Given the following code in Java and attached images( containing related code): //Controller (ONLY EDIT THE Controller.java file) import java.util.LinkedList; import java.util.Scanner; public class Controller { public Controller() { LinkedList googList = new LinkedList(); LinkedList amazList = new LinkedList(); Scanner input = new Scanner(System.in); do { System.out.print("Enter 1 for Google stock or 2 for Amazon, 3 to quit: "); int stockSelect = input.nextInt(); if(stockSelect == 3) break; System.out.print("Input 1 to buy, 2 to sell: "); int controlNum = input.nextInt(); System.out.print("How many stocks: "); int quantity = input.nextInt(); if(controlNum == 1) { System.out.print("At what price: "); double price = input.nextDouble(); if(stockSelect == 1) { Controller.buyStock(googList, "Google", quantity, price); } else Controller.buyStock(amazList, "Amazon", quantity, price); } else { System.out.print("Press 1 for LIFO accounting, 2 for FIFO accounting: "); controlNum = input.nextInt(); if(controlNum == 1) { if(stockSelect == 1) Controller.sellLIFO(googList, quantity); else Controller.sellLIFO(amazList, quantity); } else { if(stockSelect == 1) Controller.sellFIFO(googList, quantity); else Controller.sellFIFO(amazList, quantity); } } } while(true); input.close(); } public static void buyStock(LinkedList list, String name, int quantity, double price) { Stock temp = new Stock(name,quantity,price); list.push(temp); System.out.printf("You bought %d shares of %s stock at $%.2f per share %n", quantity, name, price); } public static void sellLIFO(LinkedList list, int numToSell) { // You need to write the code to sell the stock using the LIFO method (Stack) // You also need to calculate the profit/loss on the sale double total = 0; // this variable will store the total after the sale double profit = 0; // the price paid minus the sale price, negative # means a loss System.out.printf("You sold %d shares of %s stock at %.2f per share %n", numToSell, list.element().getName(), total/numToSell); System.out.printf("You made $%.2f on the sale %n", profit); } public static void sellFIFO(LinkedList list, int numToSell) { // You need to write the code to sell the stock using the FIFO method (Queue) // You also need to calculate the profit/loss on the sale double total = 0; // this variable will store the total after the sale double profit = 0; // the price paid minus the sale price, negative # means a loss System.out.printf("You sold %d shares of %s stock at %.2f per share %n", numToSell, list.element().getName(), total/numToSell); System.out.printf("You made $%.2f on the sale %n", profit); } } //Driver public class Driver { public static void main(String[] args) { System.out.println("Java Stock Exchange"); new Controller(); } } //Refer to images first for code and this will come after in order //Stock public Stock(int quantity, double price) { this.name = "undefined"; this.quantity = quantity; this.price = price; } public String getName() { return name; } public void setName(String name) { this.name = name; } public int getQuantity() { return quantity; } public void setQuantity(int quantity) { this.quantity = quantity; } public double getPrice() { return price; } public void setPrice(double price) { this.price = price; } public String toString() { return "Stock: " + this.getName() + " Quantity: " + this.getQuantity() + " Price: " + this.getPrice(); } } Implement the following: 1. Add the missing functionality in “controller.java” to finish the “sellLIFO” and “sellFIFO” methods. 2. Provide error checking so users can’t sell a stock they don’t own or try to sell more stock than they own. Eliminate that runtime exception. 3. Must change to where user has to input the stock they want to work with, not just Google or Amazon that can be stored in the LinkedList. 4. We like the layout and design of the application so don’t change any of the other methods or objects. Only edit the Controller.java file! 5. Solution must be new (for postive feedback) and output should state the profit from selling number of stocks that user inputs!
Given the following code in Java and attached images( containing related code): //Controller (ONLY EDIT THE Controller.java file) import java.util.LinkedList; import java.util.Scanner; public class Controller { public Controller() { LinkedList googList = new LinkedList(); LinkedList amazList = new LinkedList(); Scanner input = new Scanner(System.in); do { System.out.print("Enter 1 for Google stock or 2 for Amazon, 3 to quit: "); int stockSelect = input.nextInt(); if(stockSelect == 3) break; System.out.print("Input 1 to buy, 2 to sell: "); int controlNum = input.nextInt(); System.out.print("How many stocks: "); int quantity = input.nextInt(); if(controlNum == 1) { System.out.print("At what price: "); double price = input.nextDouble(); if(stockSelect == 1) { Controller.buyStock(googList, "Google", quantity, price); } else Controller.buyStock(amazList, "Amazon", quantity, price); } else { System.out.print("Press 1 for LIFO accounting, 2 for FIFO accounting: "); controlNum = input.nextInt(); if(controlNum == 1) { if(stockSelect == 1) Controller.sellLIFO(googList, quantity); else Controller.sellLIFO(amazList, quantity); } else { if(stockSelect == 1) Controller.sellFIFO(googList, quantity); else Controller.sellFIFO(amazList, quantity); } } } while(true); input.close(); } public static void buyStock(LinkedList list, String name, int quantity, double price) { Stock temp = new Stock(name,quantity,price); list.push(temp); System.out.printf("You bought %d shares of %s stock at $%.2f per share %n", quantity, name, price); } public static void sellLIFO(LinkedList list, int numToSell) { // You need to write the code to sell the stock using the LIFO method (Stack) // You also need to calculate the profit/loss on the sale double total = 0; // this variable will store the total after the sale double profit = 0; // the price paid minus the sale price, negative # means a loss System.out.printf("You sold %d shares of %s stock at %.2f per share %n", numToSell, list.element().getName(), total/numToSell); System.out.printf("You made $%.2f on the sale %n", profit); } public static void sellFIFO(LinkedList list, int numToSell) { // You need to write the code to sell the stock using the FIFO method (Queue) // You also need to calculate the profit/loss on the sale double total = 0; // this variable will store the total after the sale double profit = 0; // the price paid minus the sale price, negative # means a loss System.out.printf("You sold %d shares of %s stock at %.2f per share %n", numToSell, list.element().getName(), total/numToSell); System.out.printf("You made $%.2f on the sale %n", profit); } } //Driver public class Driver { public static void main(String[] args) { System.out.println("Java Stock Exchange"); new Controller(); } } //Refer to images first for code and this will come after in order //Stock public Stock(int quantity, double price) { this.name = "undefined"; this.quantity = quantity; this.price = price; } public String getName() { return name; } public void setName(String name) { this.name = name; } public int getQuantity() { return quantity; } public void setQuantity(int quantity) { this.quantity = quantity; } public double getPrice() { return price; } public void setPrice(double price) { this.price = price; } public String toString() { return "Stock: " + this.getName() + " Quantity: " + this.getQuantity() + " Price: " + this.getPrice(); } } Implement the following: 1. Add the missing functionality in “controller.java” to finish the “sellLIFO” and “sellFIFO” methods. 2. Provide error checking so users can’t sell a stock they don’t own or try to sell more stock than they own. Eliminate that runtime exception. 3. Must change to where user has to input the stock they want to work with, not just Google or Amazon that can be stored in the LinkedList. 4. We like the layout and design of the application so don’t change any of the other methods or objects. Only edit the Controller.java file! 5. Solution must be new (for postive feedback) and output should state the profit from selling number of stocks that user inputs!
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
100%
Given the following code in Java and attached images( containing related code):
//Controller (ONLY EDIT THE Controller.java file)
import java.util.LinkedList;
import java.util.Scanner;
public class Controller {
public Controller() {
LinkedList<Stock> googList = new LinkedList<Stock>();
LinkedList<Stock> amazList = new LinkedList<Stock>();
Scanner input = new Scanner(System.in);
do {
System.out.print("Enter 1 for Google stock or 2 for Amazon, 3 to quit: ");
int stockSelect = input.nextInt();
if(stockSelect == 3)
break;
System.out.print("Input 1 to buy, 2 to sell: ");
int controlNum = input.nextInt();
System.out.print("How many stocks: ");
int quantity = input.nextInt();
if(controlNum == 1) {
System.out.print("At what price: ");
double price = input.nextDouble();
if(stockSelect == 1) {
Controller.buyStock(googList, "Google", quantity, price);
}
else
Controller.buyStock(amazList, "Amazon", quantity, price);
}
else {
System.out.print("Press 1 for LIFO accounting, 2 for FIFO accounting: ");
controlNum = input.nextInt();
if(controlNum == 1) {
if(stockSelect == 1)
Controller.sellLIFO(googList, quantity);
else
Controller.sellLIFO(amazList, quantity);
}
else {
if(stockSelect == 1)
Controller.sellFIFO(googList, quantity);
else
Controller.sellFIFO(amazList, quantity);
}
}
} while(true);
input.close();
}
public static void buyStock(LinkedList<Stock> list, String name, int quantity, double price) {
Stock temp = new Stock(name,quantity,price);
list.push(temp);
System.out.printf("You bought %d shares of %s stock at $%.2f per share %n", quantity, name, price);
}
public static void sellLIFO(LinkedList<Stock> list, int numToSell) {
// You need to write the code to sell the stock using the LIFO method (Stack)
// You also need to calculate the profit/loss on the sale
double total = 0; // this variable will store the total after the sale
double profit = 0; // the price paid minus the sale price, negative # means a loss
System.out.printf("You sold %d shares of %s stock at %.2f per share %n", numToSell, list.element().getName(), total/numToSell);
System.out.printf("You made $%.2f on the sale %n", profit);
}
public static void sellFIFO(LinkedList<Stock> list, int numToSell) {
// You need to write the code to sell the stock using the FIFO method (Queue)
// You also need to calculate the profit/loss on the sale
double total = 0; // this variable will store the total after the sale
double profit = 0; // the price paid minus the sale price, negative # means a loss
System.out.printf("You sold %d shares of %s stock at %.2f per share %n", numToSell, list.element().getName(), total/numToSell);
System.out.printf("You made $%.2f on the sale %n", profit);
}
}
//Driver
public class Driver {
public static void main(String[] args) {
System.out.println("Java Stock Exchange");
new Controller();
}
}
//Refer to images first for code and this will come after in order
//Stock
public Stock(int quantity, double price) {
this.name = "undefined";
this.quantity = quantity;
this.price = price;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getQuantity() {
return quantity;
}
public void setQuantity(int quantity) {
this.quantity = quantity;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
public String toString() {
return "Stock: " + this.getName() + " Quantity: " + this.getQuantity() + " Price: " + this.getPrice();
}
}
1. Add the missing functionality in “controller.java” to finish the
“sellLIFO” and “sellFIFO” methods.
“sellLIFO” and “sellFIFO” methods.
2. Provide error checking so users can’t sell a stock they don’t own or
try to sell more stock than they own. Eliminate that runtime exception.
3. Must change to where user has to input the stock they want to work with, not just Google or Amazon that can be stored in the LinkedList.
4. We like the layout and design of the application so don’t change any
of the other methods or objects. Only edit the Controller.java file!
5. Solution must be new (for postive feedback) and output should state the profit from selling number of stocks that user inputs!

Transcribed Image Text:29
30
31
32
33
34
35
36
37
38
39
` 48 4 2 3 4 5 46 47 48
40
41
42
43
44
49
50
51
52
public Stock(int quantity) {
this.name = "undefined";
this.quantity = quantity;
this.price = 0.0;
}
public Stock (double price) {
this.name = "undefined";
}
public Stock(String name, int quantity) {
this.name = name;
this.quantity = quantity;
this.price = 0.0;
}
this.quantity = 0;
this.price = price;
public Stock(String name, double price) {
}
this.name = name;
this.quantity = 0;
this.price = price;

Transcribed Image Text:5
600 ~ 3 4 5 6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
12345678
22
public class Stock {
private String name;
private int quantity;
private double price;
public Stock() {
}
public Stock (String name, int quantity, double price) {
this.name = name;
this.quantity = quantity;
this.price = price;
}
this.name = "undefined";
this.quantity = 0;
this.price = 0.0;
public Stock(String name) {
this.name = name;
this.quantity = 0;
this.price = 0.0;
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 6 images

Follow-up Questions
Read through expert solutions to related follow-up questions below.
Follow-up Question
Awesome! the program is running perfectly! Lastly, instead of using a Hashmap, can this be done just using a LinkedList where the output should stay the same, but not using a Hashmap?
Solution
Follow-up Question
The code is missing a part.
1. Refer to the image
Running the code given does not ask for "At what selling price per share". If I want to sell the stock, it should ask me at what price I want to sell the stock, but the code provided does not do that! Please fix
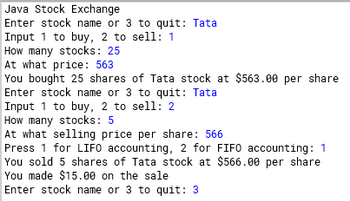
Transcribed Image Text:Java Stock Exchange
Enter stock name or 3 to quit: Tata
Input 1 to buy, 2 to sell: 1
How many stocks: 25
At what price: 563
You bought 25 shares of Tata stock at $563.00 per share
Enter stock name or 3 to quit: Tata
Input 1 to buy, 2 to sell: 2
How many stocks: 5
At what selling price per share: 566
Press 1 for LIFO accounting, 2 for FIFO accounting: 1
You sold 5 shares of Tata stock at $566.00 per share
You made $15.00 on the sale
Enter stock name or 3 to quit: 3
Solution
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
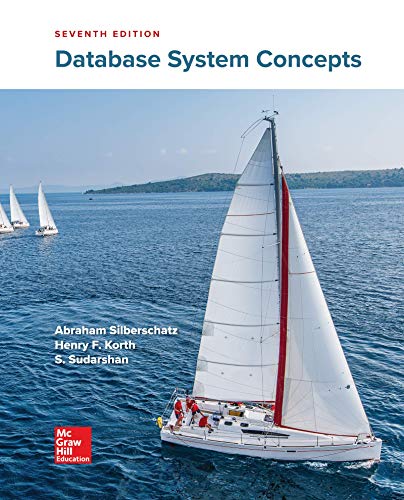
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
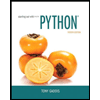
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
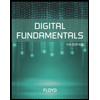
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
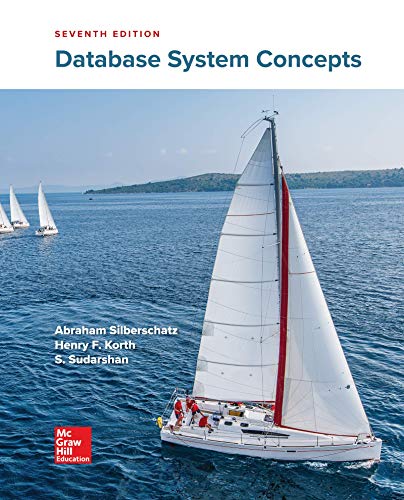
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
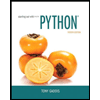
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
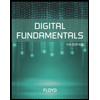
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
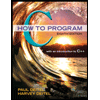
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
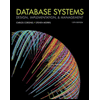
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
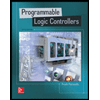
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education