Thank you for any assitance that you can provide. Need help with the following: Include comments at the beginning of each class that explain the purpose and functionality. Added additional error checking/validation mechanisms to ensure balance cannot be set to negative. Program now handles overdraft fees separately from withdrawals. Modified the logic to deduct the fee first and then proceed with the withdrawal. Override the toString method from the AccountBank class to provide a more standardized and convenient way of displaying the account information. Included some test cases in the Main class to demonstrate the functionality of the implemented classes. Java source code: Bank Account Class package bankAccount; public class AccountBank { //Variables private String firstName; private String lastName; private int accountID; private double balance; public AccountBank() { this.balance = 0; } public AccountBank(String firstName, String lastName, int accountID, double balance) { this.firstName = firstName; this.lastName = lastName; this.accountID = accountID; this.balance = balance; } //Setter & getter section for firstName, lastName, and accountID methods. public String getFirstName() { return firstName; } public String getLastName() { return lastName; } public int getAccountID() { return accountID; } public double getBalance() { return balance; } public void setFirstName(String firstName) { this.firstName = firstName; } public void setLastName(String lastName) { this.lastName = lastName; } public void setAccountID(int accountID) { this.accountID = accountID; } public void deposit(double transaction) { this.balance += transaction; } public void withdrawal(double transaction) { this.balance -= transaction; } public String accountSummary() { System.out.println("\nAccount Summary:"); String name = "First Name: " + firstName + "\nLast Name: " + lastName + "\n"; String accountInfo = name + "Account Number: " + accountID + "\nBalance: $" + balance; return accountInfo; } } } public int getAccountID() { return accountID; } public double getBalance() { return balance; } public void setFirstName(String firstName) { this.firstName = firstName; } public void setLastName(String lastName) { this.lastName = lastName; } public void setAccountID(int accountID) { this.accountID = accountID; } public void deposit(double transaction) { this.balance += transaction; } public void withdrawal(double transaction) { this.balance -= transaction; } public String accountSummary() { System.out.println("\nAccount Summary:"); String name = "First Name: " + firstName + "\nLast Name: " + lastName + "\n"; String accountInfo = name + "Account Number: " + accountID + "\nBalance: $" + balance; return accountInfo; } } Checking Account Class package bankAccount; public class CheckingAccount extends AccountBank { private double interestRate; public CheckingAccount(String firstName, String lastName, int accountID, double balance ) { super(firstName, lastName, accountID, balance); //Referring to superclass this.interestRate = 0.02; //interestRate attribute } public void processWithdrawal(double transaction) { if (transaction > this.getBalance()) { System.out.println("Non-sufficient funds (NSF)! An overdraft fee of $30 has been charged to your account."); this.withdrawal(30); this.withdrawal(transaction); } else { this.withdrawal(transaction); } } public void displayAccount() { System.out.println("\nPersonal Checking Account Information:"); System.out.println("First Name: " + this.getFirstName()); System.out.println("Last Name: " + this.getLastName()); System.out.println("Account ID: " + this.getAccountID()); System.out.println("Balance: $" + this.getBalance()); System.out.println("Interest Rate: " + interestRate + "%"); } } Test Class package bankAccount; //Test class public class Main { public static void main(String[] args) { //New instance for CheckingAccount. CheckingAccount obj = new CheckingAccount("Art", "Vandelay", 25088601, 250.0); System.out.println(obj.accountSummary()); System.out.println("\nMaking a deposit of $50.."); obj.deposit(50);//New instance for deposit. System.out.println("New Balance: $" + obj.getBalance());//Display new balance. System.out.println("\nMaking a withdrawal of $320.."); obj.processWithdrawal(320);//New instance for withdrawal. System.out.println("New Balance: $" + obj.getBalance()); obj.displayAccount(); } }
Thank you for any assitance that you can provide. Need help with the following:
- Include comments at the beginning of each class that explain the purpose and functionality.
- Added additional error checking/validation
mechanisms to ensure balance cannot be set to negative. - Program now handles overdraft fees separately from withdrawals.
- Modified the logic to deduct the fee first and then proceed with the withdrawal.
- Override the toString method from the AccountBank class to provide a more standardized and convenient way of displaying the account information.
- Included some test cases in the Main class to demonstrate the functionality of the implemented classes.
Java source code:
Bank Account Class
package bankAccount;
public class AccountBank {
//Variables
private String firstName;
private String lastName;
private int accountID;
private double balance;
public AccountBank() {
this.balance = 0;
}
public AccountBank(String firstName, String lastName, int accountID, double balance) {
this.firstName = firstName;
this.lastName = lastName;
this.accountID = accountID;
this.balance = balance;
}
//Setter & getter section for firstName, lastName, and accountID methods.
public String getFirstName() {
return firstName;
}
public String getLastName() {
return lastName;
}
public int getAccountID() {
return accountID;
}
public double getBalance() {
return balance;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
public void setAccountID(int accountID) {
this.accountID = accountID;
}
public void deposit(double transaction) {
this.balance += transaction;
}
public void withdrawal(double transaction) {
this.balance -= transaction;
}
public String accountSummary() {
System.out.println("\nAccount Summary:");
String name = "First Name: " + firstName + "\nLast Name: " + lastName + "\n";
String accountInfo = name + "Account Number: " + accountID + "\nBalance: $" + balance;
return accountInfo;
}
}
}
public int getAccountID() {
return accountID;
}
public double getBalance() {
return balance;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
public void setAccountID(int accountID) {
this.accountID = accountID;
}
public void deposit(double transaction) {
this.balance += transaction;
}
public void withdrawal(double transaction) {
this.balance -= transaction;
}
public String accountSummary() {
System.out.println("\nAccount Summary:");
String name = "First Name: " + firstName + "\nLast Name: " + lastName + "\n";
String accountInfo = name + "Account Number: " + accountID + "\nBalance: $" + balance;
return accountInfo;
}
}
Checking Account Class
package bankAccount;
public class CheckingAccount extends AccountBank {
private double interestRate;
public CheckingAccount(String firstName, String lastName, int accountID, double balance ) {
super(firstName, lastName, accountID, balance); //Referring to superclass
this.interestRate = 0.02; //interestRate attribute
}
public void processWithdrawal(double transaction) {
if (transaction > this.getBalance()) {
System.out.println("Non-sufficient funds (NSF)! An overdraft fee of $30 has been charged to your account.");
this.withdrawal(30);
this.withdrawal(transaction);
}
else {
this.withdrawal(transaction);
}
}
public void displayAccount() {
System.out.println("\nPersonal Checking Account Information:");
System.out.println("First Name: " + this.getFirstName());
System.out.println("Last Name: " + this.getLastName());
System.out.println("Account ID: " + this.getAccountID());
System.out.println("Balance: $" + this.getBalance());
System.out.println("Interest Rate: " + interestRate + "%");
}
}
Test Class
package bankAccount;
//Test class
public class Main {
public static void main(String[] args) {
//New instance for CheckingAccount.
CheckingAccount obj = new CheckingAccount("Art", "Vandelay", 25088601, 250.0);
System.out.println(obj.accountSummary());
System.out.println("\nMaking a deposit of $50..");
obj.deposit(50);//New instance for deposit.
System.out.println("New Balance: $" + obj.getBalance());//Display new balance.
System.out.println("\nMaking a withdrawal of $320..");
obj.processWithdrawal(320);//New instance for withdrawal.
System.out.println("New Balance: $" + obj.getBalance());
obj.displayAccount();
}
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

In the Bank Account class, is there a way to add error-checking/validation
The processWithdrawal method in the Checking Account class is not processing separately from the withdrawal method in the Bank Account class nor is the withdrawal fee showing up on the console. What is the fix?
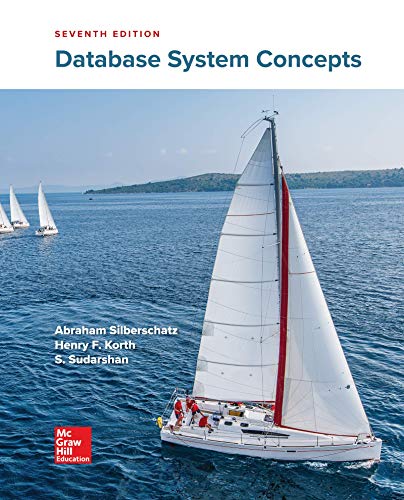
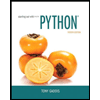
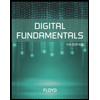
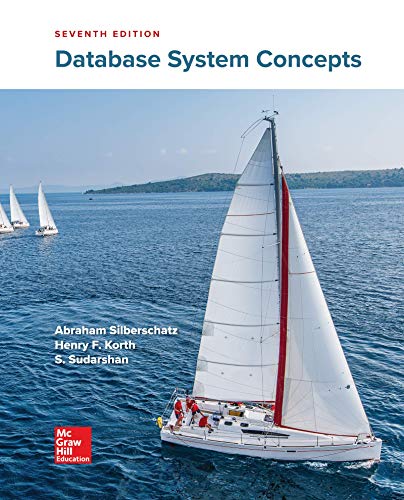
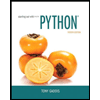
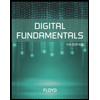
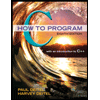
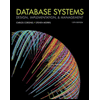
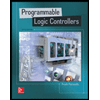