Taking a Restaurant Order: Sentinel Style. Using the restaurant menu you selected in assigment below , you will create a program that will take an order from a customer and calculate the total. Your program shall, Display the menu with the prices. Take an order using the Sentinel approach in section 5.2.5 (atatched) The user will input numbers between 1 and 10, according to the menu you created below. Use zero as sentinel value (see Listing 5.5, p. 141) Inform the user about invalid input values (greater than 10 or less than 0) Add the prices of the dishes ordered After taking the order, the program displays the subtotal, the sales tax (8.75%), the grand total, and a suggested 15% tip. You can use the program you created below and modify to incorporate the new requirements. Notice that you will still need the multi-way conditional to add the right price value to the running total of the order. import sys #Enter number for each dish and price for users DishNumber= float(input("Enter numbers between 1 and 10:")) if DishNumber == 1: print("Cajun Calamari price is $9.00") elif DishNumber== 2: print("Shrimp Cocktail price is $9.00") elif DishNumber== 3: print:("Spicy Garlic Mussels price is $9.00") elif DishNumber== 4: print("Crispy Ahi price is $10.50") elif DishNumber== 5: print("Spinach Artichoke Dip price is $8.00") elif DishNumber== 6: print("Steamed Clams price is $10.00") elif DishNumber== 7: print("Chips and Dip price is $6.50") elif DishNumber== 8: print:("Beer Battered Ribs price is $6.00") elif DishNumber== 9: print("St.Louis Ribs price is $8.00") elif DishNumber== 10: print("Mozzarella Sticks price is $7.00") else: print("Error: Select number between 1 and 10")
Taking a Restaurant Order: Sentinel Style. Using the restaurant menu you selected in assigment below , you will create a program that will take an order from a customer and calculate the total.
Your program shall,
- Display the menu with the prices.
- Take an order using the Sentinel approach in section 5.2.5 (atatched) The user will input numbers between 1 and 10, according to the menu you created below.
- Use zero as sentinel value (see Listing 5.5, p. 141)
- Inform the user about invalid input values (greater than 10 or less than 0)
- Add the prices of the dishes ordered
- After taking the order, the program displays the subtotal, the sales tax (8.75%), the grand total, and a suggested 15% tip.
You can use the program you created below and modify to incorporate the new requirements. Notice that you will still need the multi-way conditional to add the right price value to the running total of the order.
import sys
#Enter number for each dish and price for users
DishNumber= float(input("Enter numbers between 1 and 10:"))
if DishNumber == 1:
print("Cajun Calamari price is $9.00")
elif DishNumber== 2:
print("Shrimp Cocktail price is $9.00")
elif DishNumber== 3:
print:("Spicy Garlic Mussels price is $9.00")
elif DishNumber== 4:
print("Crispy Ahi price is $10.50")
elif DishNumber== 5:
print("Spinach Artichoke Dip price is $8.00")
elif DishNumber== 6:
print("Steamed Clams price is $10.00")
elif DishNumber== 7:
print("Chips and Dip price is $6.50")
elif DishNumber== 8:
print:("Beer Battered Ribs price is $6.00")
elif DishNumber== 9:
print("St.Louis Ribs price is $8.00")
elif DishNumber== 10:
print("Mozzarella Sticks price is $7.00")
else:
print("Error: Select number between 1 and 10")


Trending now
This is a popular solution!
Step by step
Solved in 2 steps

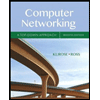
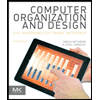
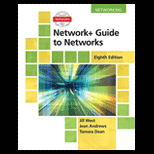
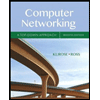
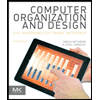
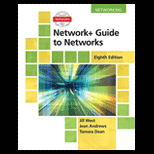
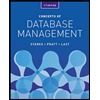
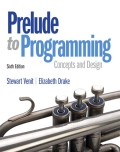
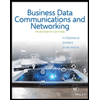