Write a C++ program that asks the user to select a color and a shape to draw. In main, begin by declaring variables and displaying the class header, and cout an explanation of the program. You may include this in your header or make it separate. You will need constants for the maximum color number and for the maximum picture number as well as a value that indicates the user has finished drawing. These will look like: //Constants const int QUIT{ 6}; const int MAX_COLORS{ 6}; const int MAX_PIX{3}; At this point, create a handle to the standard output device (the console) using: HANDLE screen = GetStdHandle(STD_OUTPUT_HANDLE); You will use this handle to access the screen to change colors. You will also need to #include . Open a do-while loop. This is the “play loop.” Provide a menu of colors and ask the user to select a color for the drawing. There will be 6 menu items, 5 colors, blue, green, cyan, red and purple. These will be selections 1-5. Selection 6 is to quit the drawing loop. Use a while loop to check to make sure that the user’s answer is in the correct range of value, 1 – 6. If it is not, loop back and ask the user to re-enter their choice. As long as the selection is not 6, present another menu and ask the user to select the picture to be drawn. There will be 3 pictures, a 1) smiling face, 2) a pyramid and 3) a picture of your choice. Check the input validity of that selection using a do while loop. Use a switch structure to process the picture selection. Declare any variables you will use in the drawings up above the switch statement. In each case statement, set the color using: SetConsoleTextAttribute(screen, colorChoice); Be sure to adjust the colorChoice value so that you will be drawing with the bright version of the color. It shows up so much better! Each picture will be drawn using the color selected by the user. Each picture can be drawn using symbols of your choice. When the user selects 6 for the color selection, drop out of the loop, and show a good-bye message.
Write a C++
In main, begin by declaring variables and displaying the class header, and cout an explanation of the program. You may include this in your header or make it separate.
You will need constants for the maximum color number and for the maximum picture number as well as a value that indicates the user has finished drawing.
These will look like:
//Constants
const int QUIT{ 6};
const int MAX_COLORS{ 6};
const int MAX_PIX{3};
At this point, create a handle to the standard output device (the console) using:
HANDLE screen = GetStdHandle(STD_OUTPUT_HANDLE);
You will use this handle to access the screen to change colors. You will also need to #include <windows.h>.
Open a do-while loop. This is the “play loop.” Provide a menu of colors and ask the user to select a color for the drawing. There will be 6 menu items, 5 colors, blue, green, cyan, red and purple. These will be selections 1-5. Selection 6 is to quit the drawing loop.
Use a while loop to check to make sure that the user’s answer is in the correct range of value, 1 – 6. If it is not, loop back and ask the user to re-enter their choice.
As long as the selection is not 6, present another menu and ask the user to select the picture to be drawn. There will be 3 pictures, a 1) smiling face, 2) a pyramid and 3) a picture of your choice. Check the input validity of that selection using a do while loop.
Use a switch structure to process the picture selection. Declare any variables you will use in the drawings up above the switch statement.
In each case statement, set the color using:
SetConsoleTextAttribute(screen, colorChoice);
Be sure to adjust the colorChoice value so that you will be drawing with the bright version of the color. It shows up so much better! Each picture will be drawn using the color selected by the user. Each picture can be drawn using symbols of your choice.
When the user selects 6 for the color selection, drop out of the loop, and show a good-bye message.

C++ program:
#include <iostream>
#include <windows.h>
// Constants
const int QUIT{6};
const int MAX_COLORS{6};
const int MAX_PIX{3};
// Function prototypes
void showMenuColors();
void showMenuPix();
void drawSmilingFace(HANDLE screen, int colorChoice);
void drawPyramid(HANDLE screen, int colorChoice);
void drawMyChoice(HANDLE screen, int colorChoice);
int main()
{
// Declare variables
int colorChoice, pictureChoice;
HANDLE screen = GetStdHandle(STD_OUTPUT_HANDLE);
// Display program header and explanation
std::cout << "CIS247A Week 3 Lab B\n"
<< "Programmer: YOUR NAME\n"
<< "This program allows the user to select a color and a shape to draw.\n"
<< "The user can select from 5 colors (blue, green, cyan, red, and purple)\n"
<< "and 3 shapes (smiling face, pyramid, and a picture of your choice).\n\n";
// Play loop
do
{
// Show menu of colors
showMenuColors();
// Get color choice from user
std::cout << "Enter your color choice (1-6): ";
std::cin >> colorChoice;
// Check if color choice is valid
while (colorChoice < 1 || colorChoice > MAX_COLORS)
{
std::cout << "Invalid choice. Enter your color choice (1-6): ";
std::cin >> colorChoice;
}
// Quit if color choice is 6
if (colorChoice == QUIT)
{
break;
}
// Show menu of pictures
showMenuPix();
// Get picture choice from user
std::cout << "Enter your picture choice (1-3): ";
std::cin >> pictureChoice;
// Check if picture choice is valid
while (pictureChoice < 1 || pictureChoice > MAX_PIX)
{
std::cout << "Invalid choice. Enter your picture choice (1-3): ";
std::cin >> pictureChoice;
}
// Process picture choice
switch (pictureChoice )
{
case 1:
drawSmilingFace(screen, colorChoice);
break;
case 2:
drawPyramid(screen, colorChoice);
break;
case 3:
drawMyChoice(screen, colorChoice);
break;
}
} while (colorChoice != QUIT);
// Display goodbye message
std::cout << "Goodbye!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

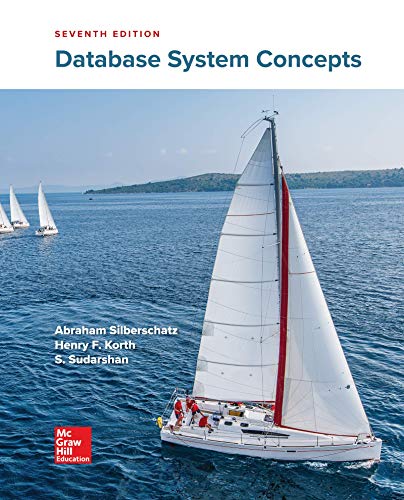
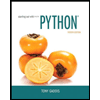
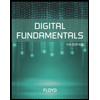
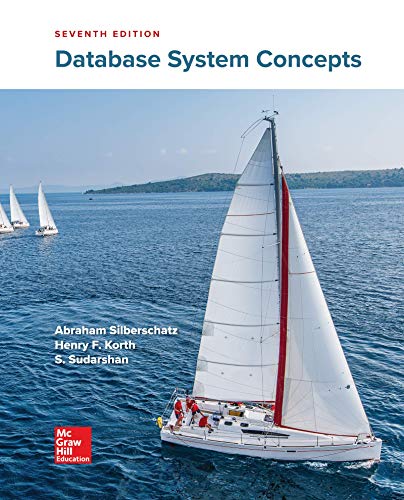
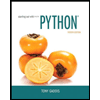
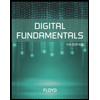
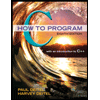
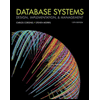
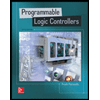