The code of a sequential search function is shown on textbook page 60. In fact, if the list is already sorted, the search can halt when the target is less than a given element in the list. For example, given my_list = [2, 5, 7, 9, 14, 27], if the search target is 6, the search can halt when it reaches 7 because it is impossible for 6 to exist after 7. Define a function linearSearchSorted, which is used to search a sorted list. This function displays the position of the target item if found, or 'Target not found' otherwise. It also displays the elements it has visited. To test your function, search for these targets in the list [2, 5, 7, 9, 14, 27]: 2, 6, 14, 27 and 28.
TROUBLESHOOT my PYTHON code, please :)
The code of a sequential search function is shown on textbook page 60. In fact, if the list is already sorted, the search can halt when the target is less than a given element in the list. For example, given my_list = [2, 5, 7, 9, 14, 27], if the search target is 6, the search can halt when it reaches 7 because it is impossible for 6 to exist after 7. Define a function linearSearchSorted, which is used to search a sorted list. This function displays the position of the target item if found, or 'Target not found' otherwise. It also displays the elements it has visited. To test your function, search for these targets in the list [2, 5, 7, 9, 14, 27]: 2, 6, 14, 27 and 28.
Expected output:
List: [2, 5, 7, 9, 14, 27]
Search target: 2
Elements visited: 2
Target found at position 0
Search target: 6
Elements visited: 2 5 7
Target not found
Search target: 14
Elements visited: 2 5 7 9 14
Target found at position 4
Search target: 27
Elements visited: 2 5 7 9 14 27
Target found at position 5
Search target: 28
Elements visited: 2 5 7 9 14 27
Target not found
MY CODE:
def sequentialSearch(target, lyst):
position = 0
while position < len(lyst):
if target == lyst[position]:
print("Search Target: ", target)
print("Elements visited: ", *lyst[:position+1])
print("Target found at position ", position)
break
elif target < lyst[position]:
print("Search Target: ", target)
print("Elements visited: ", lyst[:position+1])
print("Target not found")
return -1
position += 1
return -1
def main():
my_list = [2, 5, 7, 9, 14, 27]
sequentialSearch(2, my_list)
sequentialSearch(6, my_list)
sequentialSearch(14, my_list)
sequentialSearch(4, my_list)
sequentialSearch(27, my_list)
sequentialSearch(28, my_list)
main()
AS YOU SEE IT RUNS JUST FINE UNTIL I ASK IT TO FIND 28! IT IS SUPPOSED TO RETURN THE ENTIRE LIST AND THEN SAY "TARGET NOT FOUND" BUT IT DOESNT. PLEASE HELP ME

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

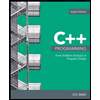
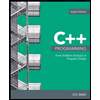