Python code keeps failing. Can someone help me locate the issue
Python code keeps failing. Can someone help me locate the issue? Thank you.
class Mancala:
def __init__(self):
"""Initialize the game."""
# create the board
self.board = [0] * 14
# initialize the seed count
self.seed_count = 48
# player 1 starts the game
self.current_player = 1
def create_players(self):
"""Create the two players."""
# player 1 is the human player
self.player1 = Player("Human", 1)
# player 2 is the computer player
self.player2 = Player("Computer", 2)
def print_board(self):
"""Print the current state of the board."""
print("+-----+-----+-----+-----+-----+-----+")
print("| {} | {} | {} | {} | {} | {} |".format(*self.board[6:12]))
print("+-----+-----+-----+-----+-----+-----+")
print("| {} | {} | {} | {} | {} | {} |".format(*self.board[6:12]))
print("+-----+-----+-----+-----+-----+-----+")
def return_winner(self):
"""Return the winner of the game."""
# player 1 wins if the game is over and player 2 has no more seeds
if self.is_game_over() and self.board[6] == 0:
return self.player1
# player 2 wins if the game is over and player 1 has no more seeds
if self.is_game_over() and self.board[13] == 0:
return self.player2
# otherwise, the game is not over yet
return None
def play_game(self):
"""Play the game."""
# create the players
self.create_players()
# print the initial state of the board
self.print_board()
# while the game is not over, keep playing
while not self.is_game_over():
# get the next move from the current player
move = self.current_player.get_move(self)
# make the move
self.make_move(move)
# print the current state of the board
self.print_board()
# check if the game is over
if self.is_game_over():
# if so, return the winner
return self.return_winner()
# switch to the other player
self.current_player = self.player1 if self.current_player == self.player2 else self.player2
def make_move(self, move):
"""Make a move in the game."""
# get the start position and end position for the move
start = move[0]
end = move[1]
# get the number of seeds to move
seeds_to_move = self.board[start]
# clear the start position
self.board[start] = 0
# move the seeds
for i in range(start+1, start+seeds_to_move+1):
self.board[i % len(self.board)] += 1
# check if the last seed was moved into the player's mancala
if self.current_player.mancala_position == end:
# if so, the player gets another turn
return
# check if the last seed was moved into an empty pit on the player's side
if self.board[end] == 1 and end in self.current_player.pit_positions:
# if so, the player captures the seeds in the opposite pit
captured_seeds = self.board[self.get_opposite_pit(end)]
self.board[self.get_opposite_pit(end)] = 0
self.board[end] = 0
self.board[self.current_player.mancala_position] += captured_seeds
return
if self.board[end] == 1 and end not in self.current_player.pit_positions:
return
self.current_player = self.player1 if self.current_player == self.player2 else self.player2
def get_opposite_pit(self, pit_position):
return len(self.board) - pit_position - 1
def is_game_over(self):
return self.board[6] == 0 or self.board[13] == 0
class Player:
def __init__(self, name, player_id):
self.name = name
self.id = player_id
self.mancala_position = 6 if self.id == 1 else 13
self.pit_positions = list(range(0, 6)) if self.id == 1 else list(range(7, 13))
def get_move(self, mancala):
possible_moves = mancala.get_possible_moves()
if len(possible_moves) == 0:
return None
print("{}, please enter a move: ".format(self.name))
print("Possible moves: {}".format(possible_moves))
move = input()
return move
def main()
game = Mancala(
winner = game.play_game(
print("{} wins!".format(winner.name))
if __name__ == "__main__":
main()

Trending now
This is a popular solution!
Step by step
Solved in 8 steps with 9 images

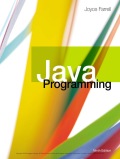
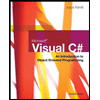
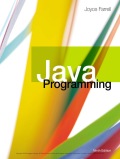
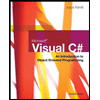