This week, make sure you add functionality to keep statistics on the number of correct answers vs. the total number of problems attempted if you haven't already done so. Then, alter the code to allow for floating-point numbers. This requires formatting the output. #include
This week, make sure you add functionality to keep statistics on the number of correct answers vs. the total number of problems attempted if you haven't already done so. Then, alter the code to allow for floating-point numbers. This requires formatting the output. #include
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
This week, make sure you add functionality to keep statistics on the number of correct answers vs. the total number of problems attempted if you haven't already done so.
Then, alter the code to allow for floating-point numbers. This requires formatting the output.
#include<stdio.h>
#include<stdlib.h>
#include<time.h>
int Menu ();
int Addition (int x, int y);
int Subtraction (int x, int y);
int Multiplication (int x, int y);
int Division (int x, int y);
int valid (char x);
int
main ()
{
int option;
srand (time (NULL));
/* total number of questions and total correct answers */
int ttlProblems = 0, correctAnswers = 0;
option = Menu ();
while (option != 5)
{
/* taking input the difficultyLevel */
char difficultyLevel;
printf ("Enter difficultyLevel of question(e,m,h): ");
getchar (); // to avoid the new line
scanf ("%c", &difficultyLevel);
if (valid (difficultyLevel) == 0)
{
printf ("Wrong difficultyLevel!\n");
continue;// if invalid input then take the input again.
}
/* if input is valid then generate the operand */
int num1, num2;
if (difficultyLevel == 'E' || difficultyLevel == 'e')
{
num1 = rand () % 10 + 1;
num2 = rand () % 10 + 1;
}
else if (difficultyLevel == 'M' || difficultyLevel == 'm')
{
num1 = rand () % 100 + 1;
num2 = rand () % 100 + 1;
}
else
{
num1 = rand () % 1000 + 1;
num2 = rand () % 1000 + 1;
}
/* do corresponding calculation */
switch (option)
{
case 1:
correctAnswers += Addition (num1, num2);// Addition function return 1 for correct answer otherwise 0.
ttlProblems++;//increasing the total questions count by 1.
break;
case 2:
correctAnswers += Subtraction (num1, num2);// Substraction function return 1 for correct answer otherwise 0.
ttlProblems++;
break;
case 3:
correctAnswers += Multiplication (num1, num2);// Multiplication function return 1 for correct answer otherwise 0.
ttlProblems++;
break;
case 4:
correctAnswers += Division (num1, num2);// Division function return 1 for correct answer otherwise 0.
ttlProblems++;
break;
default:
printf ("Choose a valid option!\n");// not a valid option.
break;
}
option = Menu ();
}
/* Printing the total question attemped and total correct answers at the end */
printf ("Total number of problems attempted: %d\n", ttlProblems);
printf ("Total number of correct answers: %d\n", correctAnswers);
}
/* this function prints the menu and take one option as input and return the input taken. */
int
Menu ()
{
int option;
puts ("Math Practice Program");
puts ("1. Addition");
puts ("2. Subtraction");
puts ("3. Multiplication");
puts ("4. Division");
puts ("5. Quit");
printf ("Enter an option ");
scanf ("%d", &option);
return option;
}
/* This function validates that the difficultyLevel provided is valid or not. */
int
valid (char x)
{
if (x == 'm' || x == 'M' || x == 'e' || x == 'E' || x == 'h' || x == 'H')
return 1;
else
return 0;
}
/* This function is for addition
it returns 1 if the answer is correct
otherwise return 0;
*/
int
Addition (int x, int y)
{
int answer, answer1;
answer1 = x + y;
int times = 1;
while (times <= 3)
{
printf ("\n%d + %d = ?...\n", x, y);
printf ("Enter answer:");
scanf ("%d", &answer);
if (answer == answer1)
{
printf ("\nCorrect!\n\n");
return 1;
}
else
{
printf ("Incorrect!\n\n");
if (times < 3)
printf ("Sorry...try again with a different answer.\n");
}
times++;
}
return 0;
}
int
Subtraction (int x, int y)
{
int answer, answer1;
answer1 = x - y;
int times = 1;
while (times <= 3)
{
printf ("\n%d - %d = ?...\n", x, y);
printf ("Enter answer:");
scanf ("%d", &answer);
if (answer == answer1)
{
printf ("\nCorrect!\n\n");
return 1;// return 1 for correct answer
}
else
{
printf ("Incorrect!\n\n");
if (times < 3)
printf ("Sorry...try again with a different answer.\n");
}
times++;
}
return 0;
}
int
Multiplication (int x, int y)
{
int answer, answer1;
answer1 = x * y;
int times = 1;
while (times <= 3) // user can attempt upto three times.
{
printf ("\n%d * %d = ?...\n", x, y);
printf ("Enter answer:");
scanf ("%d", &answer);
if (answer == answer1)
{
printf ("\nCorrect!\n\n");
return 1;
}
else
{
printf ("Incorrect!\n\n");
if (times < 3)
printf ("Sorry...try again with a different answer.\n");
}
times++;
}
return 0;
}
int
Division (int num1, int num2)
{
int remainder, remainder1, answer, answer1;
answer1 = num1 / num2;
remainder1 = num1 % num2;
int times = 1;
while (times <= 3)
{
printf ("\n%d / %d = ?...\n", num1, num2); // printing the question
printf ("Enter quotient:");
scanf ("%d", &answer);
printf ("Enter remainder:");
scanf ("%d", &remainder);
if (answer == answer1 && remainder == remainder1)
{
printf ("\nCorrect!\n\n");
return 1;// return 1 for correct answers
}
else
{
printf ("Incorrect!\n\n");
if (times < 3)
printf ("Sorry...try again with a different answer.\n");
}
times++;
}
return 0;
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

Recommended textbooks for you
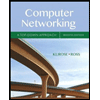
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
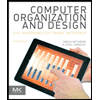
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
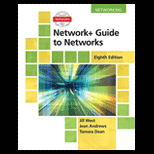
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
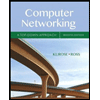
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
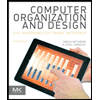
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
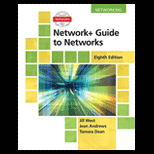
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
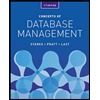
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
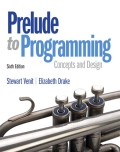
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
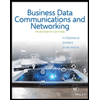
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY