Write a Java program to give the user the ability to determine the day of a given date. The user shall input 3 integers day, month, and year. You are NOT required to check if the values entered by the user are in normal range. Using the given formula, your program must output an integer that corresponds to the day of
Write a Java program to give the user the ability to determine the day of a given date. The user shall input 3 integers day, month, and year. You are NOT required to check if the values entered by the user are in normal range. Using the given formula, your program must output an integer that corresponds to the day of
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter5: Control Structures Ii (repetition)
Section: Chapter Questions
Problem 30PE
Related questions
Question

Transcribed Image Text:Write a Java program to give the user the ability to determine the day of a given date. The user shall input 3
integers day, month, and year. You are NOT required to check if the values entered by the user are in
normal range. Using the given formula, your program must output an integer that corresponds to the day of
the date.
(Note: this formula is
true only for 20* century dates)
exactDay = (day + monthCode + centuryCode + updatedYear + leapYears) % 7
exactDay: is an integer between 0 & 6, where 0->Saturday, 1->Sunday, .. 6->Friday
day: is the day entered by the user.
monthCode: An integer which can be determined from the given table below.
centuryCode: Can be considered as 6 always (only for 20" century).
updatedYear: An integer representing the last 2 digits from the given year entered by the user.
For example: if the user enters year as: 2018, then the updated year will be: 18.
leapYears: An integer representing the number of leap years in updatedYear variable. For
example: if the updatedYear=18 => we have 18/4=4 leap years.
Table
month
monthCode
4, 7
1, 10
5
2, 3, 11
4
9, 12
Example: if the user enters day, month, year as follows: 12 |/ 2018
Entered month by the user is 11, therefore the monthCode=4 (from the table)
centurayCode = 6. (constant)
updatedYear = 18 (last 2 digits from year: 2018)
leapYears = 18/4 = 4 (Integer division)
Therefore,
exactDay = (12 + 4+ 6 + 18 + 4) % 7
- 44 % 7
-2 (this should be the output of your program)
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
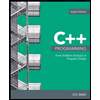
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
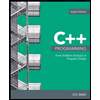
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning