Write a C++ program to determine whether the given singly linked list is a palindrome. A palindrome is a sequence of characters that reads the same forwards and backward. You must implement a solution that uses both a linked list and a stack. The program should take the following steps: Traverse the linked list and push each element onto a stack. While traversing the linked list, compare each element with the top element of the stack (pop the stack as you compare). If all elements match (i.e., the linked list is a palindrome), output "Palindrome." Otherwise, output "Not a Palindrome." Example: Input Linked List: 1 -> 2 -> 3 -> 2 -> 1 Output: Palindrome Input Linked List: 1 -> 2 -> 3 -> 4 -> 5 Output: Not a Palindrome Implement the solution using both a linked list and a stack to efficiently check if the given linked list forms a palindrome or not
Write a C++ program to determine whether the given singly linked list is a palindrome. A palindrome is a sequence of characters that reads the same forwards and backward. You must implement a solution that uses both a linked list and a stack. The program should take the following steps: Traverse the linked list and push each element onto a stack. While traversing the linked list, compare each element with the top element of the stack (pop the stack as you compare). If all elements match (i.e., the linked list is a palindrome), output "Palindrome." Otherwise, output "Not a Palindrome." Example: Input Linked List: 1 -> 2 -> 3 -> 2 -> 1 Output: Palindrome Input Linked List: 1 -> 2 -> 3 -> 4 -> 5 Output: Not a Palindrome Implement the solution using both a linked list and a stack to efficiently check if the given linked list forms a palindrome or not
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter18: Stacks And Queues
Section: Chapter Questions
Problem 21SA
Related questions
Question
Give correct answer. Don't copy other's work.

Transcribed Image Text:Write a C++ program to determine whether the
given singly linked list is a palindrome. A palindrome
is a sequence of characters that reads the same
forwards and backward. You must implement a
solution that uses both a linked list and a stack. The
program should take the following steps: Traverse
the linked list and push each element onto a stack.
While traversing the linked list, compare each
element with the top element of the stack (pop the
stack as you compare). If all elements match (i.e.,
the linked list is a palindrome), output "Palindrome."
Otherwise, output "Not a Palindrome." Example:
Input Linked List: 1 -> 2 -> 3 -> 2 -> 1 Output:
Palindrome Input Linked List: 1 -> 2 -> 3 -> 4 -> 5
Output: Not a Palindrome Implement the solution
using both a linked list and a stack to efficiently
check if the given linked list forms a palindrome or
not
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
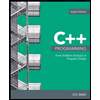
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
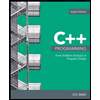
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning