Using C programming language write a program that simulates a variant of the Tiny Machine Architecture. In this implementation memory (RAM) is split into Instruction Memory (IM) and Data Memory (DM). Your code must implement the basic instruction set architecture (ISA) of the Tiny Machine Architecture: 1 -> LOAD 2 -> ADD 3 -> STORE 4 -> SUB 5 -> IN 6 -> OUT 7 -> END 8 -> JMP 9 -> SKIPZ Each piece of the architecture must be accurately represented in your code (Instruction Register, Program Counter, Memory Address Registers, Instruction Memory, Data Memory, Memory Data Registers, and Accumulator). Data Memory will be represented by an integer array. Your Program Counter will begin pointing to the first instruction of the program. Input Specifications Your simulator must run from the command line with a single input file as a parameter to main. This file will contain a sequence of instructions for your simulator to store in “Instruction Memory” and then run via the fetch/execute cycle. In the input file each instruction is represented with two integers: the first one represents the opcode and the second one a memory address or a device number depending on the instruction. Example: Input File 5 5 //IN 5 6 7 //OUT 7 3 0 //STORE 0 5 5 //IN 5 6 7 //OUT 7 3 1 //STORE 1 1 0 //LOAD 0 4 1 //SUB 1 3 0 //STORE 0 6 7 //OUT 7 1 1 //LOAD 1 6 7 //OUT 7 7 0 //END Output Specifications Your simulator should provide output according to the input file. Along with this output your program should provide status messages identifying details on the workings of your simulator. Output text does not have to reflect my example word-for-word, but please provide detail on the program as it runs in a readable format that does not conflict with the actual output of your simulator. After each instruction print the current state of the Program Counter, Accumulator, and Data Memory. The INPUT instruction is the only one that should prompt an interaction from the user. Example: Assembling Program... Program Assembled. Run. PC = 10 | A = NULL | DM = [0, 0, 0, 0, 0, 0, 0, 0, 0, 0] /* input value */ X PC = 11 | A = X | DM = [0, 0, 0, 0, 0, 0, 0, 0, 0, 0] /* outputting accumulator to screen */ X PC = 12 | A = X | DM = [0, 0, 0, 0, 0, 0, 0, 0, 0, 0] /* storing accumulator to memory location 0 */ PC = 13 | A = X | DM = [X, 0, 0, 0, 0, 0, 0, 0, 0, 0] ... etc Program complete.
Using C
Architecture. In this implementation memory (RAM) is split into Instruction Memory (IM) and Data
Memory (DM). Your code must implement the basic instruction set architecture (ISA) of the Tiny
Machine Architecture:
1 -> LOAD
2 -> ADD
3 -> STORE
4 -> SUB
5 -> IN
6 -> OUT
7 -> END
8 -> JMP
9 -> SKIPZ
Each piece of the architecture must be accurately represented in your code (Instruction Register, Program
Counter, Memory Address Registers, Instruction Memory, Data Memory, Memory Data Registers, and
Accumulator). Data Memory will be represented by an integer array. Your Program Counter will begin
pointing to the first instruction of the program.
Your simulator must run from the command line with a single input file as a parameter to main. This file
will contain a sequence of instructions for your simulator to store in “Instruction Memory” and then run
via the fetch/execute cycle. In the input file each instruction is represented with two integers: the first one
represents the opcode and the second one a memory address or a device number depending on the
instruction.
Example:
Input File
5 5 //IN 5
6 7 //OUT 7
3 0 //STORE 0
5 5 //IN 5
6 7 //OUT 7
3 1 //STORE 1
1 0 //LOAD 0
4 1 //SUB 1
3 0 //STORE 0
6 7 //OUT 7
1 1 //LOAD 1
6 7 //OUT 7
7 0 //END
Output Specifications
Your simulator should provide output according to the input file. Along with this output your program
should provide status messages identifying details on the workings of your simulator. Output text does
not have to reflect my example word-for-word, but please provide detail on the program as it runs in a
readable format that does not conflict with the actual output of your simulator. After each instruction print
the current state of the Program Counter, Accumulator, and Data Memory. The INPUT instruction is the
only one that should prompt an interaction from the user.
Example:
Assembling Program...
Program Assembled.
Run.
PC = 10 | A = NULL | DM = [0, 0, 0, 0, 0, 0, 0, 0, 0, 0]
/* input value */
X
PC = 11 | A = X | DM = [0, 0, 0, 0, 0, 0, 0, 0, 0, 0]
X
PC = 12 | A = X | DM = [0, 0, 0, 0, 0, 0, 0, 0, 0, 0]
/* storing accumulator to memory location 0 */
PC = 13 | A = X | DM = [X, 0, 0, 0, 0, 0, 0, 0, 0, 0]
... etc
Program complete.

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

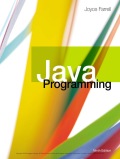
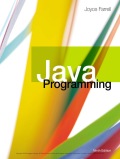