this is the "index page "code index .hbs Total Balance Deposit Deposit Withdraw Withdraw // add label for displaying user name 2.js const acctBalanceLbl = document.getElementById("acctBalanceLbl"); const deposits = []; const withdrawals = []; let totalBalance = 25; const userDeposit = document.getElementById("userDeposit"); const btnDeposit = document.getElementById("btnDeposit"); const userWithdraw = document.getElementById("userWithdraw"); const btnWithdraw = document.getElementById("btnWithdraw"); // Create our number formatter. const formatter = new Intl.NumberFormat('en-US', { style: 'currency', currency: 'USD', minimumFractionDigits: 2, /* the default value for minimumFractionDigits depends on the currency and is usually already 2 */ }); // accept deposits from user, store deposits in array btnDeposit.addEventListener('click', () => { // checks if deposit is a number if (isNaN(userDeposit.value)) { alert("Please enter a number."); return userDeposit.value = ''; } else { // checks if deposit meets parameters if (userDeposit.value < 0.01 || userDeposit.value > 10000) { alert("You can only deposit between $0.01 and $10,000.") return userDeposit.value = ''; } else { // push deposit to array deposits.push(Number(userDeposit.value)); // calculate Total Balance totalBalance += (Number(userDeposit.value)); // format TotalBalance to show $ XX.XX (2 decimal places) let totalBalanceFormatted = formatter.format(totalBalance); document.getElementById("acctBalanceLbl").innerHTML = totalBalanceFormatted; // print deposit to console to verify success console.log("$" + userDeposit.value); return userDeposit.value = ''; } } }); // accept withdrawals from user, store withdrawals in array btnWithdraw.addEventListener('click', () => { // checks if withdrawal is a number if (isNaN(userWithdraw.value)) { alert("Please enter a number."); return userWithdraw.value = ''; } else { // checks if withdrawal meets parameters if (userWithdraw.value > totalBalance - 5) { alert("Your total balance cannot drop below $5."); return userWithdraw.value = ''; } else { // push withdrawal to array withdrawals.push(Number(userWithdraw.value)); // calculate Total Balance totalBalance -= (Number(userWithdraw.value)); // format TotalBalance to show $ XX.XX (2 decimal places) let totalBalanceFormatted = formatter.format(totalBalance); document.getElementById("acctBalanceLbl").innerHTML = totalBalanceFormatted; // print withdrawal to console to verfify success console.log("$" + userWithdraw.value); return userWithdraw.value = ''; } } }); // format TotalBalance to show $ XX.XX (2 decimal places) let totalBalanceFormatted = formatter.format(totalBalance); document.getElementById("acctBalanceLbl").innerHTML = totalBalanceFormatted; ------------------------------ I use the "login "page to go to the index page ,and the index page shows like this . how could i add a code that shows the username on the right corner shows the username , put mouse on it will shows "logout:click logout will go back to the login page .
this is the "index page "code
index .hbs
<!DOCTYPE html>
<html>
<head>
<link rel="stylesheet" href="./data/l.css">
</head>
<body>
<div class="flex">
<div class="element-center">
<div id="acctBalance">
<span class="wrap"></span>
</a>
<h1>
Total Balance
</h1>
<label id="acctBalanceLbl"></label>
<input id="txtid" name="txtid" type="text" maxlength="10" placeholder="Accountnumber"><br><br>
</div>
<div id="inputs">
<h2>
Deposit
</h2>
<input type="text" id="userDeposit" required>
<button id="btnDeposit">Deposit</button>
<h2>
Withdraw
</h2>
<input type="text" id="userWithdraw" required>
<button id="btnWithdraw">Withdraw</button>
<label id="usernameLb2"></label>// add label for displaying user name
</div>
</div>
</div>
<script type="text/javascript" src="bank_account_script.js"></script>
<script type="text/javascript" src="typewriter_script.js"></script>
<script src="./data/2.js."></script>
</body>
</html>
2.js
const acctBalanceLbl = document.getElementById("acctBalanceLbl");
const deposits = [];
const withdrawals = [];
let totalBalance = 25;
const userDeposit = document.getElementById("userDeposit");
const btnDeposit = document.getElementById("btnDeposit");
const userWithdraw = document.getElementById("userWithdraw");
const btnWithdraw = document.getElementById("btnWithdraw");
// Create our number formatter.
const formatter = new Intl.NumberFormat('en-US', {
style: 'currency',
currency: 'USD',
minimumFractionDigits: 2,
/*
the default value for minimumFractionDigits depends on the currency
and is usually already 2
*/
});
// accept deposits from user, store deposits in array
btnDeposit.addEventListener('click', () => {
// checks if deposit is a number
if (isNaN(userDeposit.value)) {
alert("Please enter a number.");
return userDeposit.value = '';
} else {
// checks if deposit meets parameters
if (userDeposit.value < 0.01 || userDeposit.value > 10000) {
alert("You can only deposit between $0.01 and $10,000.")
return userDeposit.value = '';
} else {
// push deposit to array
deposits.push(Number(userDeposit.value));
// calculate Total Balance
totalBalance += (Number(userDeposit.value));
// format TotalBalance to show $ XX.XX (2 decimal places)
let totalBalanceFormatted = formatter.format(totalBalance);
document.getElementById("acctBalanceLbl").innerHTML = totalBalanceFormatted;
// print deposit to console to verify success
console.log("$" + userDeposit.value);
return userDeposit.value = '';
}
}
});
// accept withdrawals from user, store withdrawals in array
btnWithdraw.addEventListener('click', () => {
// checks if withdrawal is a number
if (isNaN(userWithdraw.value)) {
alert("Please enter a number.");
return userWithdraw.value = '';
} else {
// checks if withdrawal meets parameters
if (userWithdraw.value > totalBalance - 5) {
alert("Your total balance cannot drop below $5.");
return userWithdraw.value = '';
} else {
// push withdrawal to array
withdrawals.push(Number(userWithdraw.value));
// calculate Total Balance
totalBalance -= (Number(userWithdraw.value));
// format TotalBalance to show $ XX.XX (2 decimal places)
let totalBalanceFormatted = formatter.format(totalBalance);
document.getElementById("acctBalanceLbl").innerHTML = totalBalanceFormatted;
// print withdrawal to console to verfify success
console.log("$" + userWithdraw.value);
return userWithdraw.value = '';
}
}
});
// format TotalBalance to show $ XX.XX (2 decimal places)
let totalBalanceFormatted = formatter.format(totalBalance);
document.getElementById("acctBalanceLbl").innerHTML = totalBalanceFormatted;
------------------------------
I use the "login "page to go to the index page ,and the index page shows like this
.
how could i add a code that shows the username on the right corner shows the username , put mouse on it will shows "logout:click logout will go back to the login page .


Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

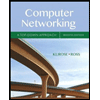
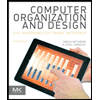
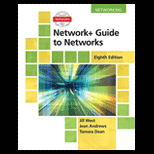
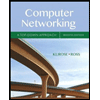
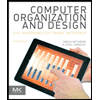
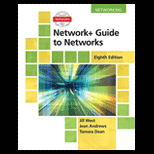
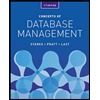
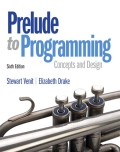
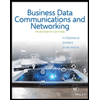