Step 1: Read your files! You should now have 3 files. Read each of these files, in the order listed below. staticarray.h-contains the class definition for StaticArray, which makes arrays behave a little more like Python lists In particular, pay attention to the private variables. Note that the array has been defined with a MAX capacity but that is not the same as having MAX elements. Which variable indicates the actual number of elements in the array? staticarray.cpp contains function definitions for StaticArray. Right now, that's just the print member function. Pay attention to the print() function. How many elements is it printing? main.cpp-client code to test your staticarray class. • Note that the multi-line comment format (/.../) has been used to comment out everything in main below the first print statement. As you proceed through the lab, you will need to move the starting comment (/") to a different location. Other than that, no changes Step 2: Default constructor The first step is to write the default constructor, which should initialize the array to empty by specifying that currently there are no elements in it. You can get away with just one line of code inside this function. On a piece of paper, write down the prototype for this function. Then highlight the black bar below to double-check, and put the prototype into the public section of the class definition in [staticarray.h In staticarray.cpp, write the function definition. Remember, just one line! Ask for help if that doesn't make sense. At this point, you should compile and run the code. The output statements in main will tell you what to expect. Step 3: Append The next step is to define an append() member function, which should do 2 things: 1. If there's room in the array (how can you determine this?), append the given value to the end of the array 2. Return true if the value was appended and false if the array was full. On a plece of paper, write down the prototype for this function. Then highlight the black bar below to double-check, and put the prototype into the public section of the class definition in staticarray.h In staticarray.cpp, write the function definition. Alas, this is more than just one line. In main.cpp, put the code that tests append (up to the declaration of indices) back into your code. Compile and check the output to make sure everything is correct so far. Step 4: At The at() member function is going to work just like the brackets notation for arrays. It gets passed an index, and should return the element at that index. In other words, arr.at(0) is like our version of arr[0]. If the index is outside the bounds of the array, it should return -11111 (an arbitrary default value). The ability to do this is a nice benefit vs. regular arrays. Write the prototype for the at function, and then check below:
Step 1: Read your files! You should now have 3 files. Read each of these files, in the order listed below. staticarray.h-contains the class definition for StaticArray, which makes arrays behave a little more like Python lists In particular, pay attention to the private variables. Note that the array has been defined with a MAX capacity but that is not the same as having MAX elements. Which variable indicates the actual number of elements in the array? staticarray.cpp contains function definitions for StaticArray. Right now, that's just the print member function. Pay attention to the print() function. How many elements is it printing? main.cpp-client code to test your staticarray class. • Note that the multi-line comment format (/.../) has been used to comment out everything in main below the first print statement. As you proceed through the lab, you will need to move the starting comment (/") to a different location. Other than that, no changes Step 2: Default constructor The first step is to write the default constructor, which should initialize the array to empty by specifying that currently there are no elements in it. You can get away with just one line of code inside this function. On a piece of paper, write down the prototype for this function. Then highlight the black bar below to double-check, and put the prototype into the public section of the class definition in [staticarray.h In staticarray.cpp, write the function definition. Remember, just one line! Ask for help if that doesn't make sense. At this point, you should compile and run the code. The output statements in main will tell you what to expect. Step 3: Append The next step is to define an append() member function, which should do 2 things: 1. If there's room in the array (how can you determine this?), append the given value to the end of the array 2. Return true if the value was appended and false if the array was full. On a plece of paper, write down the prototype for this function. Then highlight the black bar below to double-check, and put the prototype into the public section of the class definition in staticarray.h In staticarray.cpp, write the function definition. Alas, this is more than just one line. In main.cpp, put the code that tests append (up to the declaration of indices) back into your code. Compile and check the output to make sure everything is correct so far. Step 4: At The at() member function is going to work just like the brackets notation for arrays. It gets passed an index, and should return the element at that index. In other words, arr.at(0) is like our version of arr[0]. If the index is outside the bounds of the array, it should return -11111 (an arbitrary default value). The ability to do this is a nice benefit vs. regular arrays. Write the prototype for the at function, and then check below:
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
![Step 1: Read your files!
You should now have 3 files. Read each of these files, in the order listed below.
staticarray.h -- contains the class definition for StaticArray, which makes arrays behave a little more like Python lists
O In particular, pay attention to the private variables. Note that the array has been defined with a MAX capacity but that is not the same as having MAX elements. Which variable indicates the actual number of elements in the array?
staticarray.cpp -- contains function definitions for StaticArray. Right now, that's just the print() member function.
0 Pay attention to the print() function. How many elements is it printing?
main.cpp -- client code to test your staticarray class.
Note that the multi-line comment format (/* ... */) has been used to comment out everything in main below the first print statement. As you proceed through the lab, you will need to move the starting comment (/*) to a different location. Other than that, no changes ever need to be made to this file.
Step 2: Default constructor
●
O
The first step is to write the default constructor, which should initialize the array to empty by specifying that currently there are no elements in it. You can get away with just one line of code inside this function.
On a piece of paper, write down the prototype for this function. Then highlight the black bar below to double-check, and put the prototype into the public section of the class definition in staticarray.h.
In staticarray.cpp, write the function definition. Remember, just one line! Ask for help if that doesn't make sense.
At this point, you should compile and run the code. The output statements in main will tell you what to expect.
Step 3: Append
The next step is to define an append() member function, which should do 2 things:
1. If there's room in the array (how can you determine this?), append the given value to the end of the array
2. Return true if the value was appended and false if the array was full.
On a piece of paper, write down the prototype for this function. Then highlight the black bar below to double-check, and put the prototype into the public section of the class definition in staticarray.h.
In [staticarray.cpp, write the function definition. Alas, this is more than just one line.
In main.cpp, put the code that tests append (up to the declaration of indices) back into your code. Compile and check the output to make sure everything is correct so far.
Step 4: At
The at() member function is going to work just like the brackets notation for arrays. It gets passed an index, and should return the element at that index. In other words, arr.at(0) is like our version of arr[0].
If the index is outside the bounds of the array, it should return -11111 (an arbitrary default value). The ability to do this is a nice benefit vs. regular arrays.
Write the prototype for the at function, and then check below:
Again, add the prototype to the .h and the function definition to the .cpp. Then add everything up to "Testing sum" back into main.
Step 5: Sum
This function should just return the sum of all the current array elements. Double-check the prototype below -- if it surprises you, you want to ask for help at this point. Then add this member function to your class. In main, put everything up to "Testing remove" back in.
Step 6: Remove
You should pass this function the value you want to remove, and then delete the first array element equal to that value. In the example below, we have the values 200 through 209 in an array, and we pass 203 to remove:
(len is 10 before calling remove)
200 201 202 203 204 205 206 207 208 209
Here's what the array should look like after deletion. Note that, because the length is 9, we will consider the second 209 as arbitrary garbage; it's past the end of the array we're modeling.
200 201 202 204 205 206 207 208 209
prob 209 but it doesn't
matter
(len is now 9)
This function should return true if the delete was successful, and false if the value didn't match any array element. For example, for this array, remove (214) should return false.
Write and double-check the prototype:
Then add this function to your class, and run the original main, with all tests back in.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F1bfda259-7429-4717-b69c-7067c249bba0%2Fcca5a620-9570-44b2-bcdc-4fb149764e90%2Fmrrhwh9_processed.png&w=3840&q=75)
Transcribed Image Text:Step 1: Read your files!
You should now have 3 files. Read each of these files, in the order listed below.
staticarray.h -- contains the class definition for StaticArray, which makes arrays behave a little more like Python lists
O In particular, pay attention to the private variables. Note that the array has been defined with a MAX capacity but that is not the same as having MAX elements. Which variable indicates the actual number of elements in the array?
staticarray.cpp -- contains function definitions for StaticArray. Right now, that's just the print() member function.
0 Pay attention to the print() function. How many elements is it printing?
main.cpp -- client code to test your staticarray class.
Note that the multi-line comment format (/* ... */) has been used to comment out everything in main below the first print statement. As you proceed through the lab, you will need to move the starting comment (/*) to a different location. Other than that, no changes ever need to be made to this file.
Step 2: Default constructor
●
O
The first step is to write the default constructor, which should initialize the array to empty by specifying that currently there are no elements in it. You can get away with just one line of code inside this function.
On a piece of paper, write down the prototype for this function. Then highlight the black bar below to double-check, and put the prototype into the public section of the class definition in staticarray.h.
In staticarray.cpp, write the function definition. Remember, just one line! Ask for help if that doesn't make sense.
At this point, you should compile and run the code. The output statements in main will tell you what to expect.
Step 3: Append
The next step is to define an append() member function, which should do 2 things:
1. If there's room in the array (how can you determine this?), append the given value to the end of the array
2. Return true if the value was appended and false if the array was full.
On a piece of paper, write down the prototype for this function. Then highlight the black bar below to double-check, and put the prototype into the public section of the class definition in staticarray.h.
In [staticarray.cpp, write the function definition. Alas, this is more than just one line.
In main.cpp, put the code that tests append (up to the declaration of indices) back into your code. Compile and check the output to make sure everything is correct so far.
Step 4: At
The at() member function is going to work just like the brackets notation for arrays. It gets passed an index, and should return the element at that index. In other words, arr.at(0) is like our version of arr[0].
If the index is outside the bounds of the array, it should return -11111 (an arbitrary default value). The ability to do this is a nice benefit vs. regular arrays.
Write the prototype for the at function, and then check below:
Again, add the prototype to the .h and the function definition to the .cpp. Then add everything up to "Testing sum" back into main.
Step 5: Sum
This function should just return the sum of all the current array elements. Double-check the prototype below -- if it surprises you, you want to ask for help at this point. Then add this member function to your class. In main, put everything up to "Testing remove" back in.
Step 6: Remove
You should pass this function the value you want to remove, and then delete the first array element equal to that value. In the example below, we have the values 200 through 209 in an array, and we pass 203 to remove:
(len is 10 before calling remove)
200 201 202 203 204 205 206 207 208 209
Here's what the array should look like after deletion. Note that, because the length is 9, we will consider the second 209 as arbitrary garbage; it's past the end of the array we're modeling.
200 201 202 204 205 206 207 208 209
prob 209 but it doesn't
matter
(len is now 9)
This function should return true if the delete was successful, and false if the value didn't match any array element. For example, for this array, remove (214) should return false.
Write and double-check the prototype:
Then add this function to your class, and run the original main, with all tests back in.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
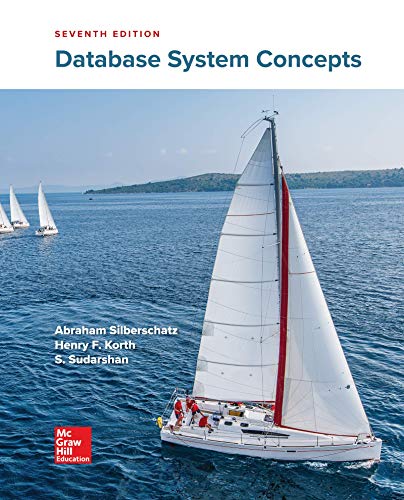
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
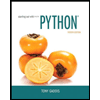
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
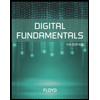
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
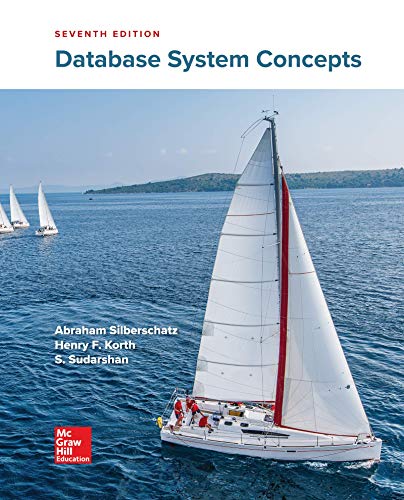
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
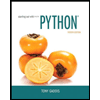
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
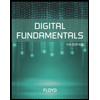
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
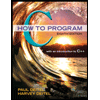
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
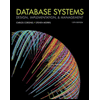
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
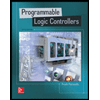
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education