Write a program in java for the following scenario and write a DriverQ class to test your construction: i) Create a class Vehicle which has the following members: Member Variables: • vehicleNumber • ownerName • mulkiaAmt • insuranceAmt Member Methods: A constructor to initialize the vehicleNumber, ownerName, mulkiaAmt and insuranceAmt. An abstract method calcDiscount() to calculate and return the discount amount. An abstract method calcTotalAmt() to calculate and return the amount to be paid. A display( ) method to display the details of the vehicle. ii)Create a subclass PetrolVehicle which is inherited from Vehicle class and has the following details: Member Variables: • serviceCharges Member Methods: • A constructor which will invoke the super class constructor and initialize serviceCharges. • Implement the calcDiscount() method to calculate the discount as, Service Charges Discount 0- 50 1.000 50 - 75 2.000 75 - 90 3.000 90 - 100 4.000 Implement the calcTotalAmt() method to calculate the total amount as, totalAmt =(mulkiaAmt + insuranceAmt + serviceCharges) - discount A method display() to display all the details of the vehicle. iii) Create a driver class named DriverQ which contains the main method. Create objects as follows, PetrolVehicle ("90335MR", “Hashim", 21.0, 45.0, 10.0) Print details of the PetrolVehicle objects.
Write a program in java for the following scenario and write a DriverQ class to test your construction: i) Create a class Vehicle which has the following members: Member Variables: • vehicleNumber • ownerName • mulkiaAmt • insuranceAmt Member Methods: A constructor to initialize the vehicleNumber, ownerName, mulkiaAmt and insuranceAmt. An abstract method calcDiscount() to calculate and return the discount amount. An abstract method calcTotalAmt() to calculate and return the amount to be paid. A display( ) method to display the details of the vehicle. ii)Create a subclass PetrolVehicle which is inherited from Vehicle class and has the following details: Member Variables: • serviceCharges Member Methods: • A constructor which will invoke the super class constructor and initialize serviceCharges. • Implement the calcDiscount() method to calculate the discount as, Service Charges Discount 0- 50 1.000 50 - 75 2.000 75 - 90 3.000 90 - 100 4.000 Implement the calcTotalAmt() method to calculate the total amount as, totalAmt =(mulkiaAmt + insuranceAmt + serviceCharges) - discount A method display() to display all the details of the vehicle. iii) Create a driver class named DriverQ which contains the main method. Create objects as follows, PetrolVehicle ("90335MR", “Hashim", 21.0, 45.0, 10.0) Print details of the PetrolVehicle objects.
Chapter11: Advanced Inheritance Concepts
Section: Chapter Questions
Problem 2PE
Related questions
Concept explainers
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Question

Transcribed Image Text:Write a program in java for the following scenario and write a DriverQ class to test your construction:
i)
Create a class Vehicle which has the following members:
Member Variables:
• vehicleNumber
• ownerName
• mulkiaAmt
insuranceAmt
Member Methods:
A constructor to initialize the vehicleNumber, ownerName, mulkiaAmt and insuranceAmt.
An abstract method calcDiscount() to calculate and return the discount amount.
An abstract method calcTotalAmt() to calculate and return the amount to be paid.
• A display( ) method to display the details of the vehicle.
ii)Create a subclass PetrolVehicle which is inherited from Vehicle class and has the following details:
Member Variables:
• serviceCharges
Member Methods:
A constructor which will invoke the super class constructor and initialize serviceCharges.
• Implement the calcDiscount() method to calculate the discount as,
Service Charges
Discount
0- 50
1.000
50 - 75
75 - 90
2.000
3.000
90 - 100
4.000
Implement the calcTotalAmt() method to calculate the total amount as,
totalAmt =(mulkiaAmt + insuranceAmt + serviceCharges) - discount
A method display() to display all the details of the vehicle.
ii)
Create a driver class named DriverQ which contains the main method. Create objects as follows,
PetrolVehicle ("90335MR", "Hashim", 21.0, 45.0, 10.0)
iv)
Print details of the PetrolVehicle objects.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
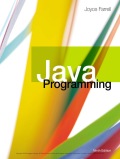
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
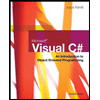
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
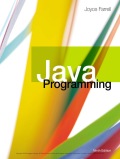
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
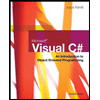
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,