Write the following function: The function gets a root of a Binary Tree of ints, and a function f. it applies f to the data of each node in the tree. // applies f to each node of the tree under roo
Write the following function: The function gets a root of a Binary Tree of ints, and a function f. it applies f to the data of each node in the tree. // applies f to each node of the tree under roo
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Write the following function:
The function gets a root of a Binary Tree of ints, and a function f. it applies f to the data of each node in the tree.
// applies f to each node of the tree under root
void map(BTnode_t* root, int (*f)(int));
struct BTnode {
int value;
struct BTnode*left;
struct BTnode*right;
struct BTnode*parent;
};
typedef struct BTnode BTnode_t;
BTNODE.C:
BTnode_t* create_node(int val) {
BTnode_t* newNode = (BTnode_t*) malloc(sizeof(BTnode_t));
newNode->value = val;
newNode->left = NULL;
newNode->right = NULL;
newNode->parent = NULL;
returnnewNode;
}
void set_left_child(BTnode_t* parent, BTnode_t* left_child) {
if (parent)
parent->left = left_child;
if (left_child)
left_child->parent = parent;
}
void set_right_child(BTnode_t* parent, BTnode_t* right_child) {
if (parent)
parent->right = right_child;
if (right_child)
right_child->parent = parent;
}
void print_pre_order(BTnode_t* root) {
if (root == NULL)
return;
printf("%d ", root->value);
print_pre_order(root->left);
print_pre_order(root->right);
}
void print_in_order(BTnode_t* root) {
if (root == NULL)
return;
print_in_order(root->left);
printf("%d ", root->value);
print_in_order(root->right);
}
void print_post_order(BTnode_t* root) {
if (root == NULL)
return;
print_post_order(root->left);
print_post_order(root->right);
printf("%d ", root->value);
}
Test for the function:
int square(int x) { return x*x;}
bool test_q3map() {
/***
// creates the following tree
// 1
// / \
// 2 3
****/
BTnode_t* one = create_node(1);
BTnode_t* two = create_node(2);
BTnode_t* three = create_node(3);
set_left_child(one, two);
set_right_child(one, three);
map(one, square);
if (one->value == 1 && two->value == 4 && three->value == 9) {
printf("Q3-map ok\n");
returntrue;
}
else {
printf("Q3-map ERROR\n");
returnfalse;
}
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Recommended textbooks for you
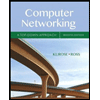
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
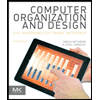
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
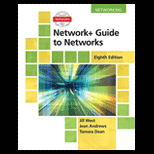
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
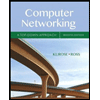
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
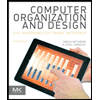
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
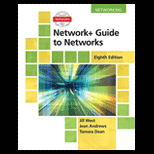
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
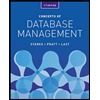
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
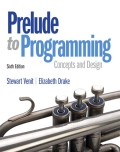
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
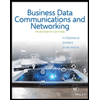
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY