Write a code that : - Accepts integers as inputs and append them to the doubly linked list till it receives -1 - Then swaps the head and the second node in the created doubly linked list - Note: you should check: - If it is an empty linked list and then print('Empty!') - your algorithm should work for a link list with a single node (and should return the same list for that case!) Some of the code in main.py has been written below: class Node: def __init__(self, data): self.data = data self.next = None self.prev = None # Empty Doubly Linked List class DoublyLinkedList: def __init__(self): self.head = None # Inserts a new node on the front of list def append(self, new_data): if self.head == None: self.head = new_data self.tail = new_data else: self.tail.next = new_data new_data.prev = self.tail self.tail = new_data def printList(self): node = self.head while(node is not None): print(node.data), node = node.next def swap(self): # ** Your code goes here ** return if __name__ == '__main__': # ** Your code goes here ** LL.swap() LL.printList()
Write a code that : - Accepts integers as inputs and append them to the doubly linked list till it receives -1 - Then swaps the head and the second node in the created doubly linked list - Note: you should check: - If it is an empty linked list and then print('Empty!') - your
Some of the code in main.py has been written below:
class Node:
def __init__(self, data):
self.data = data
self.next = None
self.prev = None
# Empty Doubly Linked List
class DoublyLinkedList:
def __init__(self):
self.head = None
# Inserts a new node on the front of list
def append(self, new_data):
if self.head == None:
self.head = new_data
self.tail = new_data
else:
self.tail.next = new_data
new_data.prev = self.tail
self.tail = new_data
def printList(self):
node = self.head
while(node is not None):
print(node.data),
node = node.next
def swap(self):
# ** Your code goes here **
return
if __name__ == '__main__':
# ** Your code goes here **
LL.swap()
LL.printList()

Step by step
Solved in 4 steps with 3 images

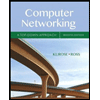
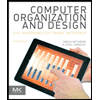
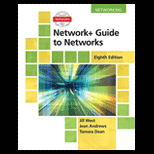
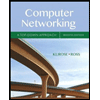
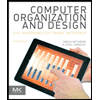
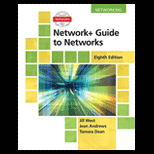
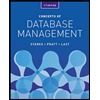
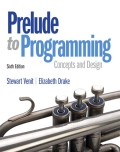
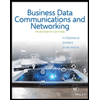