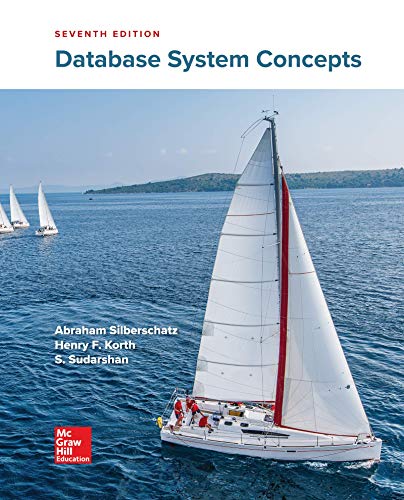
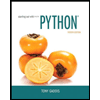
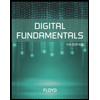
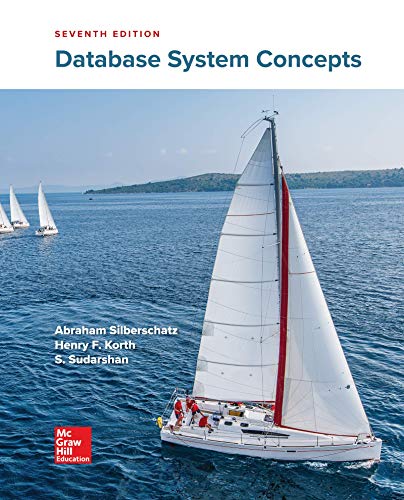
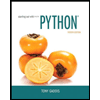
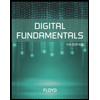
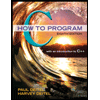
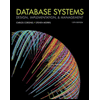
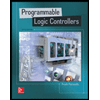