Use the classes Show, Movie, Series, and Platform that you implemented in Lab8, and perform the following modifications which are shown in this UML diagram: + updateRate():double Platform - shows: ArrayList + Platform() + addShow(): void + searchShows(): void <> Ratable + printMovies(): void + printSeries(): void + updateRate():double + printTop100): void + watchAndUpdate(): void - duration: double - production Year: int Movie Show + name: String - times Watched: int - rate: double -maxRate: int = 5 - date Added: java.util.Date + Show() + Show(name:String) + getRate(): double + getTimes Watched():int + getMaxRate():int + getDate Added(): java.util.Date + updateRate():double + toString(): String + equals(o: Object): boolean + compareTo(o: Show):int + getDuration(): double + Movie() + Movie(name:String, duration:double, production Year: int) + getDuration(): double + getProduction Year(): int + toString(): String + equals(o: Object): boolean <> java.lang.Comparable +compareTo(o:Show): int numOfEpisodes: int episodeDuration: double Series + Series() + Series (name:String, numOfEpisodes:int, episodeDuration: double) + getNumOfEpisodes(): int +getEpisodeDuration(): double + toString():String + getDuration(): double
Please help solve this with java i posted it before and got rejected :(
Modify the Show class such that it implements the Comparable interface of java.lang. Remember to
add the concrete type Show between <> when you implement Comparable. Note the syntax error that
occurs and understand why it occurs.
2. Implement (override) the compareTo method in the Show class such that it compares the shows
according to their rates: returns 1 if the first show’s rate is greater than the second, -1if the first show’s
rate is less than the second, and 0 if they are equal. (First show is “this”, second show is o)
3. Define an interface named Ratable, that has the method updateRate as shown in the UML diagram, in
a file named Ratable.java.
4. Modify the Show class such that it implements the Ratable interface. Note the syntax error that occurs
in the updateRate method and understand why it occurs.
5. Modify the updateRate method in the Show class such that it returns a double value that represents the
updated rate.
6. Modify the Platform class such that it implements the Ratable interface. Note the syntax error that
occurs and understand why it occurs.
7. Implement (override) the updateRate method in the Platform class such that it returns the average of
the rates of all shows in the platform.
8. Define the abstract method getDuration() in the Show class as shown in the UML diagram. Note the
syntax error that occurs and understand why it occurs.
9. Re-define the Show class to be abstract. Does this solve the syntax error that occurred in the previous
step? Note the syntax error that occurs now in the Series class and understand why it occurs. Why
doesn’t the same error occur in the Movie class.
10. Implement (override) the getDuration method in the Series class such that it returns the multiplication
of the numOfEpisodes by the episodeDuration.
11. In the Platform class, implement the method printTop10 which prints the top 10 shows in the platform
according to their rates in a message dialog box. If the number of shows in the platform is less than or
equals 10, it must print names and rates of the available shows ordered according to their rates from
the highest rated to the least rated each on a line. If the number of shows in the platform is greater than
10, names and rates of the ten shows with the highest rates must be printed from the highest rated to
the least rated each on a line. This method must use the sort method of the Arrays class.
12. Modify the searchShows method such that it asks the user to enter the name of the show he wants to
search for or part of it. The search must be performed to find all the shows whose names contain the
entered String. Names (only) of these shows must be displayed in a message dialog box each on a new
line.
13. Add the method watchAndRate to the Platform class as shown in the UML diagram. The method must
ask the user to enter the name of the show he wants to watch, search for the show (assume that the user
may not enter the name with case sensitivity), and invoke the updateRate method for that show. If no
show with the entered name is found, you must print the following message in a message dialog box
“Sorry! Could not find show with title: ….”.
14. Add the two options: Watch and Rate, Show Top10 to the options provided by the menu in the main
method. For each option invoke the appropriate method. Your menu must look like the one below:


Step by step
Solved in 7 steps with 3 images

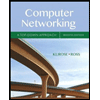
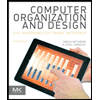
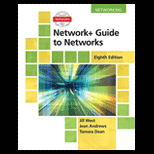
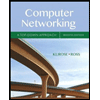
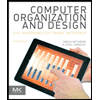
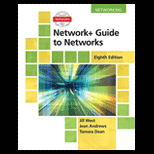
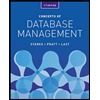
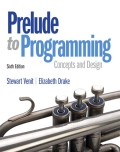
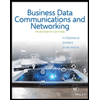