BACKGROUND GDOT has contacted you to help write code in C++ to control the railroad signals (barriers with flashing lights and sounds across railroad tracks) in Georgia. You must create a Railroad Signal class with three hidden attributes (Up, Down and Signal), two constructors (a default that sets Up to on, Down and signal to off, and an overloaded that sets all Up to off, Down and Signal to on) and a method that changes the position and activates or deactivates the Signal. Here is the algorithm for the given C++ code: #include using namespace std; class RailroadSignal { private: bool Up; bool Down; bool Signal; public: // Default constructor that sets Up to on, Down and Signal to off RailroadSignal() : Up(true), Down(false), Signal(false) {} // Overloaded constructor that sets all Up to off, Down and Signal to on RailroadSignal(bool up, bool down, bool signal) : Up(up), Down(down), Signal(signal) {} // Method to change the position and activate or deactivate the Signal void changePosition(bool up, bool down, bool signal) { Up = up; Down = down; Signal = signal; // Code to control the railroad signals in Georgia based on the values of Up, Down, and Signal if (Up && Down && Signal) { cout << "Railroad signals are up, down, and signal is on." << endl; } else if (Up && Down && !Signal) { cout << "Railroad signals are up and down, and signal is off." << endl; } else { cout << "Invalid position for railroad signals." << endl; } } }; int main() { // Create a default instance of RailroadSignal RailroadSignal signal1; signal1.changePosition(true, false, true); // Change position of signal1 // Create an overloaded instance of RailroadSignal RailroadSignal signal2(false, true, true); signal2.changePosition(false, true, false); // Change position of signal2 return 0; } Question: You must create (in C++) two Railroad Signal objects from the class you just created (above) to prove that your class works properly for GDOT, the first object should use the default constructor and the second object should use the overloaded constructor.
BACKGROUND
GDOT has contacted you to help write code in C++ to control the railroad signals (barriers with flashing lights and sounds across railroad tracks) in Georgia. You must create a Railroad Signal class with three hidden attributes (Up, Down and Signal), two constructors (a default that sets Up to on, Down and signal to off, and an overloaded that sets all Up to off, Down and Signal to on) and a method that changes the position and activates or deactivates the Signal.
Here is the
#include <iostream>
using namespace std;
class RailroadSignal {
private:
bool Up;
bool Down;
bool Signal;
public:
// Default constructor that sets Up to on, Down and Signal to off
RailroadSignal() : Up(true), Down(false), Signal(false) {}
// Overloaded constructor that sets all Up to off, Down and Signal to on
RailroadSignal(bool up, bool down, bool signal) : Up(up), Down(down), Signal(signal) {}
// Method to change the position and activate or deactivate the Signal
void changePosition(bool up, bool down, bool signal) {
Up = up;
Down = down;
Signal = signal;
// Code to control the railroad signals in Georgia based on the values of Up, Down, and Signal
if (Up && Down && Signal) {
cout << "Railroad signals are up, down, and signal is on." << endl;
} else if (Up && Down && !Signal) {
cout << "Railroad signals are up and down, and signal is off." << endl;
} else {
cout << "Invalid position for railroad signals." << endl;
}
}
};
int main() {
// Create a default instance of RailroadSignal
RailroadSignal signal1;
signal1.changePosition(true, false, true); // Change position of signal1
// Create an overloaded instance of RailroadSignal
RailroadSignal signal2(false, true, true);
signal2.changePosition(false, true, false); // Change position of signal2
return 0;
}
Question:
You must create (in C++) two Railroad Signal objects from the class you just created (above) to prove that your class works properly for GDOT, the first object should use the default constructor and the second object should use the overloaded constructor.

Step by step
Solved in 2 steps with 1 images

Using the objects you just created above (previous question), call the method to change the lights and arms to the next in the sequence (in C++).
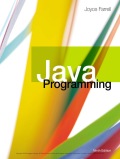
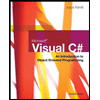
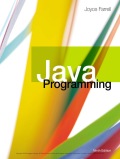
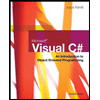