Q1: How many total calls to malloc are made during createEmployee (including readEmployee and anything it calls)? Q2: If the employee's name is Joe Smith, how much total memory is allocated for the structure and the name (not just for the name)?
//below is the code I need help with answering the questions in the image//
#include <stdio.h>
#include <stdlib.h>
struct employees
{
char name[20];
int ssn[9];
int yearBorn, salary;
};
// function to read the employee data from the user
void readEmployee(struct employees *emp)
{
printf("Enter name: ");
gets(emp->name);
printf("Enter ssn: ");
for (int i = 0; i < 9; i++)
scanf("%d", &emp->ssn[i]);
printf("Enter birth year: ");
scanf("%d", &emp->yearBorn);
printf("Enter salary: ");
scanf("%d", &emp->salary);
}
// function to create a pointer of employee type
struct employees *createEmployee()
{
// creating the pointer
struct employees *emp = malloc(sizeof(struct employees));
// function to read the data
readEmployee(emp);
// returning the data
return emp;
}
// function to print the employee data to console
void display(struct employees *e)
{
printf("%s", e->name);
printf(" %d%d%d-%d%d-%d%d%d%d", e->ssn[0], e->ssn[1], e->ssn[2], e->ssn[3], e->ssn[4], e->ssn[5], e->ssn[6], e->ssn[7], e->ssn[8]);
printf(" %d", e->yearBorn);
printf("\n$%d.", e->salary);
}
// function to free the memory of the employee pointer
void releaseEmployee(struct employees *e)
{
free(e);
}
// main method
int main()
{
// creating the employee
struct employees *emp = createEmployee();
// printing the information
display(emp);
// free the memory
releaseEmployee(emp);
return 0;
}
![Q1: How many total calls to malloc are made during createEmployee (including
readEmployee and anything it calls)?
Q2: If the employee's name is Joe Smith, how much total memory is allocated
for the structure and the name (not just for the name)?
Q3: Many people put the SSN in a char[9] field (I kind of encouraged it). Is that
big enough to hold the ssn string? Why didn't it create a problem?
Q4: How many calls to free do you need to make? Does it agree with the number
of calls to malloc that were made?
Q5: What would happen if you passed in the address of the initialized global
variable into releaseEmployee? Would anything different happen if you passed in
the address of a local variable (on the stack)?](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F2c4d07ff-5ab1-477f-b7b6-63a497af629e%2F88ad5e18-7d82-4e40-82ca-17c1c8bf2e35%2Ftnjyhxg_processed.png&w=3840&q=75)

Step by step
Solved in 2 steps

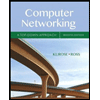
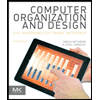
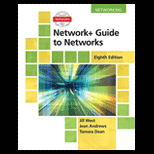
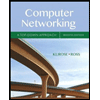
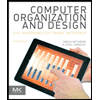
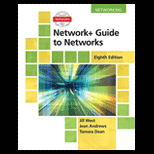
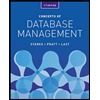
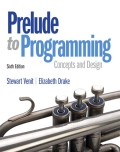
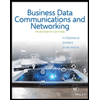