Write a Python code This lab requires you to write a complete program using a condition controlled loop, a counter controlled loop, and an accumulator. The program is as follows: Write a program that will allow a grocery store to keep track of the total number of bottles collected for seven days. The program will calculate the total number of bottles returned for the week and the amount paid out (the total returned times .10 cents). The output of the program should include the total number of bottles returned and the total paid out. The program will ask the user if they have more data to enter and will end the program if they do not. Step 1: In the pseudocode below, declare the following variables under the documentation for Step 1. A variable called totalBottles that is initialized to 0 This variable will store the accumulated bottle values A variable called counter and that is initialized to 1 This variable will control the loop A variable called todayBottles that is initialized to 0 This variable will store the number of bottles returned on a day A variable called totalPayout that is initialized to 0 This variable will store the calculated value of totalBottles times .10 A variable called keepGoing that is initialized to "y" This variable will be used to run the program again Step 2: In the pseudocode below, make calls to the following functions under the documentation for Step 2. A function call to getBottles that returns the total number of bottles for the week. A function called calcPayout that passes totalPayout and It returns the total payout for the week. A module called printInfo that passes totalBottles and Step 3: In the pseudocode below, write a condition controlled while loop around your function calls using the keepGoing variable under the documentation for Step 3. Complete Steps 1-3 below: Module main () // Step 1: Declare variables below ___________________________________________________ ___________________________________________________ ___________________________________________________ ___________________________________________________ ___________________________________________________ // Step 3: Loop to run program again While _________________________________ // Step 2: Call functions totalBottles = _________________________________ totalPayout = _________________________________ ________________________________________________ Display "Do you want to enter another week’s worth of data?" Display "(Enter y or n)" Input _________________________________ End While End Module Step 4: In the pseudocode below, write the missing lines, including: The 3 missing variable declarations and initializing them to 0 The missing condition (Hint: should run seven iterations) The missing input variable The missing accumulator The increment statement for the counter The missing return statement // getBottles function Function getBottles() NBR_OF_DAYS = 7 // Declare and initialize totalBottles, todayBottles, counter to 0 ___________________________________ ___________________________________ ___________________________________ While b._______________________________ Display "Enter number of bottles returned for day #", counter, ":" Input c.______________________________ ___________________________________ ___________________________________ End While Return f._______________________________ End Function Step 5: In the pseudocode below, write the missing lines, including: The missing parameter list The missing calculation The missing return statement // calcPayout function Function Real calcPayout(a.______________________) PAYOUT_PER_BOTTLE = .10 totalPayout = 0 // resets to 0 for multiple runs ______________________________ Return c._________________________ End Function Step 6: In the pseudocode below, write the missing lines, including: The missing parameter list The missing display statement The missing display statement // printInfo module Module printInfo(a.______________________________________) _______________________________________________ _______________________________________________ End Module
Write a Python code
This lab requires you to write a complete
Write a program that will allow a grocery store to keep track of the total number of bottles collected for seven days.
The program will calculate the total number of bottles returned for the week and the amount paid out (the total returned times .10 cents).
The output of the program should include the total number of bottles returned and the total paid out.
The program will ask the user if they have more data to enter and will end the program if they do not.
Step 1: In the pseudocode below, declare the following variables under the documentation for Step 1.
- A variable called totalBottles that is initialized to 0
- This variable will store the accumulated bottle values
- A variable called counter and that is initialized to 1
- This variable will control the loop
- A variable called todayBottles that is initialized to 0
- This variable will store the number of bottles returned on a day
- A variable called totalPayout that is initialized to 0
- This variable will store the calculated value of totalBottles times .10
- A variable called keepGoing that is initialized to "y"
- This variable will be used to run the program again
Step 2: In the pseudocode below, make calls to the following functions under the documentation for Step 2.
- A function call to getBottles that returns the total number of bottles for the week.
- A function called calcPayout that passes totalPayout and It returns the total payout for the week.
- A module called printInfo that passes totalBottles and
Step 3: In the pseudocode below, write a condition controlled while loop around your function calls using the keepGoing variable under the documentation for Step 3.
Complete Steps 1-3 below:
Module main ()
// Step 1: Declare variables below
___________________________________________________
___________________________________________________
___________________________________________________
___________________________________________________
___________________________________________________
// Step 3: Loop to run program again
While _________________________________
// Step 2: Call functions
totalBottles = _________________________________
totalPayout = _________________________________
________________________________________________
Display "Do you want to enter another week’s worth of data?"
Display "(Enter y or n)"
Input _________________________________
End While
End Module
Step 4: In the pseudocode below, write the missing lines, including:
- The 3 missing variable declarations and initializing them to 0
- The missing condition (Hint: should run seven iterations)
- The missing input variable
- The missing accumulator
- The increment statement for the counter
- The missing return statement
// getBottles function
Function getBottles()
NBR_OF_DAYS = 7
// Declare and initialize totalBottles, todayBottles, counter to 0
- ___________________________________
___________________________________
___________________________________
While b._______________________________
Display "Enter number of bottles returned for day #",
counter, ":"
Input c.______________________________
- ___________________________________
- ___________________________________
End While
Return f._______________________________
End Function
Step 5: In the pseudocode below, write the missing lines, including:
- The missing parameter list
- The missing calculation
- The missing return statement
// calcPayout function
Function Real calcPayout(a.______________________)
PAYOUT_PER_BOTTLE = .10
totalPayout = 0 // resets to 0 for multiple runs
- ______________________________
Return c._________________________
End Function
Step 6: In the pseudocode below, write the missing lines, including:
- The missing parameter list
- The missing display statement
- The missing display statement
// printInfo module
Module printInfo(a.______________________________________)
- _______________________________________________
- _______________________________________________
End Module

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

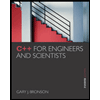
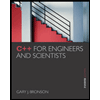