This application must be able to handle creating new entries in a proprietary database as well as returning searches for items in the current database. You MUST implement the following: Start the program by displaying the title of your program (you come up with a name) Have a primary prompt that allows you to enter commands (e.g. “Enter command >” ) Interpret primary commands and move to secondary states based on the commands entered. Commands you must support at the primary prompt are: a.) new entry b.) search by actor c.) search by year d.) search by runtime (in minutes) e.) search by director f.) search by title g.) quit Your secondary states must do the following: For “new entry” → Prompts to do the following: Enter title > Enter year > Enter runtime (minutes) > Enter actor 1 > Enter actor 2 > Enter Director > You must add this entry into the database in one-line (use delimiters between tokens; come up with your own strategy for token order but BE CONSISTENT!) After entry, return to the main command state... For “search by actor” → Prompts to: Enter actor > Once actor is entered, search database (hopefully already in memory.) Display a list of movie titles that the actor has been in. This must display the titles of movies actor has been in regardless of if actor was actor 1 or actor 2. (If nothing found, display “No titles found for actor” and return to main prompt.) After entry, return to the main command state... For “search by year” → Prompt to: Enter year > Once year is entered, search database and return list of titles made in that year. (If nothing found, display “No titles found for year” and return to main prompt.) After entry, return to the main command state... For “search by runtime” → Prompts to: Enter runtime (minutes) > Search like how you did for year. Display list and return to main prompt. If nothing found, display a prompt saying so and return to main prompt. For “search by director” → Prompts to: Enter director > After entry, return to the main command state... For “search by title” → Prompts to: Enter title > If the title is found, return the following about title: Actors: actor1, actor 2 Director: director name Year: year made Runtime: x minutes if title not found, post message saying so and return to main prompt. After entry, return to the main command state. For “quit” → This simply exits the program (close out any open I / O streams prior to exit!) Other Requirements: File I/O class (for saving / loading the database) You can make these two classes; one for read / one for write if you want. Keyboard input class (handles declaring Scanner object, retrieving text input, closing object) Class representing a single entry. Use this to easily pass entry information around as a parameter. This class must contain private fields for: title, actor1, actor2, year, runtime, director Also, use get methods (public) to retrieve data from fields Have a class that extends the functionality of the single-entry class and holds the whole current database as a class object. (Hint: You can use an ArrayList behind-the-scenes to do this.) Create get method that can lookup single entry based on certain criteria (index number, search by criteria, etc.) You can overload your get method if you wis
Make a Movie
Project Specifications
Background
This application must be able to handle creating new entries in a proprietary database as well as returning searches for items in the current database.
You MUST implement the following:
- Start the program by displaying the title of your program (you come up with a name)
- Have a primary prompt that allows you to enter commands
(e.g. “Enter command >” )
- Interpret primary commands and move to secondary states based on the commands entered. Commands you must support at the primary prompt are:
a.) new entry
b.) search by actor
c.) search by year
d.) search by runtime (in minutes)
e.) search by director
f.) search by title
g.) quit
- Your secondary states must do the following:
For “new entry” → Prompts to do the following:
Enter title >
Enter year >
Enter runtime (minutes) >
Enter actor 1 >
Enter actor 2 >
Enter Director >
You must add this entry into the database in one-line (use delimiters between tokens; come up with your own strategy for token order but BE CONSISTENT!)
- After entry, return to the main command state...
For “search by actor” → Prompts to:
Enter actor >
Once actor is entered, search database (hopefully already in memory.) Display a list of movie titles that the actor has been in. This must display the titles of movies actor has been in regardless of if actor was actor 1 or actor 2. (If nothing found, display “No titles found for actor” and return to main prompt.)
- After entry, return to the main command state...
For “search by year” → Prompt to:
Enter year >
Once year is entered, search database and return list of titles made in that year. (If nothing found, display “No titles found for year” and return to main prompt.)
- After entry, return to the main command state...
For “search by runtime” → Prompts to:
Enter runtime (minutes) >
Search like how you did for year. Display list and return to main prompt. If nothing found, display a prompt saying so and return to main prompt.
For “search by director” → Prompts to:
Enter director >
- After entry, return to the main command state...
For “search by title” → Prompts to:
Enter title >
If the title is found, return the following about title:
Actors: actor1, actor 2
Director: director name
Year: year made
Runtime: x minutes
if title not found, post message saying so and return to main prompt.
- After entry, return to the main command state.
For “quit” → This simply exits the program (close out any open I / O streams prior to exit!)
Other Requirements:
- File I/O class (for saving / loading the database) You can make these two classes; one for read / one for write if you want.
- Keyboard input class (handles declaring Scanner object, retrieving text input, closing object)
- Class representing a single entry. Use this to easily pass entry information around as a parameter. This class must contain private fields for:
- title, actor1, actor2, year, runtime, director
- Also, use get methods (public) to retrieve data from fields
- Have a class that extends the functionality of the single-entry class and holds the whole current database as a class object. (Hint: You can use an ArrayList behind-the-scenes to do this.)
- Create get method that can lookup single entry based on certain criteria (index number, search by criteria, etc.) You can overload your get method if you wish.
Classes to use in images.



Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 3 images

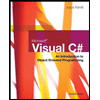
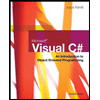