The Mastermind game board game is a code breaking game with two players. One player (your program) becomes the codemaker, the other the codebreaker. The codemaker (your program) creates a 4 digit secret code which is randomly generated by the supplied code below and the codebreaker tries to guess the 4 digit pattern. import random secretCode =[] for i in range (0,4): n = random.randint(1,9) secretCode.append(str(n)) print (secretCode) # take this out when you are playing the game for real because it is a secret The secret code pattern generated above will consist of any of the digits 1-9 and can contain multiples of the same digit. Your program should then tells the codebreaker which digits should be in their guess by displaying a sorted list of the digit characters contained in the secret code python list . Use the loop for digit in sorted(secretCode): #display each digit on the same line for the player to see; there could be duplicate digits in the secret Prompt the codebreaker for a 4 digit pattern guess of valid digits listed (no spaces). Once entered, your program provides feedback by displaying a message stating how many values have been entered correctly (such as 2) but not which ones are in the correct position. To do this, you will have to use a for loop to loop through the list and check each position of the secret code to see if that particular digit character matches the same corresponding position in the string the user entered for their guess. The player gets 5 guesses and if they do not get the secret code in 5 guesses, they lose. If they guess the secret code, display a congratulatory message. Requirements: Your program will play the guessing game as follows: Display a sorted list of possible digits for the code that were generated from the randomizing. Prompt the codebreaker for a guess. Count how many digits were in the correct place. End the game if: the guess is correct and print out a winner message it was the last guess (the fifth) and the guess is incorrect. Print out a loser message and the value of the secret code. If no end condition occurs, print out how many of the guess values are exactly right (correct number in the correct position). Example. The secret code is 1234 and the guess is 4132. The feedback would be 1 digit exactly correct. Continue the game until an end condition occurs. Sample Interaction (you may use your own style as long as the required functionality is there) Let’s play the Mystery code game! Here are the digits that are valid guesses. 1668 Enter your guess 8166 You guessed 1 correctly Enter your guess 6816 You guessed 0 correctly Enter your guess 6861 You guessed 1 correctly Enter your guess 1668 Congratulations, you won! Let’s play the Mystery code game! Here are the digits that are valid guesses. 5578 Enter your guess 7855 You guessed 1 correctly Enter your guess 5785 You guessed 2 correctly Enter your guess 5875 You guessed 1 correctly Enter your guess 5578 You guessed 2 correctly Enter your guess 5758 Sorry, you loose!
The Problem
The Mastermind game board game is a code breaking game with two players. One player (your program) becomes the codemaker, the other the codebreaker. The codemaker (your program) creates a 4 digit secret code which is randomly generated by the supplied code below and the codebreaker tries to guess the 4 digit pattern.
import random
secretCode =[]
for i in range (0,4):
n = random.randint(1,9)
secretCode.append(str(n))
print (secretCode) # take this out when you are playing the game for real because it is a secret
The secret code pattern generated above will consist of any of the digits 1-9 and can contain multiples of the same digit.
Your program should then tells the codebreaker which digits should be in their guess by displaying a sorted list of the digit characters contained in the secret code python list . Use the loop
for digit in sorted(secretCode):
#display each digit on the same line for the player to see; there could be duplicate digits in the secret
Prompt the codebreaker for a 4 digit pattern guess of valid digits listed (no spaces). Once entered, your program provides feedback by displaying a message stating how many values have been entered correctly (such as 2) but not which ones are in the correct position. To do this, you will have to use a for loop to loop through the list and check each position of the secret code to see if that particular digit character matches the same corresponding position in the string the user entered for their guess.
The player gets 5 guesses and if they do not get the secret code in 5 guesses, they lose. If they guess the secret code, display a congratulatory message.
Requirements:
Your program will play the guessing game as follows:
- Display a sorted list of possible digits for the code that were generated from the randomizing.
- Prompt the codebreaker for a guess.
- Count how many digits were in the correct place.
- End the game if:
- the guess is correct and print out a winner message
- it was the last guess (the fifth) and the guess is incorrect. Print out a loser message and the value of the secret code.
- If no end condition occurs, print out how many of the guess values are exactly right (correct number in the correct position). Example. The secret code is 1234 and the guess is 4132. The feedback would be 1 digit exactly correct.
- Continue the game until an end condition occurs.
Sample Interaction (you may use your own style as long as the required functionality is there)
Let’s play the Mystery code game!
Here are the digits that are valid guesses.
1668
Enter your guess
8166
You guessed 1 correctly
Enter your guess
6816
You guessed 0 correctly
Enter your guess
6861
You guessed 1 correctly
Enter your guess
1668
Congratulations, you won!
Let’s play the Mystery code game!
Here are the digits that are valid guesses.
5578
Enter your guess
7855
You guessed 1 correctly
Enter your guess
5785
You guessed 2 correctly
Enter your guess
5875
You guessed 1 correctly
Enter your guess
5578
You guessed 2 correctly
Enter your guess
5758
Sorry, you loose!

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

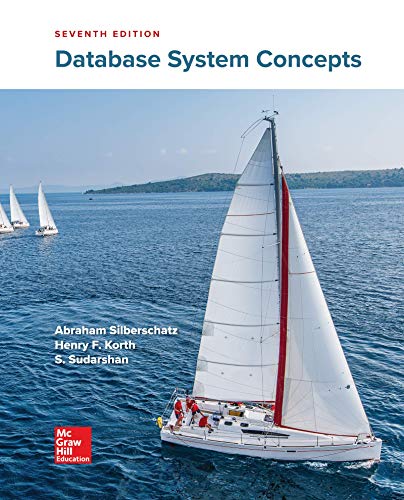
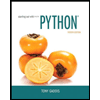
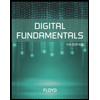
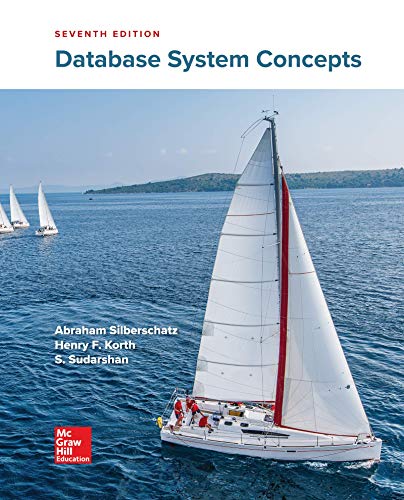
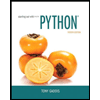
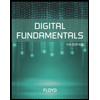
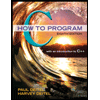
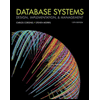
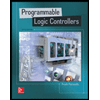