The user needs a program to interact with employee records. The kinds of interactions are "Add an Employee" (Create record), "Show All Employees" (Read records), "View an Employee" (Read one record), "Update an Employee" (Update or change a record), and "Delete an Employee" (Delete a record). These interactions form the standard data processes in much of business software Create, Read, Update, and Delete, which are known by the acronym CRUD. The user needs to continue working with records until they are ready to tell the program. To do this, the program will present a menu of CRUD options and an option to Quit. The user will choose an option and the program will react accordingly. For this lab, the program will only print out which option the user chose. In later labs, we will add more functionality. Questions: What are the inputs needed to solve this programming problem (names and data types)? What are the desired outputs (data types and descriptions)? Since this is a menu of options, what do we do if the user enters something not on our list? What are the formats for the outputs?
Follow the instructions from your instructor to accomplish the following in-class lab assignment.
The Problem to Solve:
The user needs a program to interact with employee records. The kinds of interactions are "Add an Employee" (Create record), "Show All Employees" (Read records), "View an Employee" (Read one record), "Update an Employee" (Update or change a record), and "Delete an Employee" (Delete a record). These interactions form the standard data processes in much of business software Create, Read, Update, and Delete, which are known by the acronym CRUD.
The user needs to continue working with records until they are ready to tell the program. To do this, the program will present a menu of CRUD options and an option to Quit. The user will choose an option and the program will react accordingly.
For this lab, the program will only print out which option the user chose. In later labs, we will add more functionality.
Questions:
- What are the inputs needed to solve this
programming problem (names and data types)? - What are the desired outputs (data types and descriptions)?
- Since this is a menu of options, what do we do if the user enters something not on our list?
- What are the formats for the outputs?
The Python Program
- Create an new Python file called employee_program.py. Open the file in your Python editor environment. Copy your Python program template into this new file.
- In the file, change the program title to "EMPLOYEE PROGRAM". Save and run the file to make sure that it displays the title and the Program Complete message. It is always a good idea to run your incomplete program whenever it gets to a runnable state so you can fix errors as early as possible.
- Print the menu title and the menu options. Note that there is a tab escape sequence at the beginning of each of the menu lines.
- "\t-- Main Menu --"
- "\t1) Add an Employee"
- "\t2) Show All Employees"
- "\t3) View an Employee"
- "\t4) Update an Employee"
- "\t5) Delete an Employee"
- "\tQ) Quit"
- Get the choice from the user and save the string to a variable called user_choice
- Build the decision structure where each if or elif checks if the user_choice is equal to the strings 1, 2, 3, 4, 5, Q, or q. For each match, print a message stating that the user chose to -- and the name of the task. This is the part that will be changed drastically in future programs.
- Add an else clause to the decision structure to handle the user entering something that was not on the list. For the body of the else, print a message stating that the choice was not recognized and ask that the user try again.
- Add an input statement asking the user to "Press the Enter key to continue... ". There is no need to store this input() to a variable because we are only interested in whether the user pressed Enter.
- Print that the program is complete
Questions:
- What are the inputs needed to solve this programming problem (names and data types)?
- What are the desired outputs (data types and descriptions)?
- What are the formats for the outputs?
The Python Program
Start with your program from last week's lab called employee_program.py. If that program did not work, it is going to be a lot harder to move forward.
Note that is is often better to make a copy of your program before making major changes. That way, if something goes terribly wrong, you can delete the messed up version and go back to the original to start all over. Professional programmers use version control software such as git or Subversion to handle these sorts of tasks.
- Open your employee_program.py file in your editor.
- In the main() function, after the code that shows the program title, create a variable called "done" and set it to False.
- Under the variable done, create a variable called "prompt" and set it equal to "Your choice: "
- Under this, start the while statement with the condition "not done" -- remember the colon at the end
- Highlight all of the code below the while statement and above the "Program Complete" part and tab that in one tab. This will move the code so it is considered part of the code block of the while loop
- In the if-elif-else structure, find the elif for the option Q. Under the print() statement, add a line that says done = True. This changes the loop variable to end the while loop.
- Save your file and run the program. Try different menu options and a few items that are no on the menu. Except for when you enter Q or q, the program should display a message about which item you chose, ask you to press Enter to continue, and then display the menu again.

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

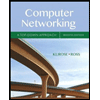
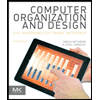
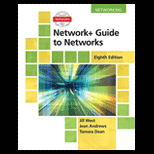
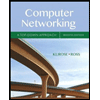
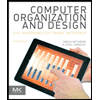
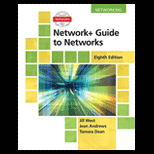
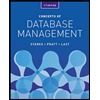
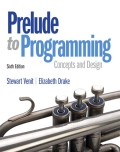
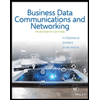