sells different electronics products such as Computers, Laptops and accessories. The store Management requires an automated software to keep track of items in stock and prices. You as a software designer and developer are asked to develop a system for the store. The first task you should carry out is to design and code a class related to stocked Items. You can name this class as Item_in_Stock. The class should consist of the following: • A data member Item_code to store an item code e.g, C15, a data member to store item name, a data member to store item description, a data member to store quantity of items in stock and a data member item_price to store price of an item. You must use the appropriate data types and access specifier for each data member. • Class should have a constructor that initialises class objects with specified item_code, quantity and price of items. • Getter and setters methods for all the data members including getItemCat() method which returns “Unknown Item Category”, getItemName() method which returns the "Unknown Item Name" and getItemDescription() which returns "Unknown Item Description”. These methods will be overridden in later tasks of the assignment to set and display information related to specific items. • An add_Item method to add items in stock with a check that item stock does not exceed 25. An appropriate message should be displayed if inserted item exceeds the limit. • An item_Sell member method to sell items, method should ask for quantity of selling item and then reduces the stock level accordingly. • A tax_on_Item method gives tax on items (Tax is fixed at 5%) • A method to set item price without tax and get method to get item price both with and without tax • A method named get_Item_Details() that provides Item code, Item Category, Item name, Item description, quantity in stock, and price with and without tax. Task 1.1 Graphically represent above mentioned class in UML by drawing class diagram. Task 1.2. Implementation of the class using Java language. Write a program called Stocked_Item with main () method to test the Item_in_Stock class. Testing is an integral part of development. Write suitable test cases in the given format below for your class. Test Case Purpose Expected result Example OUTPUT: Creating a stock with 5 units Laptops i-5 11g 8gb 1tb, price R.O 180 per item, and item code C15 Laptops item stock information: Item Category: Unknown Item Category Item Name: Unknown Item Name Description: Unknown Item Description StockCode: C15 Price Without Tax: R.O 180 Price With Tax: R.O 189 Total quantity in store: 5 Adding 3 more items Printing item stock information: Item Category: Unknown Item Category Item Name: Unknown Item Name Description: Unknown Item Description StockCode: C15 Price Without Tax: R. O 180 Price With Tax: R.O 189 Total quantity in store: 8 Sold 2 items Printing item stock information: Item Category: Unknown Item Category Item Name: Unknown Item Name Description: Unknown Item Description StockCode: C15 Price Without Tax: R. O 180 Price With Tax: R.O 189 Total quantity in store: 6 Changing price to R.O 200 Printing item stock information: Item Category: Unknown Item Category Item Name: Unknown Item Name Description: Unknown Item Description StockCode: C15 Price Without Tax: R. O 200 Price With Tax: R. O 210 Total quantity in store: 6 Adding 25 more Items The error: Item stock must not exceed 25 items
sells different electronics products such as Computers, Laptops and accessories. The store Management requires an automated software to keep track of items in stock and prices. You as a software designer and developer are asked to develop a system for the store. The first task you should carry out is to design and code a class related to stocked Items. You can name this class as Item_in_Stock. The class should consist of the following: • A data member Item_code to store an item code e.g, C15, a data member to store item name, a data member to store item description, a data member to store quantity of items in stock and a data member item_price to store price of an item. You must use the appropriate data types and access specifier for each data member. • Class should have a constructor that initialises class objects with specified item_code, quantity and price of items. • Getter and setters methods for all the data members including getItemCat() method which returns “Unknown Item Category”, getItemName() method which returns the "Unknown Item Name" and getItemDescription() which returns "Unknown Item Description”. These methods will be overridden in later tasks of the assignment to set and display information related to specific items. • An add_Item method to add items in stock with a check that item stock does not exceed 25. An appropriate message should be displayed if inserted item exceeds the limit. • An item_Sell member method to sell items, method should ask for quantity of selling item and then reduces the stock level accordingly. • A tax_on_Item method gives tax on items (Tax is fixed at 5%) • A method to set item price without tax and get method to get item price both with and without tax • A method named get_Item_Details() that provides Item code, Item Category, Item name, Item description, quantity in stock, and price with and without tax. Task 1.1 Graphically represent above mentioned class in UML by drawing class diagram. Task 1.2. Implementation of the class using Java language. Write a program called Stocked_Item with main () method to test the Item_in_Stock class. Testing is an integral part of development. Write suitable test cases in the given format below for your class. Test Case Purpose Expected result Example OUTPUT: Creating a stock with 5 units Laptops i-5 11g 8gb 1tb, price R.O 180 per item, and item code C15 Laptops item stock information: Item Category: Unknown Item Category Item Name: Unknown Item Name Description: Unknown Item Description StockCode: C15 Price Without Tax: R.O 180 Price With Tax: R.O 189 Total quantity in store: 5 Adding 3 more items Printing item stock information: Item Category: Unknown Item Category Item Name: Unknown Item Name Description: Unknown Item Description StockCode: C15 Price Without Tax: R. O 180 Price With Tax: R.O 189 Total quantity in store: 8 Sold 2 items Printing item stock information: Item Category: Unknown Item Category Item Name: Unknown Item Name Description: Unknown Item Description StockCode: C15 Price Without Tax: R. O 180 Price With Tax: R.O 189 Total quantity in store: 6 Changing price to R.O 200 Printing item stock information: Item Category: Unknown Item Category Item Name: Unknown Item Name Description: Unknown Item Description StockCode: C15 Price Without Tax: R. O 200 Price With Tax: R. O 210 Total quantity in store: 6 Adding 25 more Items The error: Item stock must not exceed 25 items
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
100%
Part 1
E-TechComputer store in Oman sells different electronics products such as Computers, Laptops and accessories.
The store Management requires an automated software to keep track of items in stock and prices. You as a software designer and developer are asked to develop a system for the store.
The first task you should carry out is to design and code a class related to stocked Items. You can name this class as Item_in_Stock.
The class should consist of the following:
• A data member Item_code to store an item code e.g, C15, a data member to store item name, a data member to store item description, a data member to store quantity of items in stock and a data member item_price to store price of an item. You must use the appropriate data types and access specifier for each data member.
• Class should have a constructor that initialises class objects with specified item_code, quantity and price of items.
• Getter and setters methods for all the data members including getItemCat() method which returns “Unknown Item Category”, getItemName() method which returns the "Unknown Item Name" and getItemDescription() which returns "Unknown Item Description”. These methods will be overridden in later tasks of the assignment to set and display information related to specific items.
• An add_Item method to add items in stock with a check that item stock does not exceed 25. An appropriate message should be displayed if inserted item exceeds the limit.
• An item_Sell member method to sell items, method should ask for quantity of selling item and then reduces the stock level accordingly.
• A tax_on_Item method gives tax on items (Tax is fixed at 5%)
• A method to set item price without tax and get method to get item price both with and without tax
• A method named get_Item_Details() that provides Item code, Item Category, Item name, Item description, quantity in stock, and price with and without tax.
Task 1.1 Graphically represent above mentioned class in UML by drawing class diagram.
Task 1.2. Implementation of the class using Java language.
Write a program called Stocked_Item with main () method to test the
Item_in_Stock class.
Testing is an integral part of development. Write suitable test cases in the given format below for your class.
Test Case Purpose Expected result
Example OUTPUT:
Creating a stock with 5 units Laptops i-5 11g 8gb 1tb, price R.O 180 per item, and item code C15 Laptops item stock information:
Item Category: Unknown Item Category
Item Name: Unknown Item Name
Description: Unknown Item Description StockCode: C15
Price Without Tax: R.O 180
Price With Tax: R.O 189
Total quantity in store: 5
Adding 3 more items
Printing item stock information:
Item Category: Unknown Item Category Item Name: Unknown Item Name Description: Unknown Item Description StockCode: C15
Price Without Tax: R. O 180
Price With Tax: R.O 189
Total quantity in store: 8
Sold 2 items
Printing item stock information:
Item Category: Unknown Item Category Item Name: Unknown Item Name Description: Unknown Item Description StockCode: C15
Price Without Tax: R. O 180
Price With Tax: R.O 189
Total quantity in store: 6
Changing price to R.O 200
Printing item stock information:
Item Category: Unknown Item Category Item Name: Unknown Item Name Description: Unknown Item Description StockCode: C15
Price Without Tax: R. O 200
Price With Tax: R. O 210
Total quantity in store: 6
Adding 25 more Items
The error: Item stock must not exceed 25 items
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
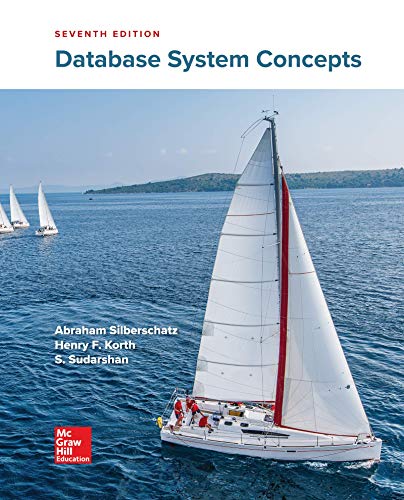
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
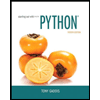
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
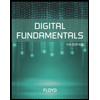
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
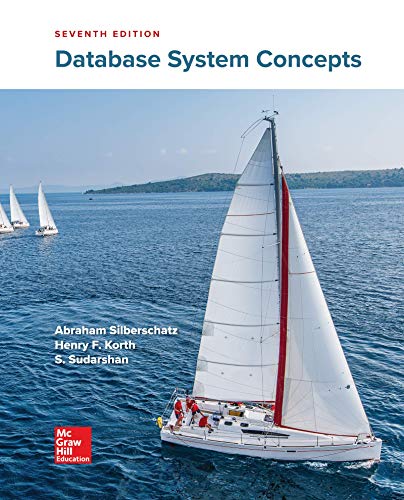
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
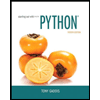
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
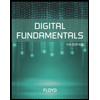
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
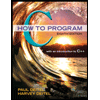
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
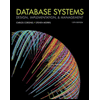
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
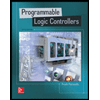
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education