The program initializes a vector with an assigned capacity and a random number for each element. The program counts the histogram of all of the contents of the vector ranging from 1 to 5 and displays it on the screen. The program is also getting the sum of all of the vectors and displays it afterwards. I just displayed the equivalent sum of the histogram and as well as the sum that i was able to get from the vectors for double checking if they match so as to know the program is correct. Your task is to improve the performance of the program, as we can see that there are things that we can do in concurrent here namely (counting of histogram and getting the sum of the vector). Convert the program to support multithreading in counting the histogram and getting the sum of the vector. Use thread, locks, mutex to improve the performance of the program. #include #include #include #include using namespace std; int main(){ const long MAX = 500000000; const int RANGE = 6; vector num(MAX); unsigned long long int sum = 0; int h1=0; int h2=0; int h3=0; int h4=0; int h5=0; for (int i = 0; i < MAX; i++){ num[i] = rand() % RANGE; //cout << num[i] << endl; //generates random numbers 1 to 5 } auto start = chrono::high_resolution_clock::now(); for (int i = 0; i < MAX; i++){ sum = sum + num[i]; } for(int i=0; i < MAX; i++){ switch(num[i]){ case 1: h1++; break; case 2: h2++; break; case 3: h3++; break; case 4: h4++; break; case 5: h5++; break; } } auto end = chrono::high_resolution_clock::now(); auto duration = chrono::duration_cast(end - start); cout << "Histogram Result is : " << endl; cout << "1 ----> " << h1 << " SUM : " << h1 << endl; cout << "2 ----> " << h1 << " SUM : " << h2*2 << endl; cout << "3 ----> " << h1 << " SUM : " << h3*3 << endl; cout << "4 ----> " << h1 << " SUM : " << h4*4 << endl; cout << "5 ----> " << h1 << " SUM : " << h5*5 << endl; unsignedlonglongint histogramsum = h1 +(h2*2)+(h3*3)+(h4*4)+(h5*5); cout << "The sum of the vector is : " << sum << " and the sum of the histogram is " << histogramsum << endl; cout << "TOTAL RUNNING TIME IS : " << duration.count() << " milliseconds...."; return 0; }
The program initializes a vector with an assigned capacity and a random number for each element. The program counts the histogram of all of the contents of the vector ranging from 1 to 5 and displays it on the screen. The program is also getting the sum of all of the vectors and displays it afterwards. I just displayed the equivalent sum of the histogram and as well as the sum that i was able to get from the vectors for double checking if they match so as to know the program is correct. Your task is to improve the performance of the program, as we can see that there are things that we can do in concurrent here namely (counting of histogram and getting the sum of the vector). Convert the program to support multithreading in counting the histogram and getting the sum of the vector. Use thread, locks, mutex to improve the performance of the program. #include #include #include #include using namespace std; int main(){ const long MAX = 500000000; const int RANGE = 6; vector num(MAX); unsigned long long int sum = 0; int h1=0; int h2=0; int h3=0; int h4=0; int h5=0; for (int i = 0; i < MAX; i++){ num[i] = rand() % RANGE; //cout << num[i] << endl; //generates random numbers 1 to 5 } auto start = chrono::high_resolution_clock::now(); for (int i = 0; i < MAX; i++){ sum = sum + num[i]; } for(int i=0; i < MAX; i++){ switch(num[i]){ case 1: h1++; break; case 2: h2++; break; case 3: h3++; break; case 4: h4++; break; case 5: h5++; break; } } auto end = chrono::high_resolution_clock::now(); auto duration = chrono::duration_cast(end - start); cout << "Histogram Result is : " << endl; cout << "1 ----> " << h1 << " SUM : " << h1 << endl; cout << "2 ----> " << h1 << " SUM : " << h2*2 << endl; cout << "3 ----> " << h1 << " SUM : " << h3*3 << endl; cout << "4 ----> " << h1 << " SUM : " << h4*4 << endl; cout << "5 ----> " << h1 << " SUM : " << h5*5 << endl; unsignedlonglongint histogramsum = h1 +(h2*2)+(h3*3)+(h4*4)+(h5*5); cout << "The sum of the vector is : " << sum << " and the sum of the histogram is " << histogramsum << endl; cout << "TOTAL RUNNING TIME IS : " << duration.count() << " milliseconds...."; return 0; }
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter7: Arrays
Section7.6: The Standard Template Library (stl)
Problem 8E
Related questions
Question
The program initializes a vector with an assigned capacity and a random number for each element. The program counts the histogram of all of the contents of the vector ranging from 1 to 5 and displays it on the screen. The program is also getting the sum of all of the vectors and displays it afterwards. I just displayed the equivalent sum of the histogram and as well as the sum that i was able to get from the vectors for double checking if they match so as to know the program is correct.
Your task is to improve the performance of the program, as we can see that there are things that we can do in concurrent here namely (counting of histogram and getting the sum of the vector). Convert the program to support multithreading in counting the histogram and getting the sum of the vector.
Use thread, locks, mutex to improve the performance of the program.
#include <iostream>
#include <vector>
#include <chrono>
#include <cstdlib>
using namespace std;
int main(){
const long MAX = 500000000;
const int RANGE = 6;
vector<int> num(MAX);
unsigned long long int sum = 0;
int h1=0;
int h2=0;
int h3=0;
int h4=0;
int h5=0;
for (int i = 0; i < MAX; i++){
num[i] = rand() % RANGE;
//cout << num[i] << endl; //generates random numbers 1 to 5
}
auto start = chrono::high_resolution_clock::now();
for (int i = 0; i < MAX; i++){
sum = sum + num[i];
}
for(int i=0; i < MAX; i++){
switch(num[i]){
case 1: h1++; break;
case 2: h2++; break;
case 3: h3++; break;
case 4: h4++; break;
case 5: h5++; break;
}
}
auto end = chrono::high_resolution_clock::now();
auto duration = chrono::duration_cast<chrono::milliseconds>(end - start);
cout << "Histogram Result is : " << endl;
cout << "1 ----> " << h1 << " SUM : " << h1 << endl;
cout << "2 ----> " << h1 << " SUM : " << h2*2 << endl;
cout << "3 ----> " << h1 << " SUM : " << h3*3 << endl;
cout << "4 ----> " << h1 << " SUM : " << h4*4 << endl;
cout << "5 ----> " << h1 << " SUM : " << h5*5 << endl;
unsignedlonglongint histogramsum = h1 +(h2*2)+(h3*3)+(h4*4)+(h5*5);
cout << "The sum of the vector is : " << sum << " and the sum of the histogram is " << histogramsum << endl;
cout << "TOTAL RUNNING TIME IS : " << duration.count() << " milliseconds....";
return 0;
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
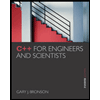
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
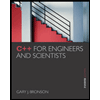
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr