Write a code in java programming. After the pandemic, you decide to open a fast-food restaurant. Since this is a start-up business, you only focus on selling one meal: burger and fries. After a couple of days of observation, you found that you need to have at least one chef for making the burger and one chef for making fries for serving customers. you need to simulate this restaurant using the Java thread. In the restaurant, you have one burger chef, one fries chef, and one waitress. In this simulation, the burger chef can produce a burger every 3 seconds, and the fries chef can produce a set of fries every 2 seconds. There is a kitchen table between the two chefs. The table is used to temporarily place the burgers or fries. Since the table has a limited size, it only allows having a maximum of 5 burgers and 5 sets of fries (total 10 food items here). For example, If the table has 5 burgers, the burger chef should start waiting until the table has a free space. Next, If the kitchen table has at least one burger and one set of fries, either the burger chef or fries chef can combine them to make a combo and place it on the ready table. Note that in your simulation, you need to show me who makes the combo. Finally, the waitress can serve these combos to customers from the ready table. The average serving time for one customer is 10 seconds, but the ready table can only have a maximum of 3 meals. Thus, a chef should wait until ready table are available before making a new combo. All wait times should take 1 second, this apply to, for example, the waitress' waiting time if there is no meal on the ready table or chef's waiting time if either the ready table or the kitchen table are not available. // You are free to add any attributes or methods you need. public class BurgerChef implements Runnable { private String name = "BurgerChef"; privateintWAIT_TIME = 1000; privateintMAKE_TIME = 3000; @Override publicvoid run() { // TODO Auto-generated method stub } } // You are free to add any attributes or methods you need. public class FriesChef implements Runnable{ private String name = "FriesChef"; privateintWAIT_TIME = 1000; privateintMAKE_TIME = 2000; @Override publicvoid run() { // TODO Auto-generated method stub } } //A work table which for chefs to place Burgers or Fries. //You need to handle race condition here. public class KitchenTable { publicstaticintlimit = 10; } //Here is the driver class public class Main { publicstaticvoid main(String[] args) { Thread burgerChef = new Thread(new BurgerChef(), "Burger Chef"); Thread friesChef = new Thread(new FriesChef(), "Fries Chef"); Thread waitress = new Thread(new Waitress(), "Waitress"); burgerChef.start(); friesChef.start(); waitress.start(); } } /A table which the meals are put as soon as they become ready //You need to handle race condition here. public class ReadyTable { publicstaticintlimit = 3; } // You are free to add any attributes or methods you need. public class Waitress implements Runnable{ private String name = "Waitress"; privateintWAIT_TIME = 1000; privateintSERVE_TIME = 10000; // 10 second @Override publicvoid run() { // TODO Auto-generated method stub } }
Write a code in java programming.
After the pandemic, you decide to open a fast-food restaurant. Since this is a start-up business, you only focus on selling one meal: burger and fries. After a couple of days of observation, you found that you need to have at least one chef for making the burger and one chef for making fries for serving customers. you need to simulate this restaurant using the Java thread.
In the restaurant, you have one burger chef, one fries chef, and one waitress. In this simulation, the
burger chef can produce a burger every 3 seconds, and the fries chef can produce a set of fries every 2 seconds.
There is a kitchen table between the two chefs. The table is used to temporarily place the burgers or fries. Since the table has a limited size, it only allows having a maximum of 5 burgers and 5 sets of fries (total 10 food items here). For example, If the table has 5 burgers, the burger chef should start waiting until the table has a free space. Next, If the kitchen table has at least one burger and one set of fries, either the burger chef or fries chef can combine them to make a combo and place it on the ready table.
Note that in your simulation, you need to show me who makes the combo.
Finally, the waitress can serve these combos to customers from the ready table. The average serving time for one customer is 10 seconds, but the ready table can only have a maximum of 3 meals. Thus, a chef should wait until ready table are available before making a new combo. All wait times should take 1 second, this apply to, for example, the waitress' waiting time if there is no meal on the ready table or chef's waiting time if either the ready table or the kitchen table are not available.
// You are free to add any attributes or methods you need.
public class BurgerChef implements Runnable {
private String name = "BurgerChef";
privateintWAIT_TIME = 1000;
privateintMAKE_TIME = 3000;
@Override
publicvoid run() {
// TODO Auto-generated method stub
}
}
// You are free to add any attributes or methods you need.
public class FriesChef implements Runnable{
private String name = "FriesChef";
privateintWAIT_TIME = 1000;
privateintMAKE_TIME = 2000;
@Override
publicvoid run() {
// TODO Auto-generated method stub
}
}
//A work table which for chefs to place Burgers or Fries.
//You need to handle race condition here.
public class KitchenTable {
publicstaticintlimit = 10;
}
//Here is the driver class
public class Main {
publicstaticvoid main(String[] args) {
Thread burgerChef = new Thread(new BurgerChef(), "Burger Chef");
Thread friesChef = new Thread(new FriesChef(), "Fries Chef");
Thread waitress = new Thread(new Waitress(), "Waitress");
burgerChef.start();
friesChef.start();
waitress.start();
}
}
/A table which the meals are put as soon as they become ready
//You need to handle race condition here.
public class ReadyTable {
publicstaticintlimit = 3;
}
// You are free to add any attributes or methods you need.
public class Waitress implements Runnable{
private String name = "Waitress";
privateintWAIT_TIME = 1000;
privateintSERVE_TIME = 10000; // 10 second
@Override
publicvoid run() {
// TODO Auto-generated method stub
}
}
![[Action] Waitress serve a meal
[Status] meals left: 2
[Action] BurgerChef add a burger on the kitchen table
[Status] burgers left: 3
[Action] BurgerChef makes Burger and Fries and place it on the ready table
[Status] burgers left: 2, fries left: 4, meals left: 3
[Action] FriesChef add a fries on the kitchen table
[Status] fries left: 5
[Action] BurgerChef add a burger on the kitchen table
[Status] burgers left: 3
[Action] BurgerChef add a burger on the kitchen table
[Status] burgers left: 4
[Action] Waitress serve a meal
[Status] meals left: 2.
[Action] BurgerChef add a burger on the kitchen table
[Status] burgers left: 5
[Action] BurgerChef makes Burger and Fries and place it on the ready table
[Status] burgers left: 4, fries left: 4, meals left: 3
=======
[Action] FriesChef add a fries on the kitchen table
[Status] fries left: 5
[Action] BurgerChef add a burger on the kitchen table
[Status] burgers left: 5
[Action] Waitress serve a meal
[Status] meals left: 2
[Action] BurgerChef add a burger on the kitchen table
[Status] burgers left: 5
[Action] Fries Chef add a fries on the kitchen table
[Action] Fries Chef makes Burger and Fries and place it on the ready table.
[Status] burgers left: 4, fries left: 4, meals left: 3
[Status] fries left: 5.
[Action] Waitress serve a meal
[Status] meals left: 2
=================
[Status] burgers left: 5
[Action] Waitress serve a meal
[Status] meals left: 2
=========================
[Action] BurgerChef makes Burger and Fries and place it on the ready table
[Status] burgers left: 4, fries left: 4, meals left: 3
=========
[Action] FriesChef add a fries on the kitchen table
[Status] fries left: 5
[Action] BurgerChef add a burger on the kitchen table
[Action] Fries Chef add a fries on the kitchen table
[Status] fries left: 5
[Action] BurgerChef makes Burger and Fries and place it on the ready table
[Status] burgers left: 4, fries left: 4, meals left: 3
=========
=================
==============](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F8e86230f-5cdf-4308-b446-8633bf74e33b%2F26be85f4-0d67-486c-ac48-d20551aa7cc3%2Faxy7q9l_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

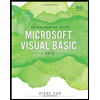
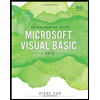