You must complete this in Python and the programs should not take any command-line arguments. You also need to make sure your programs will compile and run in at least a Linux environment. In this problem, you need to implement operation bit encoding for Assembly instructions. Given a line of text, your program should check whether it is a valid Assembly instruction type. If it is, your program should print out the opcode and optype of that instruction type; if the line of text is not exactly a valid Assembly instruction, your program should skip it and move to the next line of input without printing anything. Input Format The input to the program will consist of some number of lines. Each line contains some text, either a valid Assembly instruction type (with no extra whitespace or other characters, e.g. READ on a line by itself) or some other text. Constraints There are no specific constraints on the length or number of lines. They will be in a reasonable limit, as demonstrated by the included test cases, all of which are public. This is not a performance-centric problem. Output Format The output should consist of several lines, each of which contains a 4-bit opcode/optype string (e.g. 1000, corresponding to READ). If an instruction has undefined bits, such as NAND, those undefined bits should be represented wuth asterisks: *. Input 0 ADD Output 0 0000 Explanation 0 The input consists of a single line, containing the instruction "ADD", which corresponds to the opcode/optype 0000. Input 1 SUB SUBI Sample Output 1 00100011 Explanation 1 Both lines in this input are valid instruction types, with no additional whitespace or other characters, so they should be encoded according to the Assembly instruction table. Sample Input 2 NAND NOR WRITE JMP OUT Sample Output 2 010* 011* 1010 1011 1100 Explanation 2 All of the lines contain valid instructions that should be encoded. Since the second optype bit of both NAND and NOR is undefined in Assembly, it is left as a wildcard * here. Sample Input 3 ADDI a READ WRITE WRITE BEQ jjjj INP Sample Output 3 0001 1000 1001 1110 Explanation 3 This test case contains 3 invalid lines: 1. "a" is not a valid instruction type, so its line should be skipped. 2. "WRITE WRITE" is not a valid instruction type; even though WRITE is a valid instruction, the entire line should be considered for this problem. 3. "jjjj" is not a valid instruction type. Since 3 lines out of the 7 input lines are invalid, the output only contains 4 lines.
You must complete this in Python and the programs should not take any command-line arguments. You also need to make sure your programs will compile and run in at least a Linux environment.
In this problem, you need to implement operation bit encoding for Assembly instructions. Given a line of text, your
Input Format
The input to the program will consist of some number of lines. Each line contains some text, either a valid Assembly instruction type (with no extra whitespace or other characters, e.g. READ on a line by itself) or some other text.
Constraints
There are no specific constraints on the length or number of lines. They will be in a reasonable limit, as demonstrated by the included test cases, all of which are public. This is not a performance-centric problem.
Output Format
The output should consist of several lines, each of which contains a 4-bit opcode/optype string (e.g. 1000, corresponding to READ). If an instruction has undefined bits, such as NAND, those undefined bits should be represented wuth asterisks: *.
Input 0
ADD
Output 0
0000
Explanation 0
The input consists of a single line, containing the instruction "ADD", which corresponds to the opcode/optype 0000.
Input 1
SUB
SUBI
Sample Output 1
00100011
Explanation 1
Both lines in this input are valid instruction types, with no additional whitespace or other characters, so they should be encoded according to the Assembly instruction table.
Sample Input 2
NAND
NOR
WRITE
JMP
OUT
Sample Output 2
010*
011*
1010
1011
1100
Explanation 2
All of the lines contain valid instructions that should be encoded. Since the second optype bit of both NAND and NOR is undefined in Assembly, it is left as a wildcard * here.
Sample Input 3
ADDI
a
READ
WRITE WRITE
BEQ
jjjj
INP
Sample Output 3
0001
1000
1001
1110
Explanation 3
This test case contains 3 invalid lines: 1. "a" is not a valid instruction type, so its line should be skipped. 2. "WRITE WRITE" is not a valid instruction type; even though WRITE is a valid instruction, the entire line should be considered for this problem. 3. "jjjj" is not a valid instruction type. Since 3 lines out of the 7 input lines are invalid, the output only contains 4 lines.
![Instruction Set
Arithmetic
ADD
ADDI
SUB
SUBI
Logical
NAND
NOR
Memory
READ
WRITE
Branch
JMP
BEQ
INPUT/OUTPUT
INP
OUT
EXAMPLE
ADD RO, R1, R2 (i.e. RO = R1 + R2)
ADDI RO, R1, 8 (i.e. RO= R1 + 8)
SUB RO, R1, R2 (i.e. RO =R1 - R2)
SUBI RO, R1, 8 (i.e. RO= R1-8)
EXAMPLE
NAND RO, R1, R2 (i.e. RO= R1 NAND R2)
NOR RO, R1, R2 (i.e. R0 = R1 NAND R2)
EXAMPLE
READ RO, R1 (i.e. RO= MEM[R1])
WRITE RO, R1 (i.e. MEM[RO] = R1)
EXAMPLE
JMP RO (i.e. PCOut = RO)
BEQ RO, R1 (i.e. PCOut = R0 if R1==0)
EXAMPLE
INP RO (i.e. RO = MEM[KEYBOARD])
OUT RO, R1 (i.e. SCREEN[RO] = R1)
In[15] In[14] In[13] In[12]
OPCODE
OPTYPE
0
THE
0
1
0
1
0
1
In[15] In[14] In[13] In[12] In[11] In[10] In[9] In[8] In[7] In[6]
OPCODE
OPTYPE
DEST REGISTER
SRC1 REGISTER
0
In[15] In[14]
OPCODE
1
1
0
0
1 0
In[15] In[14] In[13] In[12]
OPCODE
OPTYPE
1
1
0
1
1
1
0
In[15] In[14] In[13] In[12]
OPCODE
OPTYPE
0
0
1
Any Reg: 000 to 111
1
In[13] |In[12] |In[11] In[10] In[9]
OPTYPE
LEFT SIDE
DEST REGISTER
DEST PTR REGISTER
In[11] In[10] In[9]
TARGET ADDRESS
In[11] In[10] In[9] In[8] In[7] In[6]
DEST REGISTER
SRC1 REGISTER
1
0
0
0
Any Reg: 000 to 111 Any Reg: 000 to 111
Any Reg: 000 to 111
In[11] In[10] In[9]
TARGET ADDRESS
Any Reg: 000 to 111
Any Reg: 000 to 111
In[8] In[7] In[6]
RIGHT SIDE
SRC PTR REGISTER
SRC REGISTER
In[8] In[7] In[6]
SRC REGISTER
Any Reg: 000 to 111
In[8] In[7] In[6]
SRC REGISTER
Any Reg: 000 to 111
In[5] In[4] In[3] In[2] In[1] In[0]
SRC2 REGISTER
Any Reg: 000-111
Six Bit Immediate Value (0-63)
Any Reg: 000-111
Six Bit Immediate Value (0-63)
In[5] In[4] In[3] In[2] In[1] In[0]
SRC2 REGISTER
UNUSED
Any Reg: 000 to
111
In[5] In[4] In[3] In[2] In[1] In[0]
In[5] In[4] In[3] In[2] In[1] In[0]
In[5] In[4] In[3] In[2] In[1] In[0]](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F7912ce54-d52f-486c-b093-cdbfa1d021eb%2Fec28c450-705f-4b6a-b3e3-08762434dc57%2F6p49g28_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 3 images

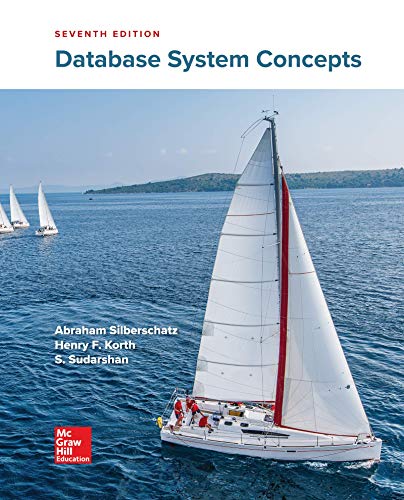
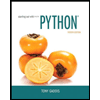
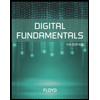
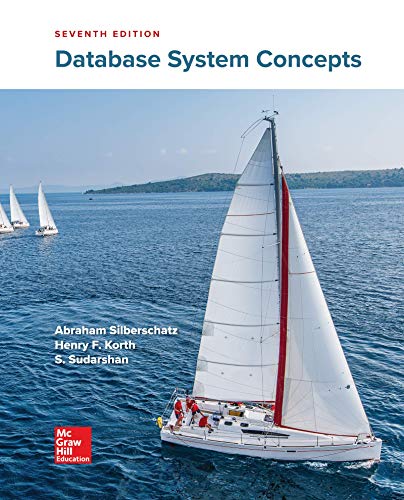
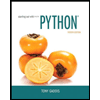
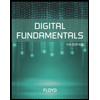
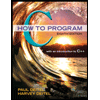
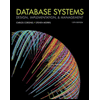
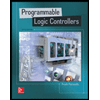