Write an assembly language program that reads from the keyboard a positive integer N (N > 0) and finds and displays on the screen all the divisors of N and all the multiples of N up to 20N. Your program must satisfy the following: Allow the user to do any number of runs entering a new value for N each time. Define and use the following 4 procedures: ReadN: asks the user to enter a positive integer N (N > 0) and reads it. If the user enters an invalid value, it displays the error message “Invalid. It must be positive. Try again.”, and reads again until a valid value is entered. It returns the entered valid value in EAX. FindDivisors: finds and displays all the divisors of N. You must optimize the loop to run as efficiently as possible. The value of N is passed to the procedure in EAX. FindMultiples: calculates and displays on the screen all the multiples of N up to 20N. The value of N is passed to the procedure in EAX. AnotherRun: asks the user “Do you want another run? (Y/N): ”. If the user enters ‘Y’ or ‘y’, it returns 1 in the AL register otherwise it returns 0 in the AL register. The sample run on the next page shows how your program should run.
Write an assembly language program that reads from the keyboard a positive integer N (N > 0) and finds and displays on the screen all the divisors of N and all the multiples of N up to 20N. Your program must satisfy the following: Allow the user to do any number of runs entering a new value for N each time. Define and use the following 4 procedures: ReadN: asks the user to enter a positive integer N (N > 0) and reads it. If the user enters an invalid value, it displays the error message “Invalid. It must be positive. Try again.”, and reads again until a valid value is entered. It returns the entered valid value in EAX. FindDivisors: finds and displays all the divisors of N. You must optimize the loop to run as efficiently as possible. The value of N is passed to the procedure in EAX. FindMultiples: calculates and displays on the screen all the multiples of N up to 20N. The value of N is passed to the procedure in EAX. AnotherRun: asks the user “Do you want another run? (Y/N): ”. If the user enters ‘Y’ or ‘y’, it returns 1 in the AL register otherwise it returns 0 in the AL register. The sample run on the next page shows how your program should run.
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter8: Arrays And Strings
Section: Chapter Questions
Problem 21PE
Related questions
Topic Video
Question
Write an assembly language program that reads from the keyboard a positive integer N (N > 0) and finds and displays on the screen all the divisors of N and all the multiples of N up to 20N. Your program must satisfy the following: Allow the user to do any number of runs entering a new value for N each time. Define and use the following 4 procedures: ReadN: asks the user to enter a positive integer N (N > 0) and reads it. If the user enters an invalid value, it displays the error message “Invalid. It must be positive. Try again.”, and reads again until a valid value is entered. It returns the entered valid value in EAX. FindDivisors: finds and displays all the divisors of N. You must optimize the loop to run as efficiently as possible. The value of N is passed to the procedure in EAX. FindMultiples: calculates and displays on the screen all the multiples of N up to 20N. The value of N is passed to the procedure in EAX. AnotherRun: asks the user “Do you want another run? (Y/N): ”. If the user enters ‘Y’ or ‘y’, it returns 1 in the AL register otherwise it returns 0 in the AL register. The sample run on the next page shows how your program should run.

Transcribed Image Text:C:\WINDOWS\system32\cmd.exe
Enter an integer N (N> 0): -1
Invalid. It must be positive. Try again.
Enter an integer N (N> 0): 78
The divisors of 78 are:
1 2 3 6 13 26 39 78
The multiples of 78 are:
78 156 234 312 390 468 546 624 702 780 858 936 1014 1092 1170 1248 1326 1404 1482 1560
Do you want another run? (Y/N): y
Enter an integer N (N> 0): 91
The divisors of 91 are:
1 7 13 91
The multiples of 91 are:
91 182 273 364 455 546 637 728 819 910 1001 1092 1183 1274 1365 1456 1547 1638 1729 1820
Do you want another run? (Y/N): y
Enter an integer N (N> 0): 154
The divisors of 154 are:
1 2 7 11 14 22 77 154
The multiples of 154 are:
154 308 462 616 770 924 1078 1232 1386 1540 1694 1848 2002 2156 2310 2464 2618 2772 2926 3080
Do you want another run? (Y/N): y
Enter an integer N (N> 0): 240
The divisors of 240 are:
1 2 3 4 5 6 8 10 12 15 16 20 24 30 40 48 60 80 120 240
The multiples of 240 are:
240 480 720 960 1200 1440 1680 1920 2160 2400 2640 2880 3120 3360 3600 3840 4080 4320 4560 4800
Do you want another run? (Y/N): n
Press any key to continue . . . .

Transcribed Image Text:Write an assembly language program that reads from the keyboard a positive integer N (N> 0)
and finds and displays on the screen all the divisors of N and all the multiples of N up to 20N.
Your program must satisfy the following:
Allow the user to do any number of runs entering a new value for N each time.
• Define and use the following 4 procedures:
●
➤ ReadN: asks the user to enter a positive integer N (N> 0) and reads it. If the user enters
an invalid value, it displays the error message "Invalid. It must be positive. Try again.",
and reads again until a valid value is entered. It returns the entered valid value in EAX.
➤ Find Divisors: finds and displays all the divisors of N. You must optimize the loop to
run as efficiently as possible. The value of N is passed to the procedure in EAX.
Find Multiples: calculates and displays on the screen all the multiples of N up to 20N.
The value of N is passed to the procedure in EAX.
➤ Another Run: asks the user "Do you want another run? (Y/N): ". If the user enters 'Y'
or 'y', it returns 1 in the AL register otherwise it returns 0 in the AL register.
The sample run on the next page shows how your program should run.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
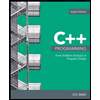
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
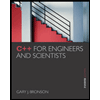
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
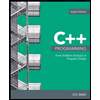
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
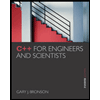
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr