The game begins by welcoming the user and asking their name. • Then it displays the rules of the game and a menu to the user. The menu must display the options to select the doors, the value of the treasures behind each door, and the minimum token required to open those doors (see the sample outputs below to get an idea). • Now the user can select a door of their choice or decide to quit the game. Note that the user is allowed to type incorrect inputs. If they do not type a valid option, your program should print an error message and show the menu again. (Hint: you can use a while loop to help with this.) • The user has a chance to change their mind at this stage and choose another door if they wish but they cannot quit now. Again, the user input must be validated. Note that user inputs that are of type strings are not case-sensitive. • Next, the magic wheel randomly generates a token. • Your program must display the token’s type to the user and decide if that is sufficient to open the selected door. If the user wins the treasures, your program must show how much the user won, congratulate them, and end the game. Otherwise, it must thank the user for playing before terminating the game. Hints: − you may want to use while loops to validate user inputs, i.e., repeat the loop until the user enters the correct (type of) input. − you may want to use Python’s random module (any function from this module can be used) to generate a token based on a random value. You can generate a random value between 0 and 1 (or 0 and 100). If this number is less than or equal to 60%, it is a silver token. Else if the number is less than or equal to 60+30 = 90%, it is a gold token. Otherwise, it is a diamond token. You will find below several sample runs of the program that demonstrate what your output could look like at this stage (user input is highlighted for emphasis). You may customize the messages and modify the format of the output. Make sure to display all required information and the output should be neatly formatted • The game will now span over three (3) levels. The user should be able to play all three levels starting with the first, the second, and then the third level. • At each level, the user will be shown a menu similar to the one you used in section 1. Note that, in this version of the game, the values of the hidden treasures get multiplied by the level number (see the sample output below). • As before, the user should be able to select a door, spin the magic wheel to collect a randomly selected token, and win (or lose the chance to win) the treasures. Again, invalid selections should be handled with suitable error messages and the prompt should be repeated. • After completing each level, the user should have the option to continue with the next level (or quit the game), irrespective of the results of the previous level. • The game must end when the user completes the third level. Sample output - 1: Hey gamer, what's your preferred name? >> Lyon Welcome to the game, Lyon! Want to find some secret treasures? Let's begin. You can choose a door to get the treasures hidden behind it. But you must also collect a token from the magic wheel to open that door. Check how much you can win and the token you need to open a door. If you win this level, you'll get 1 x the value of the treasure. But if you lose, 1 x the value of the treasure will be taken away from you. ------------------------- Level-1 ----------------------------------- Doors Treasures' value Token type [1] Door-1 $100.00 Silver [2] Door-2 $200.00 Gold [3] Door-3 $300.00 Diamond ---------------------------------------------------------------------- Which door do you want to choose? Type [1, 2, or 3]. To quit the game enter 'q'. >> 1 Do you want to change your decision and choose another door? Enter 'Yes'/'No'. >> no Lyon, you have selected Door-1. Please spin the magic wheel to collect your token. ...... (drum roll) .... you've got a gold token (81.0%). Congrats!! You've won the hidden treasure worth $100.00. Do you want to play the next level (Level-2) (enter 'Yes'/'No')? >> yes If you win this level, you'll get 2 x the value of the treasure. But if you lose, 2 x the value of the treasure will be taken away from you. ------------------------- Level-2 ----------------------------------- Doors Treasures' value Token type [1] Door-1 $200.00 Silver [2] Door-2 $400.00 Gold [3] Door-3 $600.00 Diamond ---------------------------------------------------------------------- Which door do you want to choose? Type [1, 2, or 3]. To quit the game enter 'q'. >> 3 Do you want to change your decision and choose another door? Enter 'Yes'/'No'. >> yes Which door do you want to choose? Type [1, 2, or 3] >> 1 Lyon, you have selected Door-1. Please spin the magic wheel to collect your token. ...... (drum roll) .... you've got a gold token (74.6%). Congrats!! You've won the hidden treasure worth $200.00. Do you want to play the next level (Level-3) (enter 'Yes'/'No')? >>yes
The game begins by welcoming the user and asking their name. • Then it displays the rules of the game and a menu to the user. The menu must display the options to select the doors, the value of the treasures behind each door, and the minimum token required to open those doors (see the sample outputs below to get an idea). • Now the user can select a door of their choice or decide to quit the game. Note that the user is allowed to type incorrect inputs. If they do not type a valid option, your program should print an error message and show the menu again. (Hint: you can use a while loop to help with this.) • The user has a chance to change their mind at this stage and choose another door if they wish but they cannot quit now. Again, the user input must be validated. Note that user inputs that are of type strings are not case-sensitive. • Next, the magic wheel randomly generates a token. • Your program must display the token’s type to the user and decide if that is sufficient to open the selected door. If the user wins the treasures, your program must show how much the user won, congratulate them, and end the game. Otherwise, it must thank the user for playing before terminating the game. Hints: − you may want to use while loops to validate user inputs, i.e., repeat the loop until the user enters the correct (type of) input. − you may want to use Python’s random module (any function from this module can be used) to generate a token based on a random value. You can generate a random value between 0 and 1 (or 0 and 100). If this number is less than or equal to 60%, it is a silver token. Else if the number is less than or equal to 60+30 = 90%, it is a gold token. Otherwise, it is a diamond token. You will find below several sample runs of the program that demonstrate what your output could look like at this stage (user input is highlighted for emphasis). You may customize the messages and modify the format of the output. Make sure to display all required information and the output should be neatly formatted • The game will now span over three (3) levels. The user should be able to play all three levels starting with the first, the second, and then the third level. • At each level, the user will be shown a menu similar to the one you used in section 1. Note that, in this version of the game, the values of the hidden treasures get multiplied by the level number (see the sample output below). • As before, the user should be able to select a door, spin the magic wheel to collect a randomly selected token, and win (or lose the chance to win) the treasures. Again, invalid selections should be handled with suitable error messages and the prompt should be repeated. • After completing each level, the user should have the option to continue with the next level (or quit the game), irrespective of the results of the previous level. • The game must end when the user completes the third level. Sample output - 1: Hey gamer, what's your preferred name? >> Lyon Welcome to the game, Lyon! Want to find some secret treasures? Let's begin. You can choose a door to get the treasures hidden behind it. But you must also collect a token from the magic wheel to open that door. Check how much you can win and the token you need to open a door. If you win this level, you'll get 1 x the value of the treasure. But if you lose, 1 x the value of the treasure will be taken away from you. ------------------------- Level-1 ----------------------------------- Doors Treasures' value Token type [1] Door-1 $100.00 Silver [2] Door-2 $200.00 Gold [3] Door-3 $300.00 Diamond ---------------------------------------------------------------------- Which door do you want to choose? Type [1, 2, or 3]. To quit the game enter 'q'. >> 1 Do you want to change your decision and choose another door? Enter 'Yes'/'No'. >> no Lyon, you have selected Door-1. Please spin the magic wheel to collect your token. ...... (drum roll) .... you've got a gold token (81.0%). Congrats!! You've won the hidden treasure worth $100.00. Do you want to play the next level (Level-2) (enter 'Yes'/'No')? >> yes If you win this level, you'll get 2 x the value of the treasure. But if you lose, 2 x the value of the treasure will be taken away from you. ------------------------- Level-2 ----------------------------------- Doors Treasures' value Token type [1] Door-1 $200.00 Silver [2] Door-2 $400.00 Gold [3] Door-3 $600.00 Diamond ---------------------------------------------------------------------- Which door do you want to choose? Type [1, 2, or 3]. To quit the game enter 'q'. >> 3 Do you want to change your decision and choose another door? Enter 'Yes'/'No'. >> yes Which door do you want to choose? Type [1, 2, or 3] >> 1 Lyon, you have selected Door-1. Please spin the magic wheel to collect your token. ...... (drum roll) .... you've got a gold token (74.6%). Congrats!! You've won the hidden treasure worth $200.00. Do you want to play the next level (Level-3) (enter 'Yes'/'No')? >>yes
Programming Logic & Design Comprehensive
9th Edition
ISBN:9781337669405
Author:FARRELL
Publisher:FARRELL
Chapter8: Advanced Data Handling Concepts
Section: Chapter Questions
Problem 1GZ
Related questions
Question
• The game begins by welcoming the user and asking their name.
• Then it displays the rules of the game and a menu to the user. The menu must display the
options to select the doors, the value of the treasures behind each door, and the minimum
token required to open those doors (see the sample outputs below to get an idea).
• Now the user can select a door of their choice or decide to quit the game. Note that the user
is allowed to type incorrect inputs. If they do not type a valid option, your program should
print an error message and show the menu again. (Hint: you can use a while loop to help
with this.)
• Then it displays the rules of the game and a menu to the user. The menu must display the
options to select the doors, the value of the treasures behind each door, and the minimum
token required to open those doors (see the sample outputs below to get an idea).
• Now the user can select a door of their choice or decide to quit the game. Note that the user
is allowed to type incorrect inputs. If they do not type a valid option, your program should
print an error message and show the menu again. (Hint: you can use a while loop to help
with this.)
• The user has a chance to change their mind at this stage and choose another door if they
wish but they cannot quit now. Again, the user input must be validated. Note that user inputs
that are of type strings are not case-sensitive.
• Next, the magic wheel randomly generates a token.
• Your program must display the token’s type to the user and decide if that is sufficient to open
the selected door. If the user wins the treasures, your program must show how much the
user won, congratulate them, and end the game. Otherwise, it must thank the user for
playing before terminating the game.
Hints:
− you may want to use while loops to validate user inputs, i.e., repeat the loop until the
user enters the correct (type of) input.
− you may want to use Python’s random module (any function from this module can be
used) to generate a token based on a random value. You can generate a random value
between 0 and 1 (or 0 and 100). If this number is less than or equal to 60%, it is a silver
token. Else if the number is less than or equal to 60+30 = 90%, it is a gold token. Otherwise,
it is a diamond token.
You will find below several sample runs of the program that demonstrate what your output could
look like at this stage (user input is highlighted for emphasis). You may customize the messages
and modify the format of the output. Make sure to display all required information and the
output should be neatly formatted
wish but they cannot quit now. Again, the user input must be validated. Note that user inputs
that are of type strings are not case-sensitive.
• Next, the magic wheel randomly generates a token.
• Your program must display the token’s type to the user and decide if that is sufficient to open
the selected door. If the user wins the treasures, your program must show how much the
user won, congratulate them, and end the game. Otherwise, it must thank the user for
playing before terminating the game.
Hints:
− you may want to use while loops to validate user inputs, i.e., repeat the loop until the
user enters the correct (type of) input.
− you may want to use Python’s random module (any function from this module can be
used) to generate a token based on a random value. You can generate a random value
between 0 and 1 (or 0 and 100). If this number is less than or equal to 60%, it is a silver
token. Else if the number is less than or equal to 60+30 = 90%, it is a gold token. Otherwise,
it is a diamond token.
You will find below several sample runs of the program that demonstrate what your output could
look like at this stage (user input is highlighted for emphasis). You may customize the messages
and modify the format of the output. Make sure to display all required information and the
output should be neatly formatted
• The game will now span over three (3) levels. The user should be able to play all three levels
starting with the first, the second, and then the third level.
• At each level, the user will be shown a menu similar to the one you used in section 1. Note
that, in this version of the game, the values of the hidden treasures get multiplied by the
level number (see the sample output below).
• As before, the user should be able to select a door, spin the magic wheel to collect a
randomly selected token, and win (or lose the chance to win) the treasures. Again, invalid
selections should be handled with suitable error messages and the prompt should be
repeated.
• After completing each level, the user should have the option to continue with the next level
(or quit the game), irrespective of the results of the previous level.
• The game must end when the user completes the third level.
Sample output - 1:
• At each level, the user will be shown a menu similar to the one you used in section 1. Note
that, in this version of the game, the values of the hidden treasures get multiplied by the
level number (see the sample output below).
• As before, the user should be able to select a door, spin the magic wheel to collect a
randomly selected token, and win (or lose the chance to win) the treasures. Again, invalid
selections should be handled with suitable error messages and the prompt should be
repeated.
• After completing each level, the user should have the option to continue with the next level
(or quit the game), irrespective of the results of the previous level.
• The game must end when the user completes the third level.
Sample output - 1:
Hey gamer, what's your preferred name?
>> Lyon
Welcome to the game, Lyon! Want to find some secret treasures? Let's begin.
You can choose a door to get the treasures hidden behind it.
But you must also collect a token from the magic wheel to open that door.
Check how much you can win and the token you need to open a door.
If you win this level, you'll get 1 x the value of the treasure.
But if you lose, 1 x the value of the treasure will be taken away from you.
------------------------- Level-1 -----------------------------------
Doors Treasures' value Token type
[1] Door-1 $100.00 Silver
[2] Door-2 $200.00 Gold
[3] Door-3 $300.00 Diamond
----------------------------------------------------------------------
Which door do you want to choose? Type [1, 2, or 3]. To quit the game enter 'q'.
>> 1
Do you want to change your decision and choose another door? Enter 'Yes'/'No'.
>> no
Lyon, you have selected Door-1. Please spin the magic wheel to collect your token.
...... (drum roll) .... you've got a gold token (81.0%).
Congrats!! You've won the hidden treasure worth $100.00.
Do you want to play the next level (Level-2) (enter 'Yes'/'No')? >> yes
If you win this level, you'll get 2 x the value of the treasure.
But if you lose, 2 x the value of the treasure will be taken away from you.
------------------------- Level-2 -----------------------------------
Doors Treasures' value Token type
[1] Door-1 $200.00 Silver
[2] Door-2 $400.00 Gold
[3] Door-3 $600.00 Diamond
----------------------------------------------------------------------
Which door do you want to choose? Type [1, 2, or 3]. To quit the game enter 'q'.
>> 3
Do you want to change your decision and choose another door? Enter 'Yes'/'No'.
>> yes
Which door do you want to choose? Type [1, 2, or 3] >> 1
Lyon, you have selected Door-1. Please spin the magic wheel to collect your token.
...... (drum roll) .... you've got a gold token (74.6%).
Congrats!! You've won the hidden treasure worth $200.00.
Do you want to play the next level (Level-3) (enter 'Yes'/'No')? >>yes
>> Lyon
Welcome to the game, Lyon! Want to find some secret treasures? Let's begin.
You can choose a door to get the treasures hidden behind it.
But you must also collect a token from the magic wheel to open that door.
Check how much you can win and the token you need to open a door.
If you win this level, you'll get 1 x the value of the treasure.
But if you lose, 1 x the value of the treasure will be taken away from you.
------------------------- Level-1 -----------------------------------
Doors Treasures' value Token type
[1] Door-1 $100.00 Silver
[2] Door-2 $200.00 Gold
[3] Door-3 $300.00 Diamond
----------------------------------------------------------------------
Which door do you want to choose? Type [1, 2, or 3]. To quit the game enter 'q'.
>> 1
Do you want to change your decision and choose another door? Enter 'Yes'/'No'.
>> no
Lyon, you have selected Door-1. Please spin the magic wheel to collect your token.
...... (drum roll) .... you've got a gold token (81.0%).
Congrats!! You've won the hidden treasure worth $100.00.
Do you want to play the next level (Level-2) (enter 'Yes'/'No')? >> yes
If you win this level, you'll get 2 x the value of the treasure.
But if you lose, 2 x the value of the treasure will be taken away from you.
------------------------- Level-2 -----------------------------------
Doors Treasures' value Token type
[1] Door-1 $200.00 Silver
[2] Door-2 $400.00 Gold
[3] Door-3 $600.00 Diamond
----------------------------------------------------------------------
Which door do you want to choose? Type [1, 2, or 3]. To quit the game enter 'q'.
>> 3
Do you want to change your decision and choose another door? Enter 'Yes'/'No'.
>> yes
Which door do you want to choose? Type [1, 2, or 3] >> 1
Lyon, you have selected Door-1. Please spin the magic wheel to collect your token.
...... (drum roll) .... you've got a gold token (74.6%).
Congrats!! You've won the hidden treasure worth $200.00.
Do you want to play the next level (Level-3) (enter 'Yes'/'No')? >>yes
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
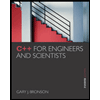
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
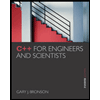
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr