Write a C3# program that implements a class called MyToys. Your class should have the following data items: Toy Number (string), Toy Description (string), Toy Value (in $) (double), and Year Acquired (integer). You need to define properties with appropriate accessor functions for each of the data items. Methods should include a default constructor that sets values to the empty string or zero, a constructor that allows the user to specify all four values for the data items, a member method called DisplayToyInfo that outputs all of the information for the given toy in a reasonable format. Challenge Problem: Code your set accessors to restrict your Toy Value data member to positive numbers and your Year Acquired data member to be prior to 2021. //my main() method for this program contains the following code MyToys mtToy1 = new MyToys(); mtToy1.ToyNumber = "Toy42"; mtToy1.ToyDescription = "Garfield Stuffed Cat"; mtToy1.ToyValue = 29.99; mtToy1.YearAcquired = 2001; Console.WriteLine("Display Toy1 Information"); mtToy1.DisplayToyInfo(); MyToys mtToy2 = new MyToys(); Console.WriteLine("\nDisplay Toy2 Information"); mtToy2.DisplayToyInfo(); MyToys mtToy3 = new MyToys("Toy13", "Silver Corvette Hot Wheels", 599.95, 1963); Console.WriteLine("\nDisplay Toy3 Information"); mtToy3.DisplayToyInfo();
Write a C3# program that implements a class called MyToys. Your class should have the following data items: Toy Number
(string), Toy Description (string), Toy Value (in $) (double), and Year Acquired (integer). You need to define properties
with appropriate accessor functions for each of the data items. Methods should include a default constructor that sets
values to the empty string or zero, a constructor that allows the user to specify all four values for the data items, a
member method called DisplayToyInfo that outputs all of the information for the given toy in a reasonable format.
Challenge Problem: Code your set accessors to restrict your Toy Value data member to positive numbers and your Year
Acquired data member to be prior to 2021.
//my main() method for this program contains the following code
MyToys mtToy1 = new MyToys();
mtToy1.ToyNumber = "Toy42";
mtToy1.ToyDescription = "Garfield Stuffed Cat";
mtToy1.ToyValue = 29.99;
mtToy1.YearAcquired = 2001;
Console.WriteLine("Display Toy1 Information");
mtToy1.DisplayToyInfo();
MyToys mtToy2 = new MyToys();
Console.WriteLine("\nDisplay Toy2 Information");
mtToy2.DisplayToyInfo();
MyToys mtToy3 = new MyToys("Toy13", "Silver Corvette Hot Wheels", 599.95, 1963);
Console.WriteLine("\nDisplay Toy3 Information");
mtToy3.DisplayToyInfo();

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 3 images

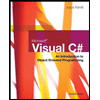
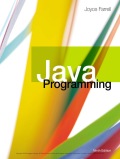
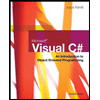
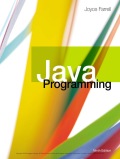